The std::find_if Algorithm C++
-
Use the
std::find_if
Function to Search for Elements Satisfying the Given Criteria -
Use the
std::find_first_of
Function to Search for Element Matches in Two Ranges -
Use the
find_end
Function to Split the String With Delimiters
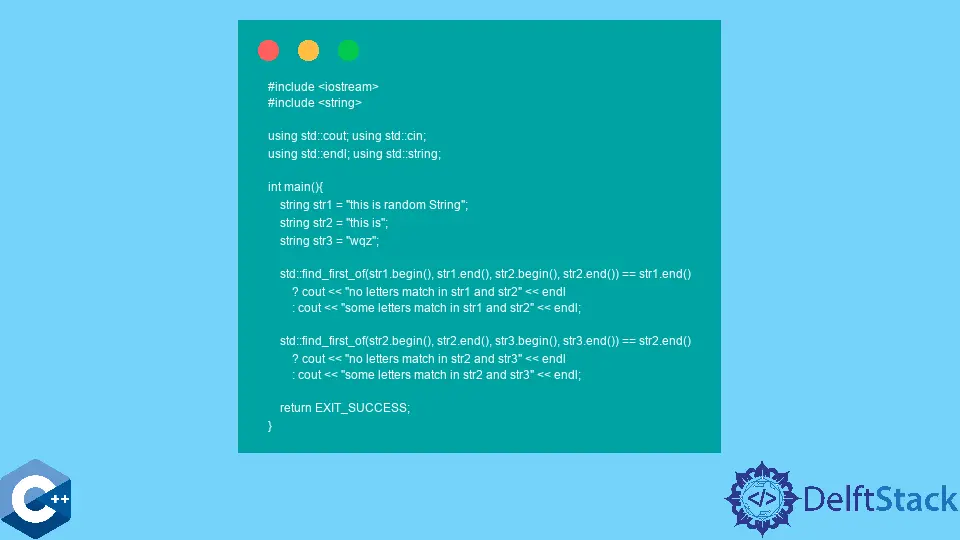
This article will demonstrate how to utilize the std::find_if
algorithm from C++ Standard Template Library.
Use the std::find_if
Function to Search for Elements Satisfying the Given Criteria
The std::find_if
function is part of STL algorithms, and it provides a searching method for elements in the range satisfying the given condition. Namely, the condition is specified as a callable object that returns a bool
value.
The basic overload of the std::find_if
function accepts two iterators denoting the range that needs to be searched. The third parameter represents the callable object used to evaluate the elements in the range. Note that range iterators should at least satisfy LegacyInputIterator
requirements.
std::find_if
returns the iterator to the first element satisfying the given criteria or the second argument iterator if no such element is found.
In the following code snippet, we employ the std::find_if
algorithm on std::string
objects and use the isupper
function as the callable object. Consequently, the function should help us determine if the string contains only lowercase letters or not.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str1 = "this is random String";
string str2 = "this is";
string str3 = "wqz";
std::find_if(begin(str1), end(str1), isupper) != end(str1)
? cout << "str1 contains uppercase letters" << endl
: cout << "str1 contains only lowercase letters" << endl;
std::find_if(begin(str2), end(str2), isupper) != end(str2)
? cout << "str2 contains uppercase letters" << endl
: cout << "str2 contains only lowercase letters" << endl;
return EXIT_SUCCESS;
}
Output:
str1 contains uppercase letters
str2 contains only lowercase letters
Use the std::find_first_of
Function to Search for Element Matches in Two Ranges
std::find_first_of
is another powerful algorithm from STL that can be utilized to search for the same elements in two given ranges. The functions accept four iterators as parameters, the first two of which denote the range that needs to be searched for elements passed as the last two iterator arguments.
The latter two iterators must meet the requirements of LegacyForwardIterator
. In this case, we use the std::find_first_of
algorithm to check if the characters match in two strings. The function’s return value is the iterator to the first element in the searched range that matches an element from the second range. If no such element is found, the second iterator of the searched ranges is returned.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str1 = "this is random String";
string str2 = "this is";
string str3 = "wqz";
std::find_first_of(str1.begin(), str1.end(), str2.begin(), str2.end()) ==
str1.end()
? cout << "no letters match in str1 and str2" << endl
: cout << "some letters match in str1 and str2" << endl;
std::find_first_of(str2.begin(), str2.end(), str3.begin(), str3.end()) ==
str2.end()
? cout << "no letters match in str2 and str3" << endl
: cout << "some letters match in str2 and str3" << endl;
return EXIT_SUCCESS;
}
Output:
some letters match in str1 and str2
no letters match in str2 and str3
Use the find_end
Function to Split the String With Delimiters
On the other hand, we have the find_end
algorithm to search for the last occurrence of the given range sequence in another range. Like the std::find_first_of
, this algorithm accepts four iterators denoting both ranges, but it tries to find the exact sequence match in the first range.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string str1 = "this is random String";
string str2 = "this is";
string str3 = "wqz";
auto ret = std::find_end(str1.begin(), str1.end(), str2.begin(), str2.end());
ret == str1.end() ? cout << "no such sequence found" << endl
: cout << "last occurrence found at "
<< std::distance(str1.begin(), ret) << endl;
ret = std::find_end(str2.begin(), str2.end(), str3.begin(), str3.end());
ret == str2.end() ? cout << "no such sequence found" << endl
: cout << "last occurrence found at "
<< std::distance(str2.begin(), ret) << endl;
return EXIT_SUCCESS;
}
Output:
last occurrence found at 0
no such sequence found
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook