C++ Struct Default Values Initialization
- Understanding Structs in C++
- Initializing Default Values with Brace-or-Equal-Initializers
- Customizing Default Values in Structs
- Advantages of Using Brace-or-Equal-Initializers
- Conclusion
- FAQ
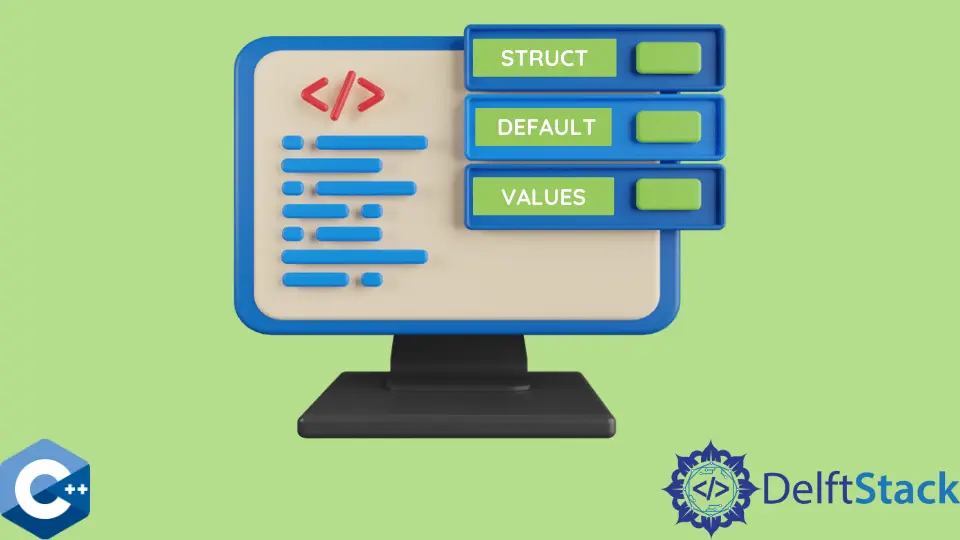
When working with structs in C++, it’s essential to understand how to initialize default values effectively. One of the most powerful features introduced in C++11 is the brace-or-equal-initializers, which allow developers to assign default values to struct members directly within the struct definition. This feature simplifies the code and enhances readability, making it easier to manage data structures.
In this article, we will explore how to use brace-or-equal-initializers to initialize default values in structs, along with practical examples that illustrate their application. Whether you’re a seasoned developer or just starting with C++, mastering this concept will undoubtedly improve your programming skills and efficiency.
Understanding Structs in C++
Structs in C++ are user-defined data types that group variables of different types under a single name. They are similar to classes but have default public access. Structs are particularly beneficial when you want to encapsulate related data. By using brace-or-equal-initializers, you can set default values for struct members, which can be particularly helpful in scenarios where you want to ensure certain variables always have a defined state.
Basic Syntax of Structs
The basic syntax for defining a struct in C++ is straightforward. Here’s a quick overview:
struct StructName {
DataType member1;
DataType member2;
// Other members
};
This structure allows you to create instances of StructName
that can hold various types of data.
Initializing Default Values with Brace-or-Equal-Initializers
The brace-or-equal-initializers offer a clean and efficient way to assign default values to struct members. This feature allows you to specify default values directly in the struct definition, making your code more maintainable and less error-prone.
Example of Default Value Initialization
Here’s a simple example that demonstrates how to use brace-or-equal-initializers to set default values for a struct:
#include <iostream>
#include <string>
struct Person {
std::string name = "John Doe";
int age = 30;
};
int main() {
Person person;
std::cout << "Name: " << person.name << ", Age: " << person.age << std::endl;
return 0;
}
Output:
Name: John Doe, Age: 30
In this example, the Person
struct has two members: name
and age
. Both members have default values assigned. When an instance of Person
is created, it automatically initializes name
to “John Doe” and age
to 30. This approach eliminates the need for additional constructor logic to set default values, making the code cleaner and easier to read.
Customizing Default Values in Structs
While default values are handy, there may be situations where you want to customize them when creating an instance of the struct. You can achieve this by defining a constructor alongside the default values.
Example with Custom Constructor
Here’s an example that illustrates how to customize default values using a constructor:
#include <iostream>
#include <string>
struct Person {
std::string name = "John Doe";
int age = 30;
Person(std::string n, int a) : name(n), age(a) {}
};
int main() {
Person person("Alice", 25);
std::cout << "Name: " << person.name << ", Age: " << person.age << std::endl;
return 0;
}
Output:
Name: Alice, Age: 25
In this code, the Person
struct has a constructor that allows you to pass custom values for name
and age
. When creating an instance of Person
, you can specify these values. If you omit the constructor, the default values will be used instead.
Advantages of Using Brace-or-Equal-Initializers
Using brace-or-equal-initializers for default values in structs comes with several advantages:
- Clarity: It’s immediately clear what the default values are, enhancing code readability.
- Reduced Boilerplate: You eliminate the need for separate constructors just to assign default values.
- Consistency: Default values are always initialized, reducing the chances of uninitialized variables leading to undefined behavior.
Example of Structs with Multiple Members
To further illustrate the flexibility of brace-or-equal-initializers, consider a struct with multiple members:
#include <iostream>
struct Rectangle {
double length = 1.0;
double width = 1.0;
double area() {
return length * width;
}
};
int main() {
Rectangle rect;
std::cout << "Area of rectangle: " << rect.area() << std::endl;
return 0;
}
Output:
Area of rectangle: 1
In this example, the Rectangle
struct has default values for both length
and width
. The area
method calculates the area based on these values. When you create a Rectangle
instance, it automatically initializes the dimensions, allowing you to compute the area without any additional setup.
Conclusion
Understanding how to initialize default values in C++ structs using brace-or-equal-initializers is a valuable skill for any developer. This feature not only simplifies your code but also enhances readability and maintenance. By incorporating default values directly into your struct definitions, you can streamline your development process and reduce the risk of errors associated with uninitialized variables. As you continue to explore C++, mastering these concepts will undoubtedly elevate your programming capabilities.
FAQ
-
What are structs in C++?
Structs are user-defined data types that group variables of different types under a single name, similar to classes but with default public access. -
What are brace-or-equal-initializers?
Brace-or-equal-initializers are a feature introduced in C++11 that allows developers to assign default values to struct members directly in the struct definition. -
How do I customize default values in a struct?
You can customize default values by defining a constructor in the struct that accepts parameters for the members you want to initialize. -
What are the advantages of using default values in structs?
Advantages include clarity, reduced boilerplate code, and consistency in ensuring that variables are always initialized. -
Can I have a method in a struct?
Yes, structs in C++ can contain methods, just like classes, allowing you to define behaviors associated with the data.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook