How to Set null in DateTime in C#
-
Understanding the Basics of
DateTime
inC#
-
Assigning a Max and Min Values to a
DateTime
inC#
-
Assigning
null
Value toDateTime
inC#
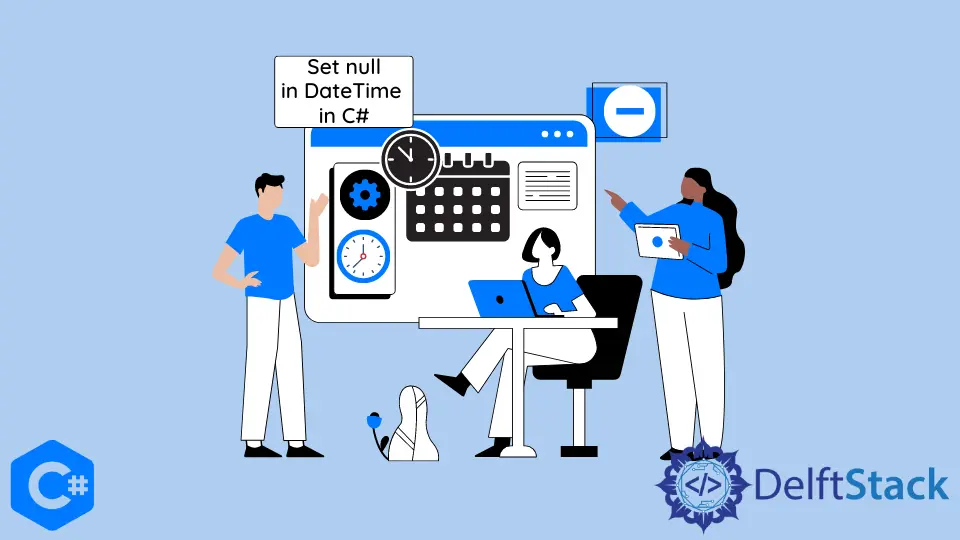
We’ll see how to set a null
value for DateTime
in this lesson. To understand this concept fully, we need to be familiar with the fundamentals of DateTime
and nullable values.
Understanding the Basics of DateTime
in C#
Let’s say a user wants to start the clock from the start of the day on 25th December 2015. We will assign values to the DateTime
object.
Code Snippet:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
DateTime date1 = new DateTime(2015, 12, 25); // Assgining User Defined Time ;
Console.WriteLine("Time " + date1);
Console.ReadKey();
}
}
}
Output:
Time 12/25/2015 12:00:00 AM
Assigning a Max and Min Values to a DateTime
in C#
Assigning a min value to a DateTime
would start the time back from the start Min Time: 1/1/0001 12:00:00 AM
. The same goes for the Max time, and it would start the time with the maximum value of time Max Time: 12/31/9999 11:59:59 PM
.
Code Snippet:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// How to Define an uninitialized date. ?
// First Method to use MinValue field of datetime object
DateTime date2 = DateTime.MinValue; // minimum date value
Console.WriteLine("Time: " + date2);
// OR
date2 = DateTime.MaxValue;
Console.WriteLine("Max Time: " + date2);
Console.ReadKey();
}
}
}
Output:
Min Time: 1/1/0001 12:00:00 AM
Max Time: 12/31/9999 11:59:59 PM
Assigning null
Value to DateTime
in C#
The DateTime
is not nullable because, by default, it is a value type. The value type is a form of data stored in its memory allocation.
On the other hand, if we were to use the Nullable DateTime
. We can assign a null
value to it.
Code Snippet:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// By default DateTime is not nullable because it is a Value Type;
// Problem # Assgin Null value to datetime instead of MAX OR MIN Value.
// Using Nullable Type
Nullable<DateTime> nulldatetime; // variable declaration
nulldatetime = DateTime.Now; // Assgining DateTime to Nullable Object.
Console.WriteLine("Current Date Is " + nulldatetime); // printing Date..
nulldatetime = null; // assgining null to datetime object.
Console.WriteLine("My Value is null ::" + nulldatetime);
Console.ReadKey();
}
}
}
Output:
Current Date Is 02/11/2022 18:57:33
My Value is null ::
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn