C#에서 명령줄 인수 구문 분석
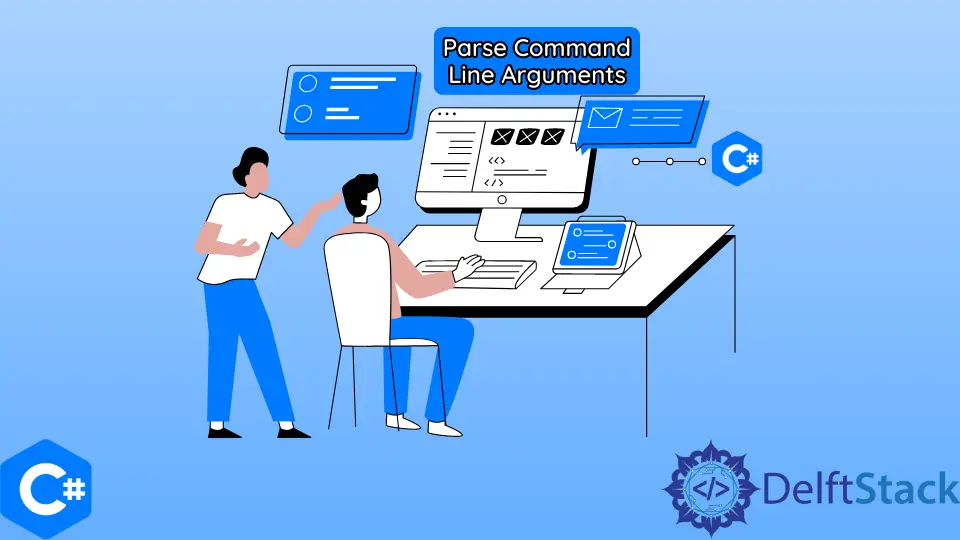
이 게시물은 C# 프로그래밍 언어를 사용하여 명령줄 인수를 구문 분석하는 방법을 소개합니다. 우리는 이 목표를 완료하기 위해 두 가지 접근 방식과 전략을 조사할 것입니다.
명령의 표준 형식은 commandName -argumentName argumentValue
이며, 여기서 commandName
은 명령 이름입니다. 예를 들어 Linux에서 사용되는 잘 알려진 apt
명령을 살펴보겠습니다.
apt install exiftool
CommandLineParser
를 사용하여 C#
의 인수 구문 분석
명령과 인수를 수동으로 구문 분석하는 대신 CommandLineParser
와 같은 라이브러리를 사용하여 작업을 수행할 수 있으므로 걱정할 필요가 없습니다. 당신의 일차 집중은 명령 논리를 행동으로 옮기기 위해 갈 수 있습니다.
CommandLineParser
가 인수를 수신하고 다음과 같은 방식으로 명령을 실행할 수 있도록 하는 코드를 개발하는 방법을 보여드리겠습니다.
static void Main(string[] args) {
Parser.Default.ParseArguments<UpdateCommand, InstallCommand>(args).WithParsed<ICommand>(
t => t.Execute());
}
먼저 NuGet
패키지를 설치해야 합니다. 이 패키지는 다음 명령을 실행하여 수행할 수 있습니다.
Install-Package CommandLineParser
명령에 대한 기본 클래스 또는 인터페이스가 아직 없는 경우 생성합니다. 반면에 인터페이스를 구축하면 코드에서 .WithParsed<ICommand>
하나만 사용할 수 있습니다.
public interface ICommand {
void Execute();
}
UpdateCommand
를 먼저 추가한 다음 Verb
속성을 추가해야 합니다. 이 매개변수는 update
라는 단어를 만나면 UpdateCommand
개체를 생성하도록 CommandLineParser
에 지시합니다.
[Verb("update", HelpText = "updates your system to the newest version")]
public class UpdateCommand : ICommand {
public void Execute() {
Console.WriteLine("Updating the system");
}
}
바로 이 순간에 InstallCommand
와 install
명령의 message
매개변수를 입력합니다. 직접적인 결과로 Message
속성과 Option
속성을 포함합니다.
이 속성은 CommandLineParser
에 인수를 속성으로 변환하는 지침을 제공합니다.
[Verb("install", HelpText = "installing your software")]
public class InstallCommand : ICommand {
[Option("message", Required = true, HelpText = "installing the program")]
public string Message { get; set; }
public void Execute() {
Console.WriteLine($"Executing install for the : {Message}");
}
}
마지막으로 CommandLineParser
에 인수를 유형 매개변수로 전달하여 구문 분석합니다.
If-Else
문을 사용하여 C#
의 인수 구문 분석
다음은 명령줄 인수를 구문 분석하는 예입니다.
static void Main(string[] args) {
if (!(args.Length != 0)) {
Console.WriteLine("arguments not correct");
return;
}
}
static void Main(string[] args)
를 읽는 줄의 목적은 명령줄에서 인수를 가져와 args
배열에 저장하는 것입니다. if (!(args.Length != 0))
행은 총 인수의 길이가 0인지 여부를 확인합니다.
길이가 0이면 프로그램에 명령줄 인수가 제공되지 않았음을 나타냅니다.
Console.WriteLine("arguments not correct");
사용자가 다음에 올바른 수의 인수를 입력할 수 있도록 인수가 올바르지 않음을 화면에 표시합니다.
var c = args[0];
var c = args[0];
line은 변수 이름 c
를 만들고 명령줄에 전달된 첫 번째 인수를 저장합니다.
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
변수 c
가 명령줄을 통해 전송된 인수의 시작 부분에 update
인수와 함께 제공되는지 여부를 결정합니다. 대답이 긍정적이면 update()
함수가 호출됩니다.
install
조건은 c
변수의 시작 부분에 install
인수가 포함되어 있는지 확인하고 포함되면 args
변수의 길이를 2에 비교하여 두 개의 인수를 포함해야 하는지 여부를 결정합니다.
따라서 명령줄을 통해 제공된 매개변수의 매개변수 목록 내부를 확인합니다.
args
의 길이가 2이면 args[1]
를 매개변수로 사용하여 install()
함수를 호출합니다.
else
케이스는 다른 케이스와 일치하지 않을 때마다 실행되며, 이 시점에서 Invalid Command
화면에 인쇄됩니다.
static void update() {
Console.WriteLine("updating your system");
}
update()
함수는 update
가 인수로 전송될 때 "Updating your system"
을 인쇄합니다.
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
install()
함수는 install
이 인수로 전송될 때 "Installing the program {message}"
를 인쇄합니다.
전체 소스 코드:
using System;
class CommandLineParser {
static void Main(string[] args) {
if ((args.Length == 0)) {
Console.WriteLine("arguments not correct");
return;
}
var c = args[0];
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
}
static void update() {
Console.WriteLine("updating your system");
}
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn