Nested Classes in C++
- What are Nested Classes?
- Characteristics of Nested Classes
- Advantages of Using Nested Classes
- Conclusion
- FAQ
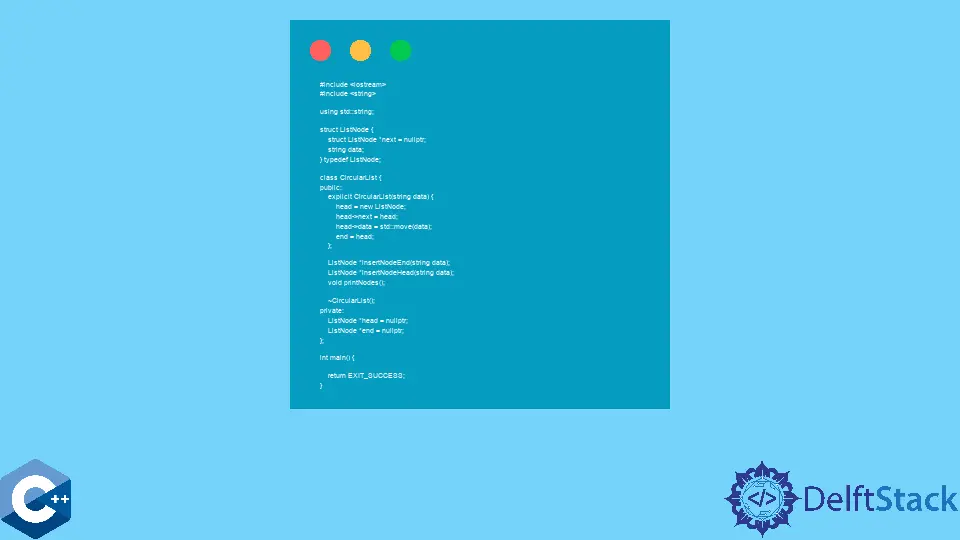
Nested classes in C++ are a powerful feature that allows developers to define classes within other classes. This unique structure can enhance encapsulation, improve code organization, and facilitate access to the enclosing class’s members.
In this article, we will explore the concept of nested classes, their characteristics, and how they can be effectively utilized in C++. Whether you are a beginner or an experienced programmer, understanding nested classes will help you write cleaner and more maintainable code. Let’s dive into the world of nested classes and discover their potential in C++ programming.
What are Nested Classes?
Nested classes are defined within the scope of another class, known as the enclosing class. This relationship allows the nested class to access the private and protected members of the enclosing class. The primary purpose of nested classes is to logically group classes that are only used in one place, thus improving code readability and maintainability.
For example, consider a scenario where you have a class representing a university. Within this class, you might have another class representing students. By nesting the student class within the university class, you clearly indicate that students are a part of the university context.
Here’s a simple example to illustrate this concept:
#include <iostream>
using namespace std;
class University {
public:
class Student {
public:
void display() {
cout << "Student enrolled in the University." << endl;
}
};
};
int main() {
University::Student student;
student.display();
return 0;
}
Output:
Student enrolled in the University.
In this example, the Student
class is nested within the University
class. When we create a Student
object, we can access its method display()
without any issues. This demonstrates how nested classes can help organize related functionalities.
Characteristics of Nested Classes
Nested classes come with several characteristics that set them apart from regular classes. Understanding these characteristics can help you leverage nested classes effectively in your C++ programs.
-
Access Control: A nested class can access the private and protected members of its enclosing class. This feature allows for tighter coupling between the nested class and its enclosing class, enabling better encapsulation.
-
Scope: The scope of a nested class is limited to its enclosing class. This means that you cannot create a nested class object without referencing the enclosing class. This limitation helps to avoid naming conflicts and keeps the code organized.
-
Instantiation: To instantiate a nested class, you must first create an instance of the enclosing class, or you can use the scope resolution operator. This requirement reinforces the relationship between the two classes.
-
Static Nested Classes: C++ supports static nested classes, which do not require an instance of the enclosing class to be instantiated. Static nested classes can only access static members of the enclosing class.
Here’s an example showcasing a static nested class:
#include <iostream>
using namespace std;
class Outer {
public:
static class Inner {
public:
void show() {
cout << "Inside Static Nested Class." << endl;
}
};
};
int main() {
Outer::Inner inner;
inner.show();
return 0;
}
Output:
Inside Static Nested Class.
In this example, the Inner
class is static. We can create an instance of Inner
without needing an instance of Outer
. This flexibility can be beneficial when you want to encapsulate functionality without tying it to a specific instance of the enclosing class.
Advantages of Using Nested Classes
Using nested classes in C++ can provide several advantages that can enhance your programming experience. Here are some key benefits:
-
Improved Organization: By nesting classes, you can group related functionalities together. This organization makes your code more readable and easier to navigate.
-
Encapsulation: Nested classes can access the private and protected members of their enclosing class, which can lead to better encapsulation. This feature allows for a more cohesive design where the nested class acts as a helper or utility for the enclosing class.
-
Reduced Naming Conflicts: Since nested classes are scoped within their enclosing class, you reduce the likelihood of naming conflicts with other classes in your program. This scoping helps maintain clarity and prevents errors.
-
Logical Grouping: When you have classes that are closely related, nesting them logically groups them together. This grouping can help convey the relationship between classes more clearly to anyone reading the code.
Here’s an example that demonstrates the advantages of nested classes:
#include <iostream>
using namespace std;
class Library {
private:
string name;
public:
Library(string n) : name(n) {}
class Book {
private:
string title;
public:
Book(string t) : title(t) {}
void display() {
cout << "Book Title: " << title << endl;
}
};
void showLibrary() {
cout << "Library Name: " << name << endl;
}
};
int main() {
Library library("City Library");
library.showLibrary();
Library::Book book("C++ Programming");
book.display();
return 0;
}
Output:
Library Name: City Library
Book Title: C++ Programming
In this example, the Library
class contains a nested class Book
. This structure clearly indicates that a Book
is part of the Library
, enhancing code organization and readability. The nested class can access the enclosing class’s properties and methods, showcasing the benefits of encapsulation.
Conclusion
Nested classes in C++ provide a robust way to organize and encapsulate related functionalities. By understanding their characteristics and advantages, you can create cleaner, more maintainable code. Whether you are working on a small project or a large application, leveraging nested classes can significantly enhance your programming style. As you continue to explore C++, consider how nested classes can fit into your coding practices and improve your overall design.
FAQ
-
What are nested classes in C++?
Nested classes are classes defined within the scope of another class, allowing for better organization and encapsulation of related functionalities. -
Can nested classes access private members of the enclosing class?
Yes, nested classes can access the private and protected members of their enclosing class, enhancing encapsulation. -
What is a static nested class?
A static nested class does not require an instance of the enclosing class to be instantiated and can only access static members of the enclosing class. -
Why should I use nested classes?
Using nested classes improves code organization, reduces naming conflicts, and allows for better encapsulation of related functionalities. -
How do I create an instance of a nested class?
To create an instance of a nested class, you can either instantiate it using the scope resolution operator or create it through an instance of the enclosing class.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook