Struct Inheritance in C++
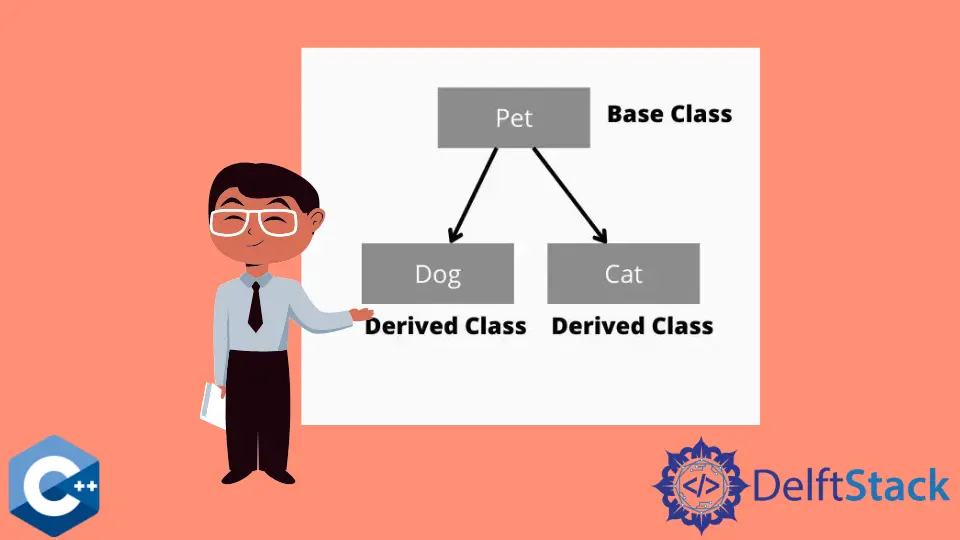
Structs and classes are similar, but the difference comes in their accessibility to other classes or functions in object-oriented programming.
Structs are by default specified as public, whereas classes are private. And in inheritance, we cannot inherit private-specified classes; we have to manually declare a class public, whereas structs are by default public, so we can easily inherit them.
What is Inheritance in C++
Inheritance is one of the core concepts of Object-Oriented Programming, and the reason behind it is that it improves the code reusability and creation of hierarchical classification.
With the help of inheritance, you can define a general class that defines some functions to perform some basic operations and extend that to other classes to inherit those functions. Let’s understand it through the diagram.
In this example, we have Dog
and Cat
, and both are different animals, but in the end, both belong to the animal pet category.
Both of these will have some same features like eating, sleeping, and walking that can be stored in the Pet
class, also called the base class, while features specific to them as sound and size can be defined in the Dog
and Cat
class, also called derived class.
And this type of inheritance is known as Single Inheritance. There are other different types which we will discuss one by one below.
Code Example:
#include <iostream>
using namespace std;
// Base class
struct Pet {
void eat() { cout << "I can eat i am pet \n"; }
void sleep() { cout << "I can sleep i am pet \n"; }
void walk() { cout << "I can walk i am pet \n"; }
};
// Derived class
struct Cat : Pet {
void sound() { cout << "I am a cat Meow Meow! \n"; }
};
// Derived class
struct Dog : Pet {
void sound() { cout << "I am a dog Bark Bark! \n"; }
};
int main() {
cout << "This is Cat's characteristics" << endl;
Cat myCat;
myCat.eat();
myCat.sleep();
myCat.walk();
myCat.sound();
cout << "\nThis is Dog's characteristics" << endl;
Dog myDog;
myDog.eat();
myDog.sleep();
myDog.walk();
myDog.sound();
}
Output:
This is Cat's characteristics
I can eat i am pet
I can sleep i am pet
I can walk i am pet
I am a cat Meow Meow!
This is Dog's characteristics
I can eat i am pet
I can sleep i am pet
I can walk i am pet
I am a dog Bark Bark!
In this example, Pet
is a base class where Cat
and Dog
are derived classes. Pet
has defined some common behaviors of cats and dogs, so we don’t define them in every class to avoid code redundancy.
Dog
and Cat
extend those common behaviors and define their specific behavior in their own derived classes.
Class Dog
and Cat
has only one behavior, sound()
, while the other comes from the base class. And the object of these derived classes is using the other functions of the base class.
Types of Inheritance in C++
C++ supports different types of inheritance, which gives an open choice to use each according to the scenario. Let’s have a look at each one by one.
- Single inheritance
- Multiple inheritance
- Multi-level inheritance
- Hierarchical inheritance
- Hybrid inheritance
Single Inheritance
In single inheritance, we have one base class and one derived class. This type is already explained in the first example.
Syntax:
class derivedClass_name : access_mode base_class {
// body of the derived class
};
Multiple Inheritance
In multiple inheritance, we can inherit the function and properties of more than one base class into a single derived class.
Syntax:
class derivedClass_name : access_mode base_class1,
access_mode base_class2,
....{
// body of the derived class
};
Multi-Level Inheritance
Every type of inheritance allows you to inherit from a base class, but multi-level inheritance has something more than that. It allows you to do multi-level inheritance, allowing you to inherit from the derived class to other subclasses.
Animals
and pets
are base classes, and cat
is the derived class inheriting functions and properties from both the base classes.
Hierarchical Inheritance
In hierarchical inheritance, more than one subclasses are inherited from a single base class in a hierarchy. And also, more than one derived class is created from a single base class.
This is an advanced type of inheritance.
Hybrid Inheritance
As known by its name hybrid inheritance, this type of inheritance comprises more different types of inheritance like hierarchical and multiple inheritances. Any combination can be done for hybrid inheritance.
In this example, we have two different types of inheritance, single and hierarchical inheritance, made as a hybrid inheritance.
Struct Inheritance in C++
In C++, a struct
is a keyword used to define a structure similar to a class but has minor differences. The core difference between a struct and a class is that the members of a struct are by default public, whereas the class has private, leading to security concerns.
A structure isn’t much secure because the functions and properties are not hidden from the end-users. On the other hand, classes are secure because they can hide programming implementations from the end-users.
General syntax of struct:
struct structureName {
member1;
member2;
..memberN;
};
Since inheritance is a core feature of object-oriented programming, structures can also use this feature. Inheritance with structure needs the struct
keyword instead of class.
Struct Inheritance in C++:
#include <iostream>
using namespace std;
// Base class
struct Pet {
void eat() { cout << "I can eat i am pet \n"; }
};
struct Dog : Pet {
void sound() { cout << "I am a dog Bark Bark! \n"; }
};
int main() {
cout << "\nThis is Dog's characteristics" << endl;
Dog myDog;
myDog.eat();
myDog.sound();
}
Output:
This is Dog's characteristics
I can eat i am pet
I am a dog Bark Bark!
We have used the word struct
before the structure name; it looks similar to classes if you noticed we hadn’t used the word public
in the structure’s body because struct is by default public.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn