STL Algorithms in C++
-
Use the
std::sort
Algorithm to Sort Generic Vector Ranges in C++ -
Use the
std::reverse
Algorithm to Reverse the Order of Elements in C++ -
Use the
std::accumulate
Algorithm to Calculate the Sum of Elements in a Range in C++ -
Use the
std::count
Algorithm to Count the Number of Elements Satisfying the Specific Criteria in C++
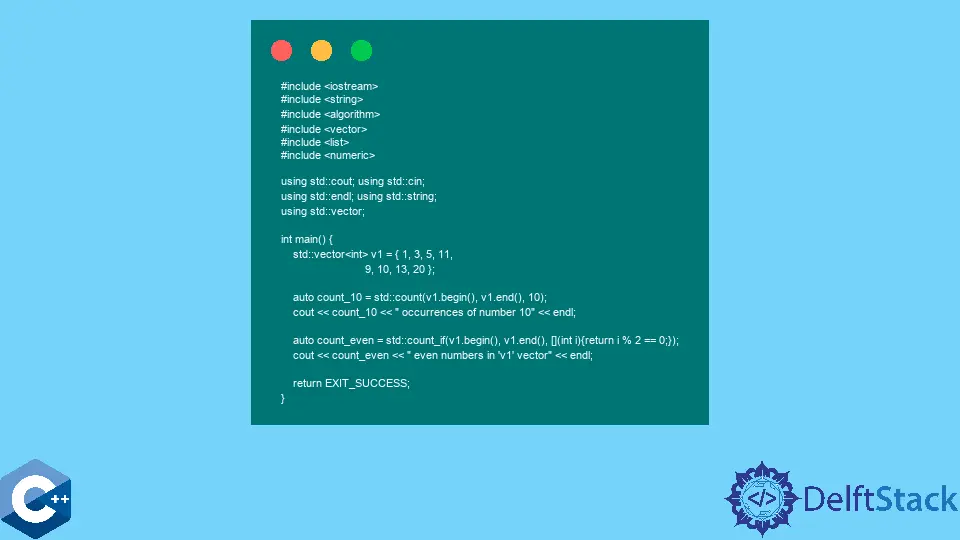
This article will introduce several functions from the STL algorithms library in C++.
Use the std::sort
Algorithm to Sort Generic Vector Ranges in C++
std::sort
is one of the most utilized algorithms in STL. It has multiple overloads, the simplest of which accepts two iterators that meet the LegacyRandomAccessIterator
requirements and sorts elements in non-descending order. The latter is said because the order of equal elements is not guaranteed to be preserved.
std::sort
is commonly used on vector
ranges. The following code snippet demonstrates the usage as such. The algorithm can optionally take the comparison function, which will be used to evaluate element pairs and sort the range correspondingly.
The following example shows one line where the STL function object - std::greater
is passed as the comparison function. Alternatively, one can define a custom function object or specify the lambda expression directly as the third parameter of std::sort
.
#include <algorithm>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
std::sort(v1.begin(), v1.end());
printRange(v1);
std::sort(v1.begin(), v1.end(), std::greater<>());
printRange(v1);
std::sort(v1.begin(), v1.end(), [](int a, int b) { return (a - 2) != b; });
printRange(v1);
return EXIT_SUCCESS;
}
Output:
1, 3, 5, 9, 10, 11, 13, 20,
20, 13, 11, 10, 9, 5, 3, 1,
1, 3, 5, 9, 10, 11, 13, 20
Use the std::reverse
Algorithm to Reverse the Order of Elements in C++
std::reverse
can be used to reverse the contents of sequence containers such as vector
, list
or deque
. The function accepts two iterator parameters and can operate on any generic type. The following code sample shows two scenarios where vector
of integers and list
of strings are reversed. We also sort the list
object using the sort
member function before invoking the std::reverse
algorithm. Note that, std::sort
algorithm does not work on std::list
container.
#include <algorithm>
#include <iostream>
#include <list>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::list;
using std::string;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
template <typename T>
void printRange(std::list<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
std::list<string> l1 = {"htop", "wtop", "rtop", "ktop", "ktop", "ptop"};
std::reverse(v1.begin(), v1.end());
printRange(v1);
l1.sort();
std::reverse(l1.begin(), l1.end());
printRange(l1);
return EXIT_SUCCESS;
}
Output:
20, 13, 10, 9, 11, 5, 3, 1,
wtop, rtop, ptop, ktop, ktop, htop,
Use the std::accumulate
Algorithm to Calculate the Sum of Elements in a Range in C++
std::accumulate
is part of numeric algorithms that can be utilized to conduct common arithmetic operations on every element of the given range. In this case, the given algorithm calculates the total sum of each element in the range. std::accumulate
has two overloads, the first of which takes the two iterators denoting the range itself and the value init
, which represents the starting value for the summation. The second overload can optionally take a function object as the fourth parameter applied instead of summation.
#include <algorithm>
#include <iostream>
#include <numeric>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
auto sum = std::accumulate(v1.begin(), v1.end(), 0);
cout << "Sum of 'v1' vector = " << sum << endl;
sum = std::accumulate(v1.begin(), v1.end(), 1, std::multiplies());
cout << "Accumulate of 'v1' vector = " << sum << endl;
return EXIT_SUCCESS;
}
Output:
Sum of 'v1' vector = 72
Accumulate of 'v1' vector = 3861000
Use the std::count
Algorithm to Count the Number of Elements Satisfying the Specific Criteria in C++
std::count
function is a useful method to count specific elements in the given range. Namely, we can pass the range iterators and the value
to match all the elements that are equal to the given value. Another overload can accept a unary predicate that returns evaluate the valid matches, and the algorithm retrieves the count number correspondingly. In the next example, we specified a lambda expression to count even numbers in the vector
object.
#include <algorithm>
#include <iostream>
#include <list>
#include <numeric>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
auto count_10 = std::count(v1.begin(), v1.end(), 10);
cout << count_10 << " occurrences of number 10" << endl;
auto count_even =
std::count_if(v1.begin(), v1.end(), [](int i) { return i % 2 == 0; });
cout << count_even << " even numbers in 'v1' vector" << endl;
return EXIT_SUCCESS;
}
Output:
1 occurrences of number 10
2 even numbers in 'v1' vector
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook