Maze Solver in C++
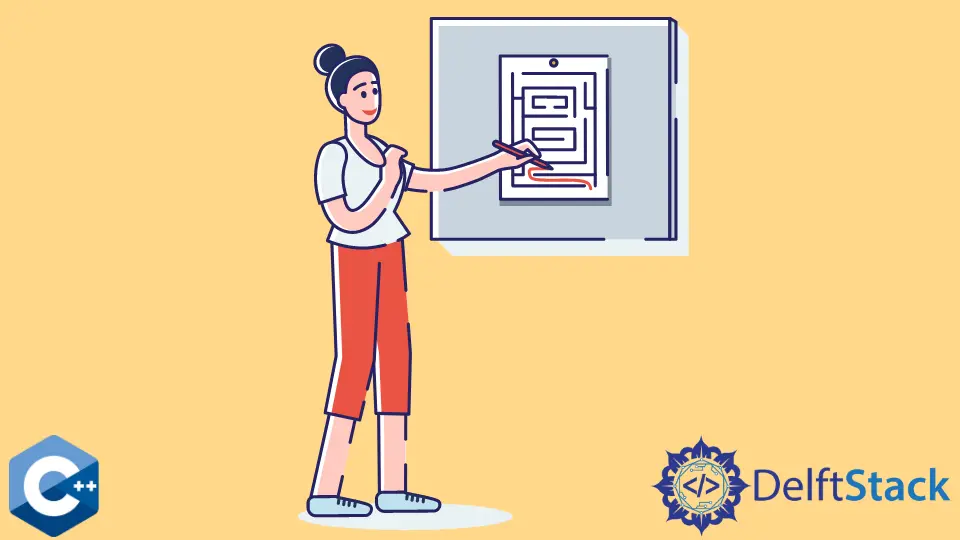
Maze solving algorithm is a classical problem in computer science and artificial intelligence.
Maze Solver in C++
The maze-solving algorithm aims to find the shortest path from start to finish by moving only in the four compass directions. The algorithm maintains two sets of information: the open list, which contains all the nodes that have yet to be visited, and the closed list, which contains all the nodes that have been visited.
To find a path from one node to another node in the maze, we need to do these things:
-
Check whether an open node is adjacent (left or right) to the current node.
-
If not, check whether a closed node is adjacent (left or right) to the current node.
-
If not, go back one step and go through steps 1 and 2 again.
Example of the Maze Solving Algorithm:
#include <iostream>
using namespace std;
bool demo_maze(char maze[9][9], bool sam[9][9], int x, int y, int u, int v) {
if (x == u && y == v) {
sam[x][y] = true;
for (int t = 0; t <= u; t++) {
for (int f = 0; f <= v; f++) {
cout << sam[t][f] << " ";
}
cout << endl;
}
cout << endl;
return true;
}
if (x > u || y > v) return false;
if (maze[x][y] == 'X') return false;
sam[x][y] = true;
bool right = demo_maze(maze, sam, x, y + 1, u, v);
bool down = demo_maze(maze, sam, x + 1, y, u, v);
sam[x][y] = false;
if (right || down) return true;
return false;
}
int main() {
char maze[9][9] = {"0000", "00X0", "000X", "0X00"};
bool sam[9][9] = {0};
int u = 3, v = 3;
bool ans = demo_maze(maze, sam, 0, 0, u, v);
}
Click here to check the working of the code as mentioned above.
Uses of the Maze Solver in C++
The maze-solving algorithm can be used for many purposes, including chess, minesweeper, and finding paths through mazes or other obstacles on a map. This algorithm can decide which way to go when you are lost and looking for your way out of a forest or maze!
The maze-solving algorithm is also used for 2D games, but it would take more time than other algorithms because of its computational complexity. It uses a recursive function that checks the coordinates of the player and then moves him to the next spot.
A recursive function calls itself, either directly or indirectly. It can be used to solve problems of recursion, and it can also be used to solve problems where the solution is not easily expressed as a short loop.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook