How to Get Current Year in C#
-
Use the
DateTime.Now.Year
Property to Get the Current Year in C# -
Use the
DateTime.UtcNow.Year
Property to Get the Current Year in C# -
Use the
DatimeTime.Today.Year
Property to Get the Current Year in C# - Conclusion
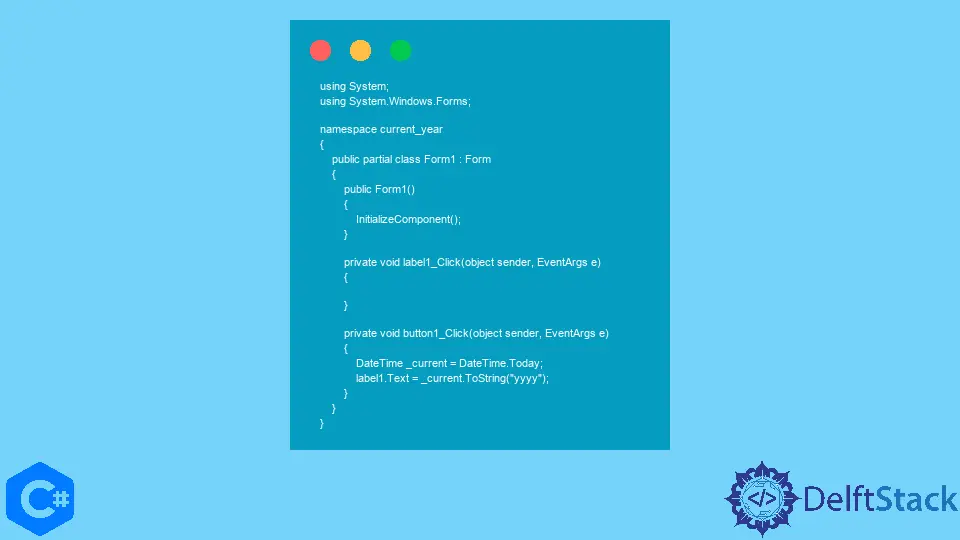
In the realm of C# programming, gaining insights into the current year is a fundamental task often encountered in various applications such as logging, timestamping, and date-based calculations. This article delves into the intricacies of extracting the current year using different properties within the DateTime
structure.
Use the DateTime.Now.Year
Property to Get the Current Year in C#
In C#, the DateTime.Now
property returns a DateTime
object representing the current date and time in the local time zone of the computer. The DateTime
structure in C# has a property called Year
that allows you to extract the year component from a DateTime
object.
Here’s a breakdown of the expression DateTime.Now.Year
:
-
DateTime.Now
:DateTime
is a structure in C# that represents dates and times. TheNow
property of theDateTime
structure returns the current date and time. -
.Year
: Once you have aDateTime
object (representing the current date and time), you can access various properties to get specific components of the date and time. TheYear
property specifically retrieves the year component from theDateTime
object.
Putting it together, DateTime.Now.Year
is a concise way to get the current year. It’s commonly used when you need the current year in various applications, such as logging, timestamping, or any situation where the current year’s information is relevant.
Here’s a simple example in a console application:
using System;
class Program
{
static void Main()
{
// Get the current year using DateTime.Now.Year property
int currentYear = DateTime.Now.Year;
// Display the current year
Console.WriteLine("The current year is: " + currentYear);
}
}
Output:
The current year is: 2023
This program uses DateTime.Now
to get the current date and time and then accesses the Year
property to retrieve and display the current year. As seen in the output, utilizing this property got the current year successfully.
Use the DateTime.UtcNow.Year
Property to Get the Current Year in C#
Each time zone has a different local time and is often further modified by daylight saving to avoid confusion about time zones; that’s where UTC comes to the rescue, and by using DateTime.UtcNow
, you can get the universal coordinated time and year in C#.
The UTC is local time plus the bias (the difference between UTC and local time) that you can code by scratch or use the pre-defined System’s DateTime.UtcNow
property.
The DateTime.UtcNow
property returns a DateTime
object representing the current Coordinated Universal Time (UTC). The DateTime
structure has a property called Year
that allows you to extract the year component from a DateTime
object.
Here’s an explanation of the expression DateTime.UtcNow.Year
:
-
DateTime.UtcNow
: Similar toDateTime.Now
,DateTime.UtcNow
returns aDateTime
object representing the current date and time. However, it returns the time in Coordinated Universal Time (UTC) rather than the local time zone of the computer. -
.Year
: Once you have aDateTime
object, you can access various properties to get specific components of the date and time. TheYear
property specifically retrieves the year component from theDateTime
object.
Putting it together, DateTime.UtcNow.Year
is a concise way to get the current year in UTC. It’s commonly used in scenarios where a standardized global time reference is needed, such as in distributed systems or when working with data across different time zones.
Here’s a simple example in a console application:
using System;
class Program
{
static void Main()
{
// Get the current year in UTC using DateTime.UtcNow.Year property
int currentYearUtc = DateTime.UtcNow.Year;
// Display the current year in UTC
Console.WriteLine("The current year in UTC is: " + currentYearUtc);
}
}
Output:
The current year in UTC is: 2023
This program uses DateTime.UtcNow
to get the current date and time in UTC and then accesses the Year
property to retrieve and display the current year.
Always remember that the DateTime.UtcNow
property represents the Greenwich MeanTime and does not depend on the time zone of the current computer configuration. Optimizing your DateTime
cache with this method is possible as it prevents excessive time queries.
Use the DatimeTime.Today.Year
Property to Get the Current Year in C#
The DateTime.Today
property returns a DateTime
object representing the current date with the time portion set to midnight (00:00:00
). Similar to DateTime.Now
, the DateTime.Today
property can be used to work with the date component only.
To extract the current year using DateTime.Today
, you can use the Year
property. Here’s an explanation:
-
DateTime.Today
: TheToday
property of theDateTime
structure returns aDateTime
object representing the current date with the time set to midnight. -
.Year
: Like withDateTime.Now
, theYear
property allows you to retrieve the year component from theDateTime
object.
Putting it together, DateTime.Today.Year
is used to get the current year from the current date without the time component. This is particularly useful when you don’t need precise time information, such as when working with date-based comparisons or logging events on a daily basis.
Here’s a simple example in a console application:
using System;
class Program
{
static void Main()
{
// Get the current year using DateTime.Today.Year property
int currentYear = DateTime.Today.Year;
// Display the current year
Console.WriteLine("The current year is: " + currentYear);
}
}
Output:
The current year is: 2023
Using the DateTime.Today
property gives you the current year in the yyyy
format. As the DateTime
property belongs to the System
, you can get any year from 0001
Anno Domini or common era to December 32, 9999
A.D. (C.E.) in the Gregorian calendar.
It represents an instant in time and provides various aspects of the date and time as you can create an object of the DateTime
property and set it to the current local date by doing Console.WriteLine
.
The purpose of the example code above is to display the current year on the console. It retrieves the current date without the time using DateTime.Today
and then extracts the year using the Year
property.
The result is printed to the console using Console.WriteLine
. This can be useful in scenarios where you need to work with the current year in a program, such as for date-related calculations or user interfaces.
Conclusion
In C# programming, knowing the current year is essential, and this guide covered simple ways to do it.
In the examples provided for all the methods, each approach offers a simple way to get the current year, depending on your application’s needs. Whether you’re focusing on local time, global standards, or just the date, these techniques empower you to manage time effectively in C#.
Remember, understanding these methods makes you better equipped to build smart and practical applications. As you continue your journey in C# programming, the insights from this guide will serve you well.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub