How to Check Internet Connection in C#
-
Use
GetIsNetworkAvailable()
to Check the Internet Connection inC#
-
Use
InternetGetConnectedState(wininet)
to Check the Internet Connection inC#
-
Ping an IP Address to Check the Internet Connection in
C#
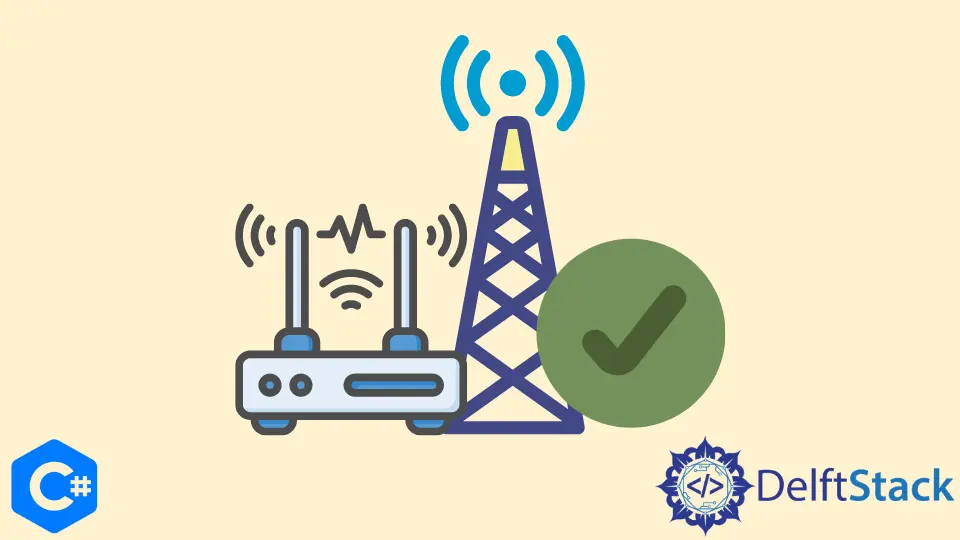
This article explains how to check if the internet connection is available or not in C# Programming Language.
In some use cases, we will need to use C# code in Windows apps to determine whether or not an internet connection is accessible. It may be to download or upload a file via the internet in Windows Forms; alternatively, it could be to acquire some data from a database located in a distant area.
Either way, an internet check is required in these circumstances.
There are a few different approaches to checking the accessibility of the internet using C#. We will look at all of these different techniques with their implementation.
Use GetIsNetworkAvailable()
to Check the Internet Connection in C#
The GetIsNetworkAvailable()
method will be used in the following example to determine whether or not a connection to a network is accessible.
Source Code:
using System.Net.NetworkInformation;
public class CheckInternetConnecition {
static void Main(string[] args) {
if (NetworkInterface.GetIsNetworkAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
The program will output the true
case if the internet connection is available.
The internet connection is available.
If the computer cannot establish a connection to the internet, the code will produce a false
case.
The internet connection is not available. Please connect to the internet and try again.
Use InternetGetConnectedState(wininet)
to Check the Internet Connection in C#
The wininet
application programming interface (API) may determine whether the local machine has an operational internet connection.
The System.Runtime.InteropServices
namespace is utilized for this, and the DllImport
tool is used to import the wininet.dll
file.
After this, construct a Boolean variable with extern static
and a function called InternetGetConnectedState
. This function should have two parameters: description
and reservedValue
.
Next, you must write a method that returns a Boolean value and uses the name IsInternetAvailable
. This method will use the function that was just discussed, which returns the internet status of the local system.
Source Code:
using System.Runtime.InteropServices;
public class PingIP {
[DllImport("wininet.dll")]
private extern static bool InternetGetConnectedState(out int desc, int resValue);
public static bool IsInternetAvailable() {
int desc;
return InternetGetConnectedState(out desc, 0);
}
static void Main(string[] args) {
if (IsInternetAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
};
}
}
The program will output the true
case if the internet connection is available.
The internet connection is available.
If the computer cannot establish a connection to the internet, the code will produce a false
case.
The internet connection is not available. Please connect to the internet and try again.
Ping an IP Address to Check the Internet Connection in C#
Ping
is a class that applications may use to determine whether or not a remote machine is accessible. Its ability to effectively reach a distant host somewhat depends on the network’s topology.
The hostname or address and a timeout value are sent to the Ping
class’s Send()
method. Sending a message will result in a PingReply
object being returned if the message is successfully received.
If an ICMP echo request is sent and a satisfactory response is received, the Status
value will be set to Success
.
Source Code:
using System.Net.NetworkInformation;
public class PingIP {
static void Main(string[] args) {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.10.16", 1000);
if (reply.Status == IPStatus.Success) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
The program will output the true
case if the internet connection is available.
The internet connection is available.
If the computer cannot establish a connection to the internet, the code will produce a false
case.
The internet connection is not available. Please connect to the internet and try again.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn