How to Insert New Elements in an STL Map in C++
-
Use the
insert
Member Function to Add a New Element tostd::map
in C++ -
Use the
insert_or_assign
Member Function to Add a New Element tostd::map
in C++ -
Use the
emplace
Member Function to Add a New Element tostd::map
in C++ -
Use the
emplace_hint
Member Function to Add a New Element tostd::map
in C++
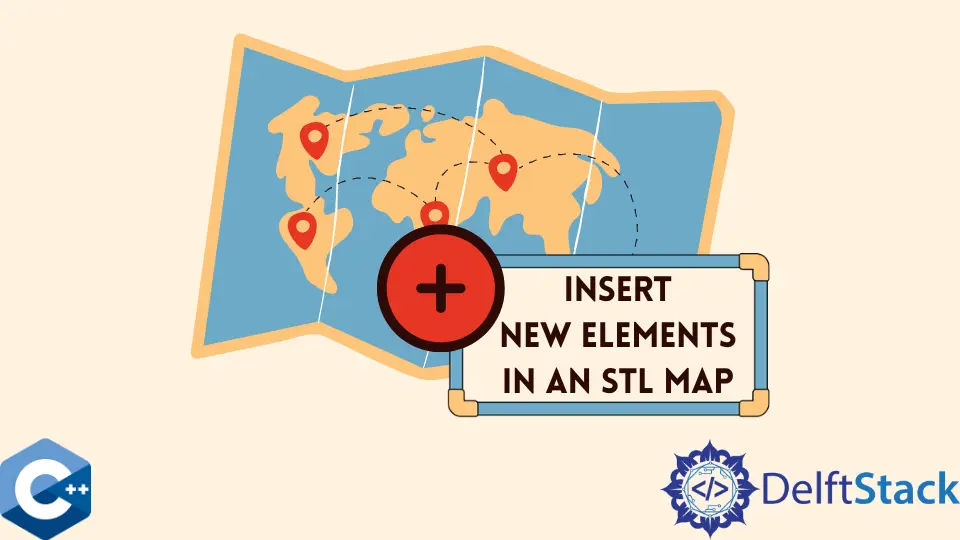
This article will explain multiple methods of how to add new elements in the std::map
object in C++.
Use the insert
Member Function to Add a New Element to std::map
in C++
The STL map
container provides a data structure for storing key-value pairs as elements, sorted automatically upon the insertion or initialization. New elements can be added to an std::map
object using several methods, the most common of which is the insert
function.
The insert
member function has multiple overloads. Still, the simplest one takes only a single argument specifying the const
reference to the inserted object. Another overload can accept an r-value reference and use the std::forward
command to construct an element in-place, as shown in the following sample code.
The latter overload returns a pair of the iterator to the inserted element and the bool
value, indicating successful insertion. We also demonstrate an overload of the insert
function that takes a list of key-values similar to the initializer list and constructs elements from the values. However, this overload does not have a return type.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.insert({"k", "ktop"});
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << "\n";
m1.insert({{"k", "ktop"}, {"p", "ptop"}});
printMap(m1);
return EXIT_SUCCESS;
}
Output:
Not Inserted
h : htop;
k : ktop;
p : ptop;
Use the insert_or_assign
Member Function to Add a New Element to std::map
in C++
The newer C++17 standard provides a member function called the insert_or_assign
, which inserts a new element in a map if the given key does not already exist. Otherwise, the function will assign the new value to the element with the existing key.
The function has several overloads, but the one called in the following example takes two arguments as r-value references to a key and a value. This overload also returns a pair of iterator and a bool
value. Notice that we check the status of the insertion with a conditional operator and print the corresponding messages.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.insert_or_assign("p", "ptop");
ret.second ? cout << "Inserted" : cout << "Assigned";
cout << "\n";
ret = m1.insert_or_assign("t", "ttop");
ret.second ? cout << "Inserted" : cout << "Assigned";
cout << "\n";
printMap(m1);
return EXIT_SUCCESS;
}
Output:
Inserted
Inserted
h : htop;
k : ktop;
p : ptop;
t : ttop;
Use the emplace
Member Function to Add a New Element to std::map
in C++
Another member function provided with the std::map
container is emplace
. This function provides efficiency in contrast to the insert
function as it does not require copying the argument values. On the contrary, the emplace
function will construct the element in-place, which means that the given parameters are forwarded to the class constructor. Note that depending on the arguments, the corresponding constructor will be called.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.emplace("t", "ttop");
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << endl;
ret = m1.emplace(std::make_pair("t", "ttop"));
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << endl;
printMap(m1);
return EXIT_SUCCESS;
}
Output:
Inserted
Not Inserted
h : htop;
k : ktop;
t : ttop;
Use the emplace_hint
Member Function to Add a New Element to std::map
in C++
Alternatively, one can utilize the emplace_hint
member function to insert a new element into a map with an additional parameter called a hint
, which specifies a position where the operation should start searching. It would return an iterator to the newly inserted element if the process were successful. Otherwise, the iterator to the existing element with the same key is returned.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.emplace_hint(m1.begin(), "t", "ttop");
m1.emplace_hint(ret, "m", "mtop");
m1.emplace_hint(m1.begin(), "p", "ptop");
printMap(m1);
return EXIT_SUCCESS;
}
Output:
h : htop;
k : ktop;
m : mtop;
p : ptop;
t : ttop;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook