在 C# 中解析命令行参数
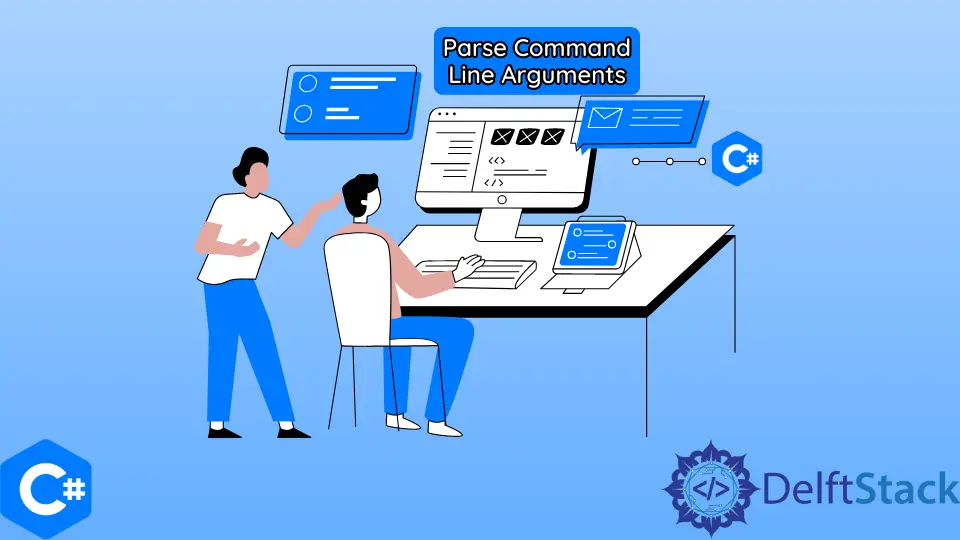
这篇文章将介绍使用 C# 编程语言解析命令行参数。我们将研究两种方法和策略来完成这一目标。
命令的标准格式是 commandName -argumentName argumentValue
,其中 commandName
是命令的名称。以 Linux 中使用的著名的 apt
命令为例。
apt install exiftool
在 C#
使用 CommandLineParser
中解析参数
你可以使用诸如 CommandLineParser
之类的库来为你执行任务,而不是手动解析命令和参数,因此你不必担心它。然后你的主要注意力可能会去把命令逻辑付诸行动。
我们将向你展示如何开发允许 CommandLineParser
接收参数并以以下方式执行你的命令的代码。
static void Main(string[] args) {
Parser.Default.ParseArguments<UpdateCommand, InstallCommand>(args).WithParsed<ICommand>(
t => t.Execute());
}
首先,你必须安装 NuGet
包,可以通过执行以下命令来完成。
Install-Package CommandLineParser
如果你的命令不存在,请为它们创建一个基类或接口。另一方面,构建接口使你可以在代码中仅使用一个 .WithParsed<ICommand>
。
public interface ICommand {
void Execute();
}
必须先添加 UpdateCommand
,然后添加 Verb
属性。此参数指示 CommandLineParser
在遇到单词 update
时生成一个 UpdateCommand
对象。
[Verb("update", HelpText = "updates your system to the newest version")]
public class UpdateCommand : ICommand {
public void Execute() {
Console.WriteLine("Updating the system");
}
}
此时放入 InstallCommand
和 install
命令的 message
参数。包括 Message
属性和 Option
属性作为直接结果。
此属性为 CommandLineParser
提供有关将参数转换为属性的说明。
[Verb("install", HelpText = "installing your software")]
public class InstallCommand : ICommand {
[Option("message", Required = true, HelpText = "installing the program")]
public string Message { get; set; }
public void Execute() {
Console.WriteLine($"Executing install for the : {Message}");
}
}
最后,将参数作为类型参数传递给 CommandLineParser
以解析它们。
在 C#
中使用 If-Else
语句解析参数
下面是解析命令行参数的示例。
static void Main(string[] args) {
if (!(args.Length != 0)) {
Console.WriteLine("arguments not correct");
return;
}
}
读取 static void Main(string[] args)
的行的目的是从命令行获取参数并将它们保存在 args
数组中。if (!(args.Length != 0))
行检查总参数的长度是否为 0。
如果长度为 0,则表明没有向程序提供命令行参数。
读取 Console.WriteLine("arguments not correct");
的行在屏幕上显示参数不正确,以便用户下次输入正确数量的参数。
var c = args[0];
var c = args[0];
line 创建变量名 c
并存储在命令行中传递的第一个参数。
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
我们将确定变量 c
是否在通过命令行发送的参数的开头提供参数 update
。如果答案是肯定的,则调用 update()
函数。
install
条件检查 c
变量是否在其开头包含 install
参数,如果包含,则通过比较 args
的长度来确定它是否应该有两个参数变量为 2。
因此,我们通过命令行验证参数列表里面给它的参数。
如果 args
的长度为 2,它会调用 install()
函数,并将 args[1]
作为参数。
只要其他情况都不匹配,就会执行 else
情况,此时它将在屏幕上打印出 Invalid Command
。
static void update() {
Console.WriteLine("updating your system");
}
当 update
作为参数发送时,update()
函数会打印 "Updating your system"
。
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
当 install
作为参数发送时,install()
函数会打印 "Installing the program {message}"
。
完整源代码:
using System;
class CommandLineParser {
static void Main(string[] args) {
if ((args.Length == 0)) {
Console.WriteLine("arguments not correct");
return;
}
var c = args[0];
if (c == "update") {
update();
} else if (c == "install") {
if (args.Length == 2)
install(args[1]);
} else {
Console.WriteLine("Invalid Command");
}
}
static void update() {
Console.WriteLine("updating your system");
}
static void install(string message) {
Console.WriteLine($"installing the program {message}");
}
}
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn