Overloaded Constructor in C++
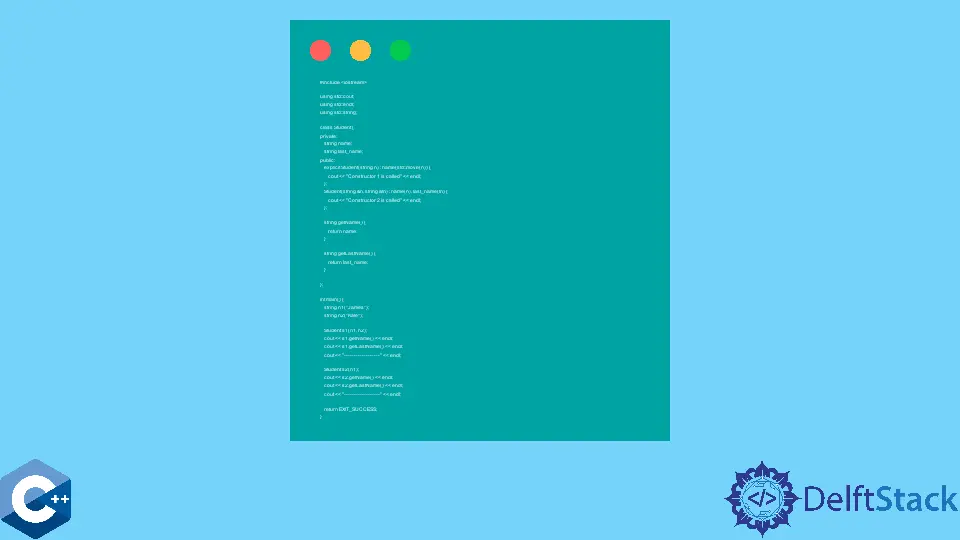
In the world of C++, constructors are special member functions that initialize objects. One powerful feature of constructors is overloading, which allows you to create multiple constructors with different parameters. This flexibility is crucial for building versatile classes that can be instantiated in various ways.
In this article, we’ll dive into the concept of overloaded constructors in C++, explore how to implement them, and provide clear examples to illustrate their usage. Whether you’re a beginner or looking to refine your skills, understanding overloaded constructors will enhance your coding capabilities and make your classes more adaptable.
What is an Overloaded Constructor?
An overloaded constructor is a constructor that has the same name as the class but takes different parameters. This allows you to create instances of a class in different ways, depending on the information available at the time of instantiation. For example, you might have a Rectangle
class that can be initialized with just a width and height or with a single value that sets both dimensions. This versatility makes your code cleaner and more intuitive.
Example of Overloaded Constructor
Let’s take a look at a simple example of how to implement overloaded constructors in C++.
#include <iostream>
using namespace std;
class Rectangle {
public:
int width, height;
Rectangle(int w, int h) {
width = w;
height = h;
}
Rectangle(int side) {
width = height = side;
}
void display() {
cout << "Width: " << width << ", Height: " << height << endl;
}
};
int main() {
Rectangle rect1(10, 5);
Rectangle square(7);
rect1.display();
square.display();
return 0;
}
Output:
Width: 10, Height: 5
Width: 7, Height: 7
In this example, we define a Rectangle
class with two constructors. The first constructor takes two parameters, w
and h
, to initialize the width and height of a rectangle. The second constructor takes a single parameter, side
, which sets both dimensions to the same value, creating a square. The display
method prints the dimensions of the rectangle or square. When we create instances of Rectangle
, we can use either constructor based on our needs.
Benefits of Using Overloaded Constructors
Overloaded constructors offer several advantages that can significantly improve your code’s design and functionality.
-
Flexibility: By allowing different ways to initialize an object, overloaded constructors make your classes more flexible. This means that users of your class can create objects with varying levels of detail, depending on what information they have at hand.
-
Readability: When your constructors are overloaded, your code becomes cleaner and easier to understand. This is because it allows for more intuitive object creation. For instance, a user can easily see that they can create a square by providing a single dimension instead of having to specify both width and height.
-
Enhanced Functionality: With overloaded constructors, you can also set default values or perform specific actions based on the parameters provided. This can be particularly useful in classes that require initialization with optional parameters.
Let’s consider another example that demonstrates these benefits.
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
string name;
int age;
Person(string n) {
name = n;
age = 0;
}
Person(string n, int a) {
name = n;
age = a;
}
void display() {
cout << "Name: " << name << ", Age: " << age << endl;
}
};
int main() {
Person person1("Alice");
Person person2("Bob", 30);
person1.display();
person2.display();
return 0;
}
Output:
Name: Alice, Age: 0
Name: Bob, Age: 30
In this example, the Person
class has two overloaded constructors. The first constructor initializes a Person
object with just a name, setting the age to a default value of 0. The second constructor allows specifying both the name and age. This flexibility makes it easy to create Person
objects in different contexts, enhancing the overall functionality of the class.
Conclusion
Overloaded constructors in C++ are a powerful feature that allows for greater flexibility and readability in your code. By enabling multiple ways to initialize objects, they enhance the functionality of your classes and make them easier to use. Whether you are developing a simple application or a complex system, understanding how to implement and utilize overloaded constructors will undoubtedly improve your programming skills.
By mastering this concept, you can create more adaptable and user-friendly classes, leading to cleaner and more maintainable code. So, the next time you design a class, consider how overloaded constructors can enhance its usability.
FAQ
-
What is an overloaded constructor in C++?
An overloaded constructor is a constructor with the same name as the class but with different parameters, allowing for multiple ways to initialize an object. -
Why should I use overloaded constructors?
Overloaded constructors provide flexibility and improve code readability by allowing different ways to create objects based on available information. -
Can a class have more than two overloaded constructors?
Yes, a class can have multiple overloaded constructors as long as each has a unique parameter list. -
What happens if I don’t provide a constructor in my class?
If you don’t provide any constructors, C++ automatically generates a default constructor that initializes member variables to default values. -
How do overloaded constructors affect memory allocation?
Overloaded constructors do not directly affect memory allocation; they simply provide different ways to initialize an object’s member variables when the object is created.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook