C#의 DateTime에서 null 설정
Haider Ali
2023년10월12일
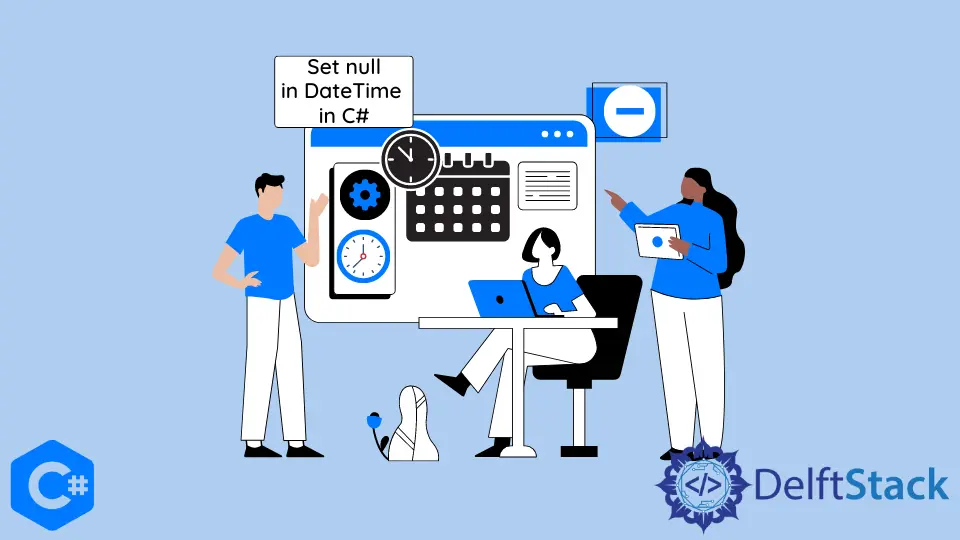
이 레슨에서 DateTime
에 null
값을 설정하는 방법을 살펴보겠습니다. 이 개념을 완전히 이해하려면 DateTime
과 nullable 값의 기본 사항에 익숙해져야 합니다.
C#
에서 DateTime
의 기본 이해
사용자가 2015년 12월 25일 하루의 시작부터 시계를 시작하려고 한다고 가정해 보겠습니다. DateTime
개체에 값을 할당합니다.
코드 조각:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
DateTime date1 = new DateTime(2015, 12, 25); // Assgining User Defined Time ;
Console.WriteLine("Time " + date1);
Console.ReadKey();
}
}
}
출력:
Time 12/25/2015 12:00:00 AM
C#
에서 DateTime
에 최대 및 최소 값 할당
DateTime
에 최소값을 할당하면 Min Time: 1/1/0001 12:00:00 AM
시작부터 시간이 다시 시작됩니다. Max 시간도 마찬가지이며 Max Time: 12/31/9999 11:59:59 PM
시간의 최대값으로 시간을 시작합니다.
코드 조각:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// How to Define an uninitialized date. ?
// First Method to use MinValue field of datetime object
DateTime date2 = DateTime.MinValue; // minimum date value
Console.WriteLine("Time: " + date2);
// OR
date2 = DateTime.MaxValue;
Console.WriteLine("Max Time: " + date2);
Console.ReadKey();
}
}
}
출력:
Min Time: 1/1/0001 12:00:00 AM
Max Time: 12/31/9999 11:59:59 PM
C#
의 DateTime
에 null
값 할당
DateTime
은 기본적으로 값 유형
이기 때문에 null을 허용하지 않습니다. 값 유형
은 메모리 할당에 저장된 데이터 형식입니다.
반면에 Nullable DateTime
을 사용하는 경우입니다. 우리는 그것에 null
값을 할당할 수 있습니다.
코드 조각:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// By default DateTime is not nullable because it is a Value Type;
// Problem # Assgin Null value to datetime instead of MAX OR MIN Value.
// Using Nullable Type
Nullable<DateTime> nulldatetime; // variable declaration
nulldatetime = DateTime.Now; // Assgining DateTime to Nullable Object.
Console.WriteLine("Current Date Is " + nulldatetime); // printing Date..
nulldatetime = null; // assgining null to datetime object.
Console.WriteLine("My Value is null ::" + nulldatetime);
Console.ReadKey();
}
}
}
출력:
Current Date Is 02/11/2022 18:57:33
My Value is null ::
작가: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn