C#에서 인터넷 연결 확인
-
GetIsNetworkAvailable()
을 사용하여C#
에서 인터넷 연결 확인 -
InternetGetConnectedState(wininet)
를 사용하여C#
에서 인터넷 연결 확인 -
C#
에서 인터넷 연결을 확인하기 위해 IP 주소를 핑합니다.
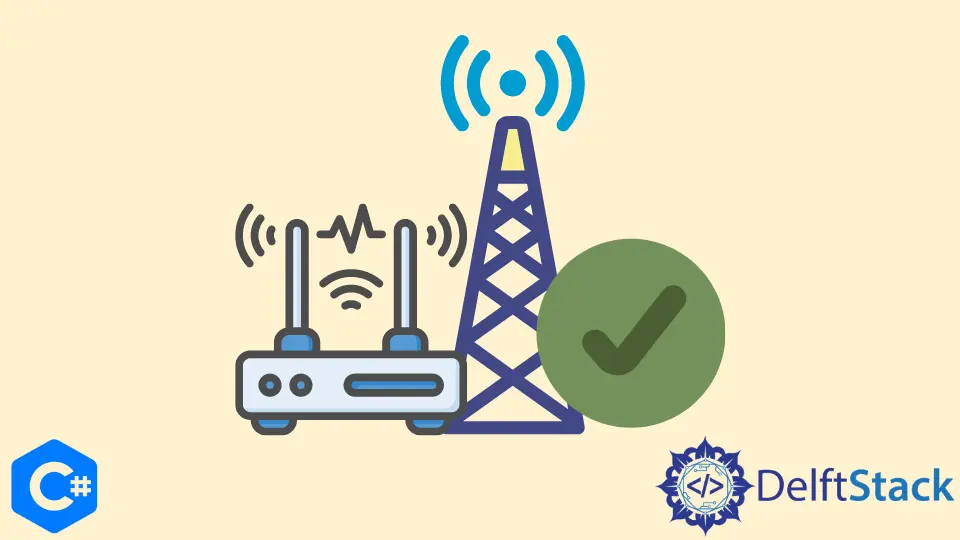
이 문서에서는 C# 프로그래밍 언어에서 인터넷 연결이 가능한지 확인하는 방법에 대해 설명합니다.
일부 사용 사례에서는 Windows 앱에서 C# 코드를 사용하여 인터넷 연결에 액세스할 수 있는지 여부를 확인해야 합니다. Windows Forms에서 인터넷을 통해 파일을 다운로드하거나 업로드하는 것일 수 있습니다. 또는 먼 지역에 위치한 데이터베이스에서 일부 데이터를 수집하는 것일 수 있습니다.
어느 쪽이든 이러한 상황에서는 인터넷 확인이 필요합니다.
C#을 사용하여 인터넷의 접근성을 확인하는 몇 가지 접근 방식이 있습니다. 구현과 함께 이러한 다양한 기술을 모두 살펴보겠습니다.
GetIsNetworkAvailable()
을 사용하여 C#
에서 인터넷 연결 확인
다음 예제에서는 GetIsNetworkAvailable()
메서드를 사용하여 네트워크 연결에 액세스할 수 있는지 여부를 결정합니다.
소스 코드:
using System.Net.NetworkInformation;
public class CheckInternetConnecition {
static void Main(string[] args) {
if (NetworkInterface.GetIsNetworkAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
프로그램은 인터넷 연결이 가능한 경우 true
사례를 출력합니다.
The internet connection is available.
컴퓨터가 인터넷에 연결할 수 없는 경우 코드는 거짓
사례를 생성합니다.
The internet connection is not available. Please connect to the internet and try again.
InternetGetConnectedState(wininet)
를 사용하여 C#
에서 인터넷 연결 확인
wininet
애플리케이션 프로그래밍 인터페이스(API)는 로컬 시스템이 인터넷에 연결되어 있는지 여부를 결정할 수 있습니다.
이를 위해 System.Runtime.InteropServices
네임스페이스를 사용하고 DllImport
도구를 사용하여 wininet.dll
파일을 가져옵니다.
그런 다음 extern static
및 InternetGetConnectedState
라는 함수를 사용하여 부울 변수를 생성합니다. 이 함수에는 description
및 reservedValue
라는 두 개의 매개변수가 있어야 합니다.
다음으로 부울 값을 반환하고 IsInternetAvailable
이라는 이름을 사용하는 메서드를 작성해야 합니다. 이 메서드는 방금 논의한 로컬 시스템의 인터넷 상태를 반환하는 함수를 사용합니다.
소스 코드:
using System.Runtime.InteropServices;
public class PingIP {
[DllImport("wininet.dll")]
private extern static bool InternetGetConnectedState(out int desc, int resValue);
public static bool IsInternetAvailable() {
int desc;
return InternetGetConnectedState(out desc, 0);
}
static void Main(string[] args) {
if (IsInternetAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
};
}
}
프로그램은 인터넷 연결이 가능한 경우 true
사례를 출력합니다.
The internet connection is available.
컴퓨터가 인터넷에 연결할 수 없는 경우 코드는 거짓
케이스를 생성합니다.
The internet connection is not available. Please connect to the internet and try again.
C#
에서 인터넷 연결을 확인하기 위해 IP 주소를 핑합니다.
‘Ping’은 응용 프로그램이 원격 시스템에 액세스할 수 있는지 여부를 결정하는 데 사용할 수 있는 클래스입니다. 멀리 떨어진 호스트에 효과적으로 도달하는 능력은 네트워크의 토폴로지에 따라 다소 달라집니다.
호스트 이름 또는 주소 및 시간 초과 값은 Ping
클래스의 Send()
메서드로 전송됩니다. 메시지를 보내면 메시지가 성공적으로 수신되면 PingReply
개체가 반환됩니다.
ICMP 에코 요청이 전송되고 만족스러운 응답이 수신되면 Status
값이 Success
로 설정됩니다.
소스 코드:
using System.Net.NetworkInformation;
public class PingIP {
static void Main(string[] args) {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.10.16", 1000);
if (reply.Status == IPStatus.Success) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
프로그램은 인터넷 연결이 가능한 경우 true
사례를 출력합니다.
The internet connection is available.
컴퓨터가 인터넷에 연결할 수 없는 경우 코드는 거짓
케이스를 생성합니다.
The internet connection is not available. Please connect to the internet and try again.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn