C#에서 ListBox 선택 항목의 값 가져오기
-
ListBox.SelectedValue
속성을 사용하여C#
에서 ListBox 선택 항목의 값 가져오기 -
ListBox.SelectedItem
속성을 사용하여C#
에서 ListBox 선택 항목의 값 가져오기 -
GetItemText()
메서드를 사용하여C#
에서 ListBox 선택 항목의 값 가져오기
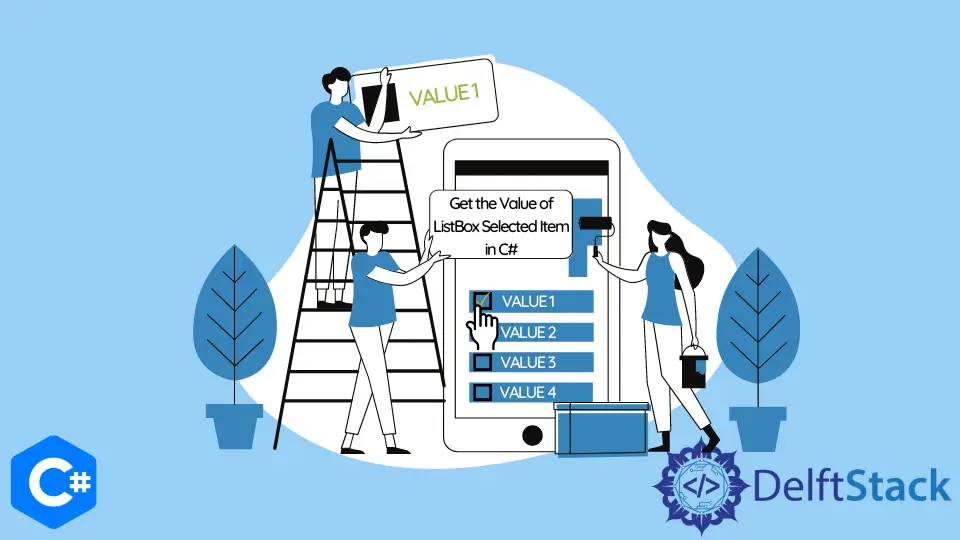
이 문서에서는 프로그래밍 방식으로 ListBox 컨트롤의 선택한 항목 값을 가져오는 방법을 설명합니다. C#에서 ListBox 컨트롤 SelectionMode
속성에는 Single
및 Multiple
의 두 가지 가능한 값이 있습니다.
Single
모드를 사용하면 ListBox 컨트롤에서 단일 항목을 선택할 수 있습니다. 일반적으로 C#에서 ListBox 선택 항목의 값을 가져오는 방법에는 여러 가지가 있습니다.
ListBox.SelectedValue
속성을 사용하여 C#
에서 ListBox 선택 항목의 값 가져오기
SelectedValue
속성은 바인딩의 대체 수단입니다. ListBox에서 선택한 개체 또는 항목의 다른 값을 얻을 수 있습니다.
SelectedValuePath
속성은 ListBox의 값을 선택하는 데 중요한 역할을 합니다. 예를 들어, ListBox가 카테고리 개체 컬렉션에 바인딩되어 있고 각각의 개체가 ID
, Name
, 및 Context
속성을 가지고 있는 경우, SelectedValuePath = Name
으로 설정하면 선택한 카테고리의 Name
속성 값이 SelectedValue
에 반환됩니다.
// create a `button1` button and a `listBox1` listbox in your C# project's `Form1`
using System;
using System.Windows.Forms;
namespace ListboxSelectedValue {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
// add items and values to the `listBox1` listbox
listBox1.Items.Add(new ListItem { Name = "Item1", Value = 1 });
listBox1.Items.Add(new ListItem { Name = "Item2", Value = 2 });
listBox1.Items.Add(new ListItem { Name = "Item3", Value = 3 });
listBox1.Items.Add(new ListItem { Name = "Item4", Value = 4 });
}
// create a class to add values and text to the `listBox1` listbox
public class ListItem {
public string Name { get; set; }
public int Value { get; set; }
public override string ToString() {
return Name;
}
}
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
var value = ((ListItem)listBox1.SelectedItem).Value;
// to display the selected value
MessageBox.Show("The value of your selected item: " + value);
}
}
}
출력:
*select an item from the listbox item2*
*press button1*
The value of your selected item: 2
ListBox.SelectedItem
속성을 사용하여 C#
에서 ListBox 선택 항목의 값 가져오기
ListBox.SelectedItem
속성은 ListBox 선택 항목의 값을 가져오거나 설정할 수 있습니다. ListBox에서 현재 선택된 항목의 인덱스 위치와 값을 얻으려면 SelectedIndex
속성을 사용하십시오.
C#에서 SelectedItem
속성은 ListBox가 바인딩된 전체 개체 또는 포함된 항목을 반환합니다. 이를 통해 전체 개체를 해당 개체의 단일 속성 값(예: Category
개체의 ID
속성)이 아니라 ListBox가 바인딩된 속성에 바인딩할 수 있습니다.
// create a `button1` button and a `listBox1` listbox with items
// use the following code in your C# form's `button1_Click` event
private void button1_Click(object sender, EventArgs e) {
// to retrive the object from listbox and store it into a string variable
string curItem = listBox1.SelectedItem.ToString();
// to show the value of selected item stored as a string
MessageBox.Show("Your selected item: " + curItem);
}
출력:
*select item3 from the listbox and press button1*
Your selected item: item3
GetItemText()
메서드를 사용하여 C#
에서 ListBox 선택 항목의 값 가져오기
C#의 GetItemText
메서드는 ListBox에서 선택한 항목의 텍스트 표현을 반환합니다. GetItemText(Object)
에 의해 반환된 값은 ToString
항목의 메서드 값입니다.
C# 프로젝트에서 listBox1
ListBox 및 button1
버튼이 포함된 Form1
을 만듭니다. 그런 다음 button1_Click
이벤트에 다음 코드를 붙여넣어 ListBox에서 선택한 항목의 값을 검색합니다.
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
string text = listBox1.GetItemText(listBox1.SelectedItem);
// to display the selected value
MessageBox.Show("The value of your selected item: " + text);
}
출력:
*select item4 from the listbox and press button1*
The value of your selected item: item4
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub