How to Read File Into String in C++
-
Use
std::ifstream
andstd::getline
to Read File Into String in C++ -
Use
istreambuf_iterator
to Read File Into String in C++ -
Use
rdbuf
to Read File Into String in C++ -
Use the
fread
Function to Read File Into String -
Use
read
to Read File Into String - Conclusion
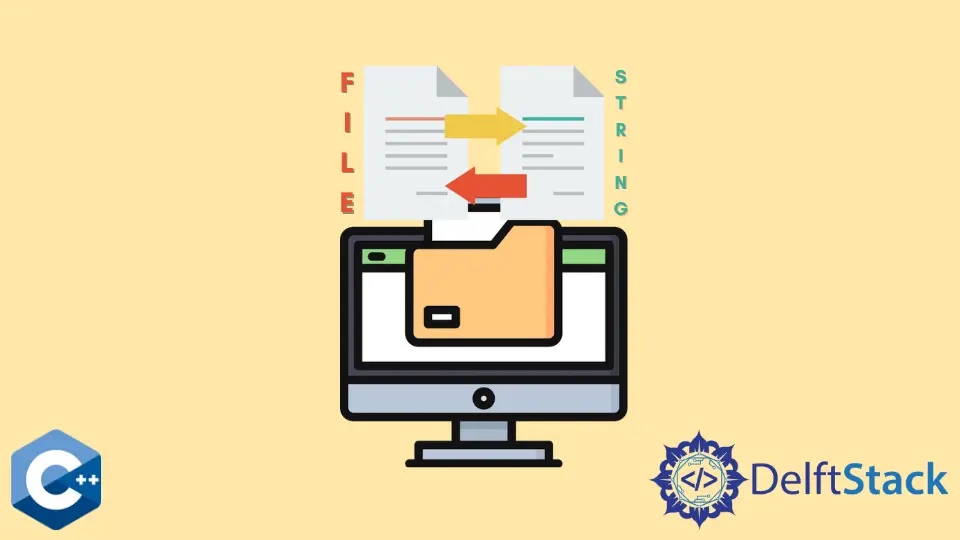
In C++, reading a file into a string is a common operation that involves extracting the contents of a file and storing them as a string variable within a C++ program. This process allows developers to efficiently manipulate and process the file’s content using string manipulation functions provided by the C++ standard library.
In this context, this article explores various approaches and methods for seamlessly reading the contents of a file and populating a C++ string, facilitating versatile file handling and data manipulation within C++ programs.
Use std::ifstream
and std::getline
to Read File Into String in C++
In C++, employing std::ifstream
in combination with std::getline
offers a powerful and versatile mechanism for reading the contents of a file into a string.
This method leverages the Input File Stream (std::ifstream
) class to facilitate seamless file input operations, while std::getline
enables efficient extraction of lines from the file, which can be subsequently concatenated to form a complete string representation.
This approach provides a clean and concise means of assimilating file data into a C++ string, offering developers flexibility and control over the file reading process within their programs.
Syntax:
std::ifstream inputFile("filename.txt");
std::string line;
std::getline(inputFile, line);
Think of std::ifstream
as the file system’s gatekeeper. It helps us create an input file stream, essentially a pathway to a specific file.
This stream becomes our means of accessing the content within that file.
The std::string line
declares a string variable named line in C++, ready to store a line of text from an input operation like std::getline
. Next, the std::getline
is a handy function made for extracting lines from an input stream.
In simpler terms, std::ifstream
opens the door to the file, and std::getline
fetches lines from it. Together, they create a smooth process of turning a file into a neat string.
Let’s take a look at the sample code below:
example.txt
:
this is an example text
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Step 1: Open the file using std::ifstream
std::ifstream inputFile("example.txt");
// Step 2: Check if the file is open
if (!inputFile.is_open()) {
std::cerr << "Error opening the file!" << std::endl;
return 1; // Exit the program with an error code
}
// Step 3: Read the file content into a string using std::getline
std::string fileContent;
std::string line;
while (std::getline(inputFile, line)) {
fileContent += line + "\n"; // Append each line to the string
}
// Step 4: Close the file
inputFile.close();
// Step 5: Display the content of the string
std::cout << "File content:\n" << fileContent;
return 0; // Exit the program successfully
}
In this sample code, we begin by including the necessary header files for input/output operations, file handling, and string manipulation.
Next, we declare a std::ifstream
object named inputFile
to open the file, example.txt
. We check if the file is successfully opened using the is_open
member function.
If it can’t open the file, we print an error message to the standard error stream (std::cerr
) and exit the program with a non-zero error code. If opening the file is successful, we declare two strings: fileContent
and line
.
The fileContent
stores all the content of the file. On the other hand, the line
is used to read each line from the file.
We then use a while
loop with std::getline
to read each line from the file and append it to the fileContent
string. The loop continues until there are no more lines to read.
After reading the file, we close it using the close
member function of the std::ifstream
class. Finally, we display the content of the string fileContent
to the standard output stream (std::cout
).
Output:
File content:
this is an example text
The output of the program is the content of example.txt
. The absence of an error message suggests that the file was opened successfully and the program exited with a success code.
Use istreambuf_iterator
to Read File Into String in C++
Using istreambuf_iterator
to read a file into a string is a method that leverages the capabilities of the Standard Template Library (STL).
This approach involves creating an ifstream
object to open the file and then utilizing istreambuf_iterator
to iterate over the file’s content and construct a string from it. The iterator reads the characters directly from the file’s buffer, allowing for a more concise and efficient way to populate a string with the entire file content.
This method is particularly useful when the goal is to read the entire content of a file into a string without the need for line-by-line processing.
Syntax:
std::ifstream inputFile("example.txt");
std::string fileContent(std::istreambuf_iterator<char>(inputFile), std::istreambuf_iterator<char>());
inputFile.close();
The first line, std::ifstream inputFile("example.txt")
, creates an ifstream
object named inputFile
to open the file example.txt
.
Then, we have istreambuf_iterator
that is used to construct a string named fileContent
by reading all the characters from the example.txt
file inside the inputFile
variable. The inputFile
is then closed after reading.
Let’s take a look at the fully functional code below:
example.txt
:
this is an example text
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Step 1: Open the file
std::ifstream inputFile("example.txt");
// Step 2: Check if the file is open
if (!inputFile.is_open()) {
std::cerr << "Error opening the file!" << std::endl;
return 1; // Exit with an error code
}
// Step 3: Read file content into a string
std::string fileContent((std::istreambuf_iterator<char>(inputFile)), (std::istreambuf_iterator<char>()));
// Step 4: Close the file
inputFile.close();
// Step 5: Display the file content
std::cout << "File content:\n" << fileContent;
return 0; // Exit the program successfully
}
In this program, we are reading the content of a file named example.txt
into a string.
Firstly, we open the file using std::ifstream
, which is specifically designed for file input. If the file opening is unsuccessful, we print an error message to the standard error stream and exit the program with an error code.
Next, we utilize the std::istreambuf_iterator
to read characters from the file. The expression std::istreambuf_iterator<char>(inputFile)
represents the beginning of the file, and std::istreambuf_iterator<char>()
represents the end of the file.
We enclose this iterator range within parentheses as we construct a string
named fileContent
. This ensures efficient reading of the entire file content into the string.
After reading, we close the file using the inputFile.close()
statement, indicating that we have finished working with it. Finally, we display the content of the string to the console using std::cout
and close the program by returning 0
, indicating successful execution.
Output:
File content:
this is an example text
This output confirms that the program successfully read the content of the file into the string and displayed it through the standard output.
Use rdbuf
to Read File Into String in C++
The rdbuf
function is a built-in method to return a pointer to the stream buffer of the file. This method allows for efficient extraction of the entire content of a file and its direct insertion into a string.
By invoking rdbuf()
, the program gains access to the underlying file buffer, enabling a straightforward and concise approach to populate a string with the file’s content. This method is particularly useful when the objective is to read the complete content of a file into a string without the need for line-by-line processing.
To understand this better, take a look at the example code below:
example.txt
:
this is an example text
#include <fstream>
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string filename("example.txt");
std::ifstream input_file(filename);
if (!input_file.is_open()) {
std::cerr << "Could not open the file - '" << filename << "'" << std::endl;
return EXIT_FAILURE;
}
std::ostringstream ss;
ss << input_file.rdbuf();
std::string file_contents = ss.str();
std::cout << file_contents << std::endl;
return EXIT_SUCCESS;
}
In the program above, we are reading the contents of the example.txt
file. We open this file using an ifstream
object named input_file
.
Afterwards, we check if it was successfully opened. If not, an error message is displayed on the standard error stream (cerr
), indicating that the file could not be opened.
The program then returns an exit failure code (EXIT_FAILURE
).
Assuming the file is successfully opened, we proceed to use an ostringstream
named ss
to capture the content of the file. The <<
operator is used to stream the contents of the file (input_file.rdbuf()
) into the ostringstream
.
The content of the file, now stored in the ostringstream
, is converted to a string using the ss.str()
function. This string is assigned to the variable file_contents
.
Finally, the program prints the contents of the file to the standard output stream (cout
). The program concludes by returning an exit success code (EXIT_SUCCESS
).
Output:
this is an example text
The output confirms that the program successfully read the content of the file and displayed it through the standard output.
Use the fread
Function to Read File Into String
Another way to read a file in C++ is using the fread
function, which can significantly enhance performance compared to other methods.
fread
is a C standard library function, not a C++ function. It is used for reading binary data from a file in C.
Syntax:
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
ptr
: Pointer to the block of memory where data will be read into.size
: Size in bytes of each element to be read.count
: Number of elements, each one with a size ofsize
, to be read.stream
: Pointer to aFILE
object that specifies the input stream.
The function returns the total number of elements successfully read, which may be less than (size * count)
if the end of the file is encountered or if an error occurs.
Example:
example.txt
:
this is an example text
#include <cstdlib>
#include <stdio.h>
#include <sys/stat.h>
int main() {
const char *filename = "example.txt";
// Open the file
FILE *file = fopen(filename, "rb");
if (file == NULL) {
perror("Error opening file");
return 1;
}
// Get the file size using stat
struct stat file_info;
if (stat(filename, &file_info) != 0) {
perror("Error getting file size");
fclose(file);
return 1;
}
// Allocate a buffer to store the file content
char *buffer = (char *)malloc(file_info.st_size);
if (buffer == NULL) {
perror("Error allocating memory");
fclose(file);
return 1;
}
// Read the file content into the buffer
size_t items_read = fread(buffer, file_info.st_size, 1, file);
if (items_read != 1) {
perror("Error reading file");
free(buffer);
fclose(file);
return 1;
}
// Close the file
fclose(file);
// Display the content of the buffer (file content)
printf("File content:\n%s\n", buffer);
// Free the allocated memory
free(buffer);
return 0;
}
This program reads the content of a file named example.txt
using the fread
function and determines the file size with the stat
Unix system call.
The program starts by attempting to open the file in binary mode ("rb"
). If the file opening fails, it prints an error message using perror
and exits with a non-zero status.
Next, it uses the stat
function to retrieve information about the file, such as its size. If obtaining file information fails, it prints an error, closes the file, and exits.
After acquiring the file size, the program gets a buffer of the same size to store the file content. If memory allocation fails, it prints an error, closes the file, and exits.
The program then reads the entire content of the file into the allocated buffer using fread
. If the reading operation encounters an error, it prints an error message, frees the allocated memory, closes the file, and exits.
Following the successful reading, the program closes the file using fclose
.
Finally, it displays the content of the buffer (the file content) to the console using printf
. After displaying the content, it frees the previously allocated memory.
Output:
File content:
this is an example text
The output shows that the program read the content of example.txt
and displayed it on the console.
Use read
to Read File Into String
In C++, you can use the read
function to read a specified number of bytes from a file, but it doesn’t directly read a file into a string as you might expect. Instead, you can read the file into a character array or a vector of characters and then construct a string from that.
Unlike fread
, read
requires a file descriptor to indicate the file to read from. File descriptors are special integers linked to open file streams the program might have during execution.
You can obtain a file descriptor using the open
function, storing it as an int
. The other read
arguments are the buffer pointer for storing data and the number of bytes to read. The latter is obtained through the fstat
function.
Notably, we use string.data
as the buffer to store the read file contents.
Syntax:
read(int fd, void *buf, size_t count)
fd
: File descriptor obtained using the open function.buf
: Pointer to the buffer where the read data will be stored.count
: Number of bytes to read.
Let’s now see this in action with an example.
example.txt
:
this is an example text
#include <fcntl.h>
#include <iostream>
#include <string>
#include <sys/stat.h>
#include <unistd.h>
int main() {
const std::string filename = "example.txt";
// Open file
int fd = open(filename.c_str(), O_RDONLY);
if (fd < 0) {
perror("open");
return EXIT_FAILURE;
}
// Get file size
struct stat sb {};
fstat(fd, &sb);
// Read file into a string
std::string fileContents(sb.st_size, '\0');
if (read(fd, (void *)fileContents.data(), sb.st_size) < 0) {
perror("read");
close(fd);
return EXIT_FAILURE;
}
// Close file
close(fd);
// Print file contents
std::cout << fileContents << std::endl;
return EXIT_SUCCESS;
}
This C++ program reads the contents of a file named example.txt
into a string using low-level file operations.
First, it attempts to open the file using the open
system call with the O_RDONLY
flag, indicating read-only mode. If the file opening fails (returns a file descriptor less than 0), it prints an error message using perror
and exits with a failure status.
Next, it retrieves the file’s size using the fstat
system call, storing the result in the sb
struct.
Then, it creates a std::string
named fileContents
with a size equal to the file size, filled with null characters. This string will later hold the contents of the file.
The program reads the file content into the string using the read
system call, passing the file descriptor, the starting address of the string’s data, and the file size as arguments. If the read operation fails, it prints an error message, closes the file using close
, and exits with a failure status.
Finally, it closes the file using close
, prints the contents of the string to the standard output using std::cout
, and exits with a success status.
Output:
this is an example text
This code demonstrates a low-level approach to reading a file into a string in C++ using system calls.
This output corresponds to the content of the file example.txt
, which is read into the std::string
named fileContents
using low-level file I/O functions (open
, fstat
, read
, and close
). The program then prints the content of the string to the standard output using std::cout
.
The content of the file is directly transferred into the string, and the result is displayed on the console.
Conclusion
In conclusion, this article explores various methods for reading a file into a string in C++, providing developers with versatile options for efficient file handling and data manipulation. The methods covered include std::ifstream
, istreambuf_iterator
, rdbuf
, fread
, and read
.
Each method has its advantages and use cases, catering to different scenarios and preferences. Developers can choose the method that best fits their specific requirements for file handling in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook