How to Sort Map by Value in C++
-
Use
std::vector
andstd::sort
Algorithm to Sort Map Elements by Value in C++ -
Use
std::map
andstd::map::emplace
to Sort Map Elements by Value in C++
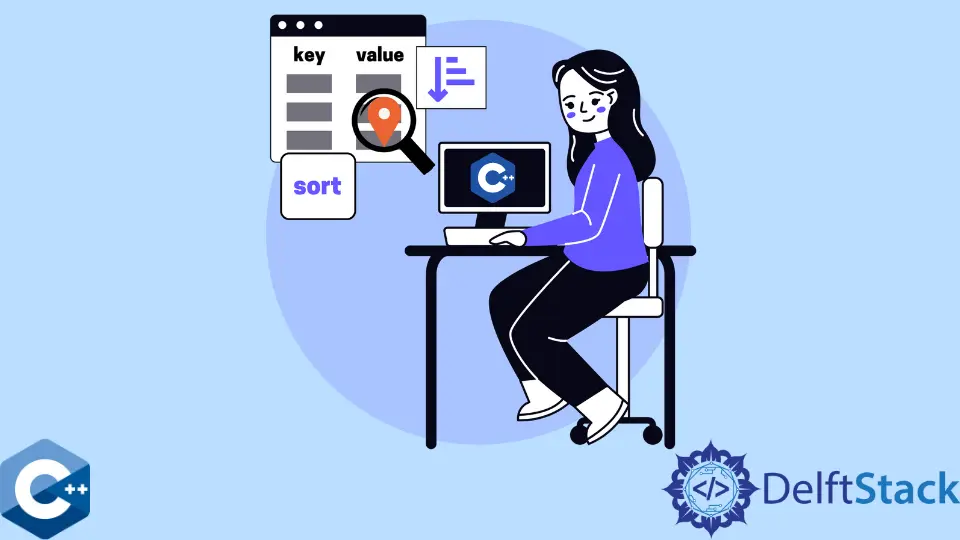
This article will demonstrate multiple methods about how to sort the map by values in C++.
Use std::vector
and std::sort
Algorithm to Sort Map Elements by Value in C++
std::map
is an associative container that can store key-value pairs with unique keys, and the latter are used to sort the elements in the object automatically. In this case, we are declaring a sample std::map
object with integer strings as keys and regular strings as values. The problem is to sort these elements by the values of the strings.
We can’t directly use the std::sort
algorithm on the std::map
structure, so we have to initialize another object that can be sorted. Thus, the declaration of the std::vector
contains the pairs of the same type. We construct vector
elements using the for
loop and emplace_back
method. Once the loop is executed, the vector
is ready to be passed to the std::sort
algorithm. Notice that we specify the lambda expression to define the element comparison function, and it only compares the second members. Finally, we can output the std::vector
elements as a sorted map representation.
#include <iostream>
#include <map>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
using std::vector;
int main() {
map<string, string> veggy_map = {{
"1",
"Yam",
},
{
"2",
"Pumpkin",
},
{
"3",
"Ginger",
},
{
"4",
"Melon",
},
{
"5",
"Beetroot",
},
{
"6",
"Spinach",
}};
cout << "Unsorted - " << endl;
for (const auto &[key, value] : veggy_map) {
cout << key << " : " << value << endl;
}
vector<std::pair<string, string> > arr;
for (const auto &item : veggy_map) {
arr.emplace_back(item);
}
std::sort(arr.begin(), arr.end(),
[](const auto &x, const auto &y) { return x.second < y.second; });
cout << "Sorted - " << endl;
for (const auto &[key, value] : arr) {
cout << key << " : " << value << endl;
}
return EXIT_SUCCESS;
}
Output:
Sorted -
5 : Beetroot
3 : Ginger
4 : Melon
2 : Pumpkin
6 : Spinach
1 : Yam
Use std::map
and std::map::emplace
to Sort Map Elements by Value in C++
The previous solution did not deal with the std::map
object itself and used an external structure for sorting. In this case, we implement a solution to store the value-sorted elements in another std::map
object. This can be achieved using the built-in map function - emplace
. Namely, we declare another map
object and construct its elements using the emplace
method, but we also pass the reversed key-value pairs. As a result, the map
container automatically sorts elements by keys: the values in the previous map
object. Next, we can use the sorted map
object for other operations that may be needed in the following code blocks without worrying that it’s stored in a different object.
#include <iostream>
#include <map>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
using std::vector;
int main() {
map<string, string> veggy_map = {{
"1",
"Yam",
},
{
"2",
"Pumpkin",
},
{
"3",
"Ginger",
},
{
"4",
"Melon",
},
{
"5",
"Beetroot",
},
{
"6",
"Spinach",
}};
cout << "Unsorted - " << endl;
for (const auto& [key, value] : veggy_map) {
cout << key << " : " << value << endl;
}
cout << "Sorted - " << endl;
map<string, string> veggy_map2;
for (const auto& [key, value] : veggy_map) {
veggy_map2.emplace(value, key);
}
for (const auto& [key, value] : veggy_map2) {
cout << value << " : " << key << endl;
}
return EXIT_SUCCESS;
}
Output:
Sorted -
5 : Beetroot
3 : Ginger
4 : Melon
2 : Pumpkin
6 : Spinach
1 : Yam
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook