Struct in Class in C++
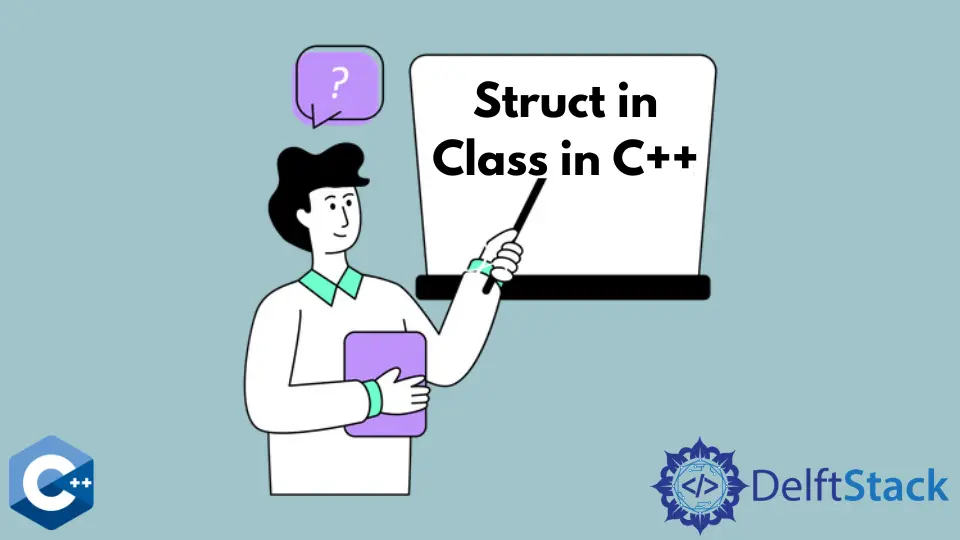
We use arrays whenever we need to store a group of similar data types. However, if there arises a situation where we need to store a group of the non-similar type of data, then we use the structure and class data types.
This article will explain the structure and the class and how to use a structure inside a class.
Struct in Class in C++
A structure is a user-defined data type used to store non-similar types of data. The keyword struct
declares it.
On the other hand, a class is also a user-defined data type to store non-similar types of data. The keyword class
denotes it.
However, the major difference between them is that the class hides the implementation details of its members and by default makes all the members of a class private, whereas a structure does not hide the implementation details of its members and by default makes all its members as public.
Now let us understand how to use a structure and a class individually in C++.
Struct in C++
We declare a structure in C++ using the struct
keyword, followed by declaring all its data members. Let us see a code example to understand it better.
#include <iostream>
using namespace std;
struct X {
int a;
};
int main() {
X x;
x.a = 10;
cout << x.a;
}
Output:
10
In the above code example, we declare a structure X
, inside which we declare a variable a
. Now, we create an instance of this structure and then try to access the variable a
by this instance x
in the main method; the structure can easily access it and change its value because all its members are, by default, public.
Class in C++
We declare a class in C++ by the class
keyword, followed by declaring the data members of the class. Let us understand the usage of a class in C++ with the help of code.
#include <iostream>
using namespace std;
class X {
int a;
};
int main() {
X x;
x.a = 10;
cout << x.a;
}
Output:
./ex.cpp: In function 'int main)':
./ex.cpp:23:9: error: 'int X::a' is private
int a;
^
./ex.cpp:28:7: error: within this context
x.a = 10;
^
./ex.cpp:23:9: error: 'int X::a' is private
int a;
^
./ex.cpp:29:13: error: within this context
cout<<x.a;
^
In the above code example, we have made a class followed by declaring a data member inside it as variable a
. However, we cannot access this data member inside our main function because the members of a class are by default private.
Therefore, it cannot be accessed and throws an error.
Use the Struct in a Class in C++
We have seen how to make a struct and a class individually. Now let us understand how we will use a struct inside a class in C++.
We will understand this concept with the help of a code example.
#include <iostream>
using namespace std;
class X {
public:
struct T {
int a;
};
};
int main() {
X x;
x.a = 10;
cout << x.a;
}
Output:
./ex.cpp: In function 'int main)':
./ex.cpp:15:7: error: 'class X' has no member named 'a'
x.a = 10;
^
./ex.cpp:16:13: error: 'class X' has no member named 'a'
cout<<x.a;
^
As you can see, the above code throws an error when we try to access the struct variable a
in our main method. This is because we have only made an instance of the class and call our variable by the class’ instance.
We did not even make the instance of the structure. Therefore, the program throws an error that does not determine the variable a
.
Now, we will be discussing how to overcome this error. As you have seen in the struct and class examples, you need to make an instance of a structure and a class before accessing its members.
The same concept goes when you are using the struct inside a class. Let’s understand this with the help of a code example.
#include <iostream>
using namespace std;
class X {
public:
struct T {
int a;
};
T t;
};
int main() {
X x;
x.t.a = 10;
cout << x.t.a;
}
Output:
10
Therefore, we have now made an instance of the structure inside the class itself and access the struct variable a
with the help of both the class and the struct’s instance.
Conclusion
In this article, we have seen the usage of the class as well as the structure in C++. Both are user-defined data types; however, the only difference between them is that the members of a class are by default private, whereas the members of a structure are by default public.
Afterward, we also discussed the problem we usually encounter while using a struct in a class.