How to Parse Command Line Arguments in C#
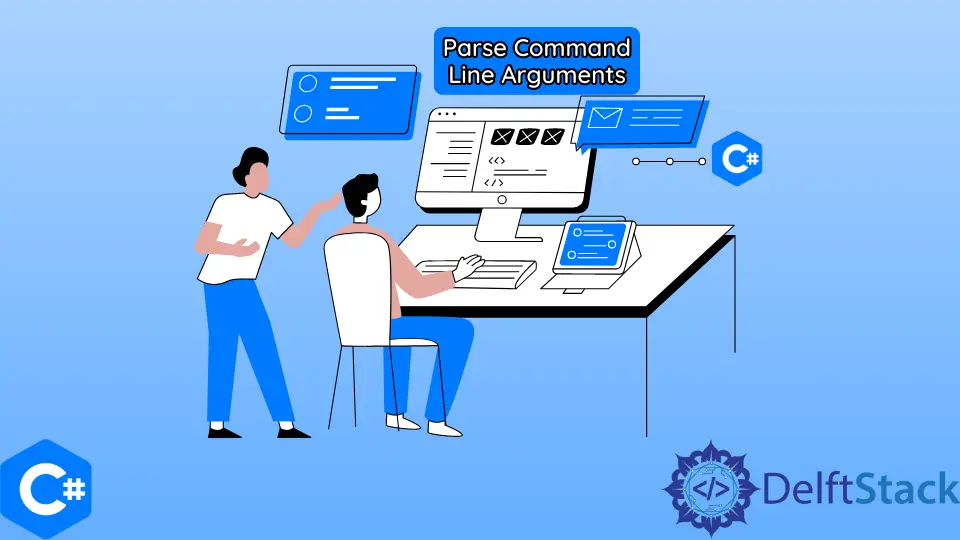
This post will introduce how to parse command-line arguments using the C# programming language. We will investigate two approaches and strategies to complete this objective.
The standard format for a command is commandName -argumentName argumentValue
, where commandName
is the command’s name. Take, for instance, the well-known apt
command used in Linux.
apt install exiftool
Use the CommandLineParser
to Parse Arguments in C#
Instead of manually parsing commands and arguments, you could use a library like CommandLineParser
to perform the task for you, so you don’t have to worry about it. Your primary concentration may then go to put the command logic into action.
We’ll show you how to develop code that allows CommandLineParser
to receive arguments and execute your commands in the manner that follows.
static void Main(string[] args) {
Parser.Default.ParseArguments<UpdateCommand, InstallCommand>(args).WithParsed<ICommand>(
t => t.Execute());
}
First, you must install the NuGet
package, which can be done by executing the following command.
Install-Package CommandLineParser
Create a base class or interface for your commands if they do not already exist. On the other hand, building an interface enables you to use only a single .WithParsed<ICommand>
in your code.
public interface ICommand {
void Execute();
}
UpdateCommand
must be added first, then the Verb
attribute. This parameter instructs CommandLineParser
to produce a UpdateCommand
object when it encounters the word update
.
[Verb("update", HelpText = "updates your system to the newest version")]
public class UpdateCommand : ICommand {
public void Execute() {
Console.WriteLine("Updating the system");
}
}
Put in the InstallCommand
at this very moment and the install
command’s message
parameter. Include the Message
property and the Option
attribute as a direct consequence.
This attribute provides the CommandLineParser
with instructions on converting arguments into properties.
[Verb("install", HelpText = "installing your software")]
public class InstallCommand : ICommand {
[Option("message", Required = true, HelpText = "installing the program")]
public string Message { get; set; }
public void Execute() {
Console.WriteLine($"Executing install for the : {Message}");
}
}
Lastly, pass the arguments to CommandLineParser
as type parameters to parse them.
Use If-Else
Statement to Parse Arguments in C#
Below is an example of parsing the command line arguments.
static void Main(string[] args) {
if (!(args.Length != 0)) {
Console.WriteLine("arguments not correct");
return;
}
}
The purpose of the line that reads static void Main(string[] args)
is to get the arguments from the command line and save them in the args
array. The if (!(args.Length != 0))
line checks if the length of total arguments is 0 or not.
If the length is 0, it indicates that no command-line arguments were supplied to the program.
The line that reads Console.WriteLine("arguments not correct");
shows on the screen that the arguments are incorrect so that the user will input the correct number of arguments the next time.
var c = args[0];
The var c = args[0];
line creates the variable name c
and stores the first argument passed in the command line.
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
We will determine whether or not the variable c
is provided with the argument update
at the beginning of the arguments sent through the command line. The update()
function is called if the answer is affirmative.
The install
condition checks to see whether the c
variable includes the install
argument at the beginning of it, and if it does, it determines whether or not it should have two arguments by comparing the length of the args
variable to 2.
Therefore, we verify that inside the parameter list of the parameters given to it through the command line.
If the length of the args
is 2, it calls the install()
function with args[1]
as the parameter.
The else
case will be executed whenever none of the other cases will match, at which point it will print out on the screen Invalid Command
.
static void update() {
Console.WriteLine("updating your system");
}
The update()
function prints "Updating your system"
when the update
is sent as an argument.
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
The install()
function prints "Installing the program {message}"
when the install
is sent as an argument.
Full Source Code:
using System;
class CommandLineParser {
static void Main(string[] args) {
if ((args.Length == 0)) {
Console.WriteLine("arguments not correct");
return;
}
var c = args[0];
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
}
static void update() {
Console.WriteLine("updating your system");
}
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn