C# で ListBox の選択項目の値を取得する
-
ListBox.SelectedValue
プロパティを使用して、C#
で ListBox の選択項目の値を取得する -
ListBox.SelectedItem
プロパティを使用して、C#
で ListBox の選択項目の値を取得する -
GetItemText()
メソッドを使用して、C#
で ListBox 選択項目の値を取得する
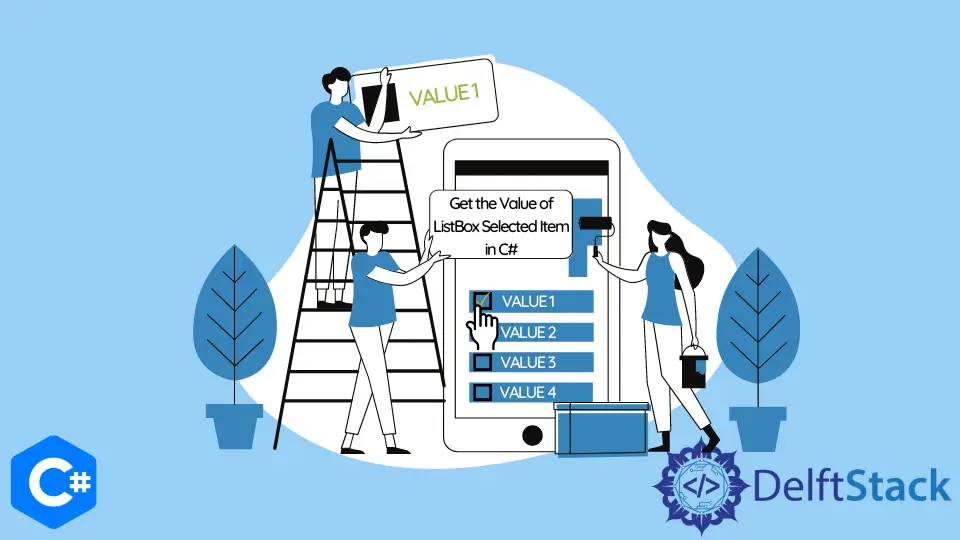
この記事では、ListBox コントロールの選択された項目の値をプログラムで取得する方法について説明します。 C# では、ListBox コントロールの SelectionMode
プロパティには、Single
と Multiple
の 2つの値があります。
Single
モードでは、ListBox コントロールから 1つの項目を選択できます。 一般に、C# で ListBox の選択された項目の値を取得する方法は複数あります。
ListBox.SelectedValue
プロパティを使用して、C#
で ListBox の選択項目の値を取得する
SelectedValue
プロパティは、バインドの代替手段です。 ListBox で選択したオブジェクトまたは項目のさまざまな値を取得できます。
SelectedValuePath
プロパティは、ListBox の値を選択する際に重要な役割を果たします。 たとえば、ListBox が、それぞれが ID
、Name
、および Context
プロパティを持つカテゴリ オブジェクトのコレクションにバインドされている場合、SelectedValuePath = Name
により、選択されたカテゴリの Name の値が生成されます。
SelectedValue
で返されるプロパティ。
// create a `button1` button and a `listBox1` listbox in your C# project's `Form1`
using System;
using System.Windows.Forms;
namespace ListboxSelectedValue {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
// add items and values to the `listBox1` listbox
listBox1.Items.Add(new ListItem { Name = "Item1", Value = 1 });
listBox1.Items.Add(new ListItem { Name = "Item2", Value = 2 });
listBox1.Items.Add(new ListItem { Name = "Item3", Value = 3 });
listBox1.Items.Add(new ListItem { Name = "Item4", Value = 4 });
}
// create a class to add values and text to the `listBox1` listbox
public class ListItem {
public string Name { get; set; }
public int Value { get; set; }
public override string ToString() {
return Name;
}
}
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
var value = ((ListItem)listBox1.SelectedItem).Value;
// to display the selected value
MessageBox.Show("The value of your selected item: " + value);
}
}
}
出力:
*select an item from the listbox item2*
*press button1*
The value of your selected item: 2
ListBox.SelectedItem
プロパティを使用して、C#
で ListBox の選択項目の値を取得する
ListBox.SelectedItem
プロパティは、ListBox で選択された項目の値を取得または設定できます。 ListBox で現在選択されている項目のインデックス位置と値を取得する場合は、SelectedIndex
プロパティを使用します。
C# では、SelectedItem
プロパティは、ListBox がバインドされているオブジェクト全体またはそれに含まれる項目を返します。 ListBox がバインドされているプロパティにオブジェクト全体をバインドすることのみを可能にし、そのオブジェクトの単一のプロパティの値 (Category
オブジェクトの ID
プロパティなど) をバインドすることはできません。
// create a `button1` button and a `listBox1` listbox with items
// use the following code in your C# form's `button1_Click` event
private void button1_Click(object sender, EventArgs e) {
// to retrive the object from listbox and store it into a string variable
string curItem = listBox1.SelectedItem.ToString();
// to show the value of selected item stored as a string
MessageBox.Show("Your selected item: " + curItem);
}
出力:
*select item3 from the listbox and press button1*
Your selected item: item3
GetItemText()
メソッドを使用して、C#
で ListBox 選択項目の値を取得する
C# の GetItemText
メソッドは、ListBox で選択された項目のテキスト表現を返します。 GetItemText(Object)
によって返される値は、ToString
アイテムのメソッドの値です。
listBox1
ListBox と button1
ボタンを含む Form1
を C# プロジェクトに作成します。 その後、button1_Click
イベントに次のコードを貼り付けて、ListBox で選択した項目の値を取得します。
private void button1_Click(object sender, EventArgs e) {
// to get the value when a listBox1 item is selected
string text = listBox1.GetItemText(listBox1.SelectedItem);
// to display the selected value
MessageBox.Show("The value of your selected item: " + text);
}
出力:
*select item4 from the listbox and press button1*
The value of your selected item: item4
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub