C++ 中的 STL 演算法
-
使用
std::sort
演算法在 C++ 中對通用向量範圍進行排序 -
使用
std::reverse
演算法反轉 C++ 中的元素順序 -
在 C++ 中使用
std::accumulate
演算法計算範圍內元素的總和 -
使用
std::count
演算法計算 C++ 中滿足特定條件的元素數量
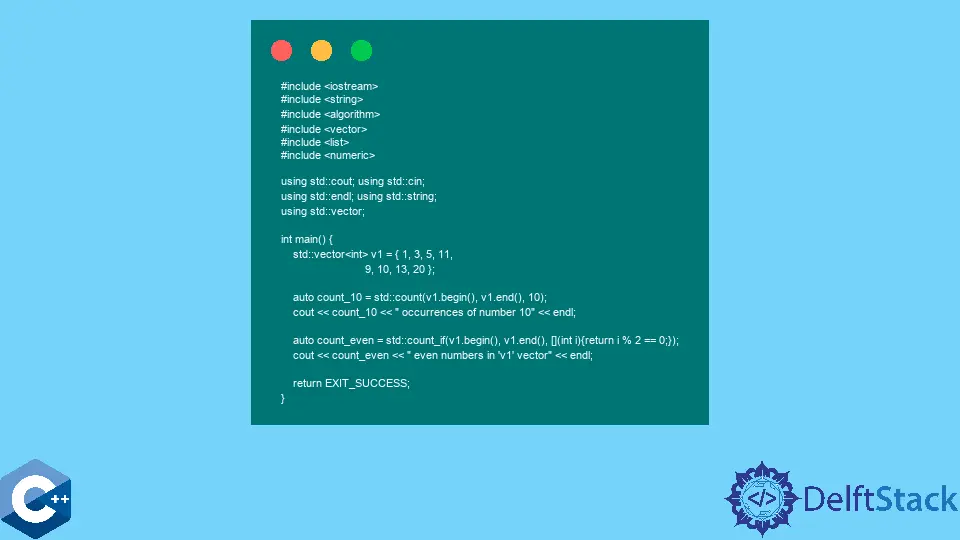
本文將介紹 C++ 中 STL 演算法庫中的幾個函式。
使用 std::sort
演算法在 C++ 中對通用向量範圍進行排序
std::sort
是 STL 中最常用的演算法之一。它有多個過載,其中最簡單的一個接受兩個滿足 LegacyRandomAccessIterator
要求的迭代器,並按非降序對元素進行排序。之所以說後者,是因為不能保證相等元素的順序。
std::sort
通常用於 vector
範圍。以下程式碼片段演示了這種用法。該演算法可以選擇採用比較函式,該函式將用於評估元素對並相應地對範圍進行排序。
以下示例顯示了 STL 函式物件 - std::greater
作為比較函式傳遞的一行。或者,可以定義自定義函式物件或直接將 lambda 表示式指定為 std::sort
的第三個引數。
#include <algorithm>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
std::sort(v1.begin(), v1.end());
printRange(v1);
std::sort(v1.begin(), v1.end(), std::greater<>());
printRange(v1);
std::sort(v1.begin(), v1.end(), [](int a, int b) { return (a - 2) != b; });
printRange(v1);
return EXIT_SUCCESS;
}
輸出:
1, 3, 5, 9, 10, 11, 13, 20,
20, 13, 11, 10, 9, 5, 3, 1,
1, 3, 5, 9, 10, 11, 13, 20
使用 std::reverse
演算法反轉 C++ 中的元素順序
std::reverse
可用於反轉序列容器的內容,例如 vector
、list
或 deque
。該函式接受兩個迭代器引數並且可以對任何泛型型別進行操作。以下程式碼示例顯示了兩個場景,其中整數 vector
和字串 list
顛倒。在呼叫 std::reverse
演算法之前,我們還使用 sort
成員函式對 list
物件進行排序。請注意,std::sort
演算法不適用於 std::list
容器。
#include <algorithm>
#include <iostream>
#include <list>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::list;
using std::string;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
template <typename T>
void printRange(std::list<T> v) {
for (const auto &item : v) {
cout << item << ", ";
}
cout << endl;
}
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
std::list<string> l1 = {"htop", "wtop", "rtop", "ktop", "ktop", "ptop"};
std::reverse(v1.begin(), v1.end());
printRange(v1);
l1.sort();
std::reverse(l1.begin(), l1.end());
printRange(l1);
return EXIT_SUCCESS;
}
輸出:
20, 13, 10, 9, 11, 5, 3, 1,
wtop, rtop, ptop, ktop, ktop, htop,
在 C++ 中使用 std::accumulate
演算法計算範圍內元素的總和
std::accumulate
是數值演算法的一部分,可用於對給定範圍的每個元素進行常見的算術運算。在這種情況下,給定演算法計算範圍內每個元素的總和。std::accumulate
有兩個過載,第一個過載表示範圍本身的兩個迭代器和表示求和的起始值的值 init
。第二個過載可以選擇將函式物件作為應用的第四個引數而不是求和。
#include <algorithm>
#include <iostream>
#include <numeric>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
auto sum = std::accumulate(v1.begin(), v1.end(), 0);
cout << "Sum of 'v1' vector = " << sum << endl;
sum = std::accumulate(v1.begin(), v1.end(), 1, std::multiplies());
cout << "Accumulate of 'v1' vector = " << sum << endl;
return EXIT_SUCCESS;
}
輸出:
Sum of 'v1' vector = 72
Accumulate of 'v1' vector = 3861000
使用 std::count
演算法計算 C++ 中滿足特定條件的元素數量
std::count
函式是一種計算給定範圍內特定元素的有用方法。也就是說,我們可以傳遞範圍迭代器和 value
來匹配所有等於給定值的元素。另一個過載可以接受返回評估有效匹配的一元謂詞,並且演算法相應地檢索計數。在下一個示例中,我們指定了一個 lambda 表示式來計算 vector
物件中的偶數。
#include <algorithm>
#include <iostream>
#include <list>
#include <numeric>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
std::vector<int> v1 = {1, 3, 5, 11, 9, 10, 13, 20};
auto count_10 = std::count(v1.begin(), v1.end(), 10);
cout << count_10 << " occurrences of number 10" << endl;
auto count_even =
std::count_if(v1.begin(), v1.end(), [](int i) { return i % 2 == 0; });
cout << count_even << " even numbers in 'v1' vector" << endl;
return EXIT_SUCCESS;
}
輸出:
1 occurrences of number 10
2 even numbers in 'v1' vector