C# でインターネット接続を確認する
-
C#
でGetIsNetworkAvailable()
を使用してインターネット接続を確認する -
C#
でInternetGetConnectedState(wininet)
を使用してインターネット接続を確認する -
C#
で IP アドレスに ping を実行してインターネット接続を確認する
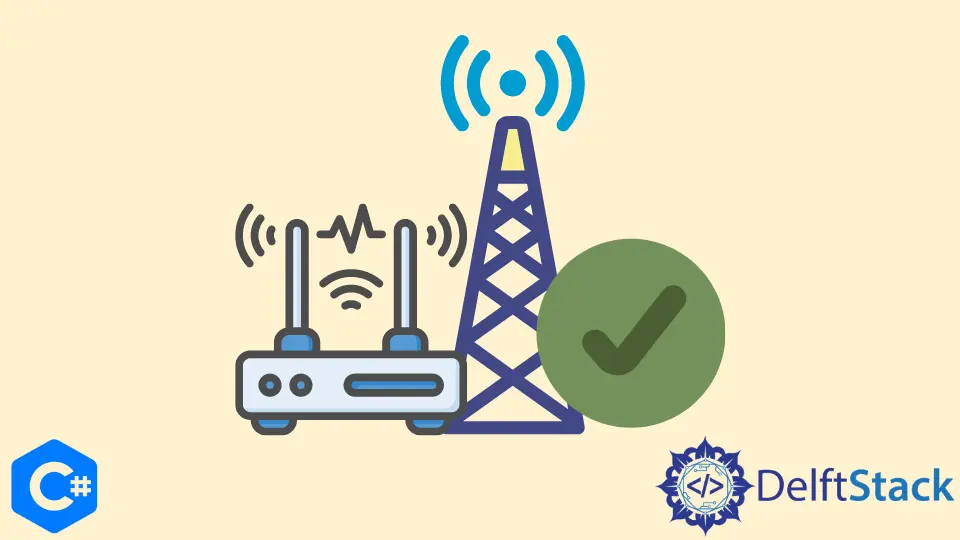
この記事では、C# プログラミング言語でインターネット接続が使用可能かどうかを確認する方法について説明します。
一部のユース ケースでは、Windows アプリで C# コードを使用して、インターネット接続にアクセスできるかどうかを判断する必要があります。 Windows フォームでインターネットを介してファイルをダウンロードまたはアップロードする場合があります。 または、離れた場所にあるデータベースからデータを取得することもできます。
いずれにせよ、このような状況ではインターネット チェックが必要です。
C# を使用してインターネットのアクセシビリティをチェックするには、いくつかの方法があります。 これらのさまざまな手法をすべて、実装とともに見ていきます。
C#
で GetIsNetworkAvailable()
を使用してインターネット接続を確認する
次の例では、GetIsNetworkAvailable()
メソッドを使用して、ネットワークへの接続にアクセスできるかどうかを判断します。
ソースコード:
using System.Net.NetworkInformation;
public class CheckInternetConnecition {
static void Main(string[] args) {
if (NetworkInterface.GetIsNetworkAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
インターネット接続が利用可能な場合、プログラムは true
ケースを出力します。
The internet connection is available.
コンピュータがインターネットへの接続を確立できない場合、コードは false
ケースを生成します。
The internet connection is not available. Please connect to the internet and try again.
C#
で InternetGetConnectedState(wininet)
を使用してインターネット接続を確認する
wininet
アプリケーション プログラミング インターフェース (API) は、ローカル マシンがインターネットに接続しているかどうかを判断する場合があります。
これには、System.Runtime.InteropServices
名前空間が使用され、DllImport
ツールを使用して wininet.dll
ファイルがインポートされます。
この後、extern static
と InternetGetConnectedState
という関数を使用してブール変数を作成します。 この関数には、description
と reservedValue
の 2つのパラメーターが必要です。
次に、ブール値を返し、IsInternetAvailable
という名前を使用するメソッドを作成する必要があります。 このメソッドは、ローカル システムのインターネット ステータスを返す、先ほど説明した関数を使用します。
ソースコード:
using System.Runtime.InteropServices;
public class PingIP {
[DllImport("wininet.dll")]
private extern static bool InternetGetConnectedState(out int desc, int resValue);
public static bool IsInternetAvailable() {
int desc;
return InternetGetConnectedState(out desc, 0);
}
static void Main(string[] args) {
if (IsInternetAvailable()) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
};
}
}
インターネット接続が利用可能な場合、プログラムは true
ケースを出力します。
The internet connection is available.
コンピュータがインターネットへの接続を確立できない場合、コードは false
ケースを生成します。
The internet connection is not available. Please connect to the internet and try again.
C#
で IP アドレスに ping を実行してインターネット接続を確認する
Ping
は、アプリケーションがリモート マシンにアクセスできるかどうかを判断するために使用できるクラスです。 遠く離れたホストに効果的に到達できるかどうかは、ネットワークのトポロジに多少依存します。
ホスト名またはアドレスとタイムアウト値は、Ping
クラスの Send()
メソッドに送信されます。 メッセージを送信すると、メッセージが正常に受信された場合、PingReply
オブジェクトが返されます。
ICMP エコー要求が送信され、満足のいく応答が受信された場合、Status
値は Success
に設定されます。
ソースコード:
using System.Net.NetworkInformation;
public class PingIP {
static void Main(string[] args) {
Ping myPing = new Ping();
PingReply reply = myPing.Send("192.168.10.16", 1000);
if (reply.Status == IPStatus.Success) {
Console.WriteLine("The internet connection is available.");
} else {
Console.WriteLine(
"The internet connection is not available. Please connect to the internet and try again.");
}
}
}
インターネット接続が利用可能な場合、プログラムは true
ケースを出力します。
The internet connection is available.
コンピュータがインターネットへの接続を確立できない場合、コードは false
ケースを生成します。
The internet connection is not available. Please connect to the internet and try again.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn