How to Use the STL Unordered Map Container in C++
-
Use the
std::unordered_map
Element to Declare an Unordered Map Container in C++ -
Use the
contains
Member Function to Check if the Given Element Exists in a Map in C++ -
Use the
erase
Member Function to Remove the Specific Element From a Map in C++ -
Use the
insert
Member Function to Add New Elements to a Map in C++
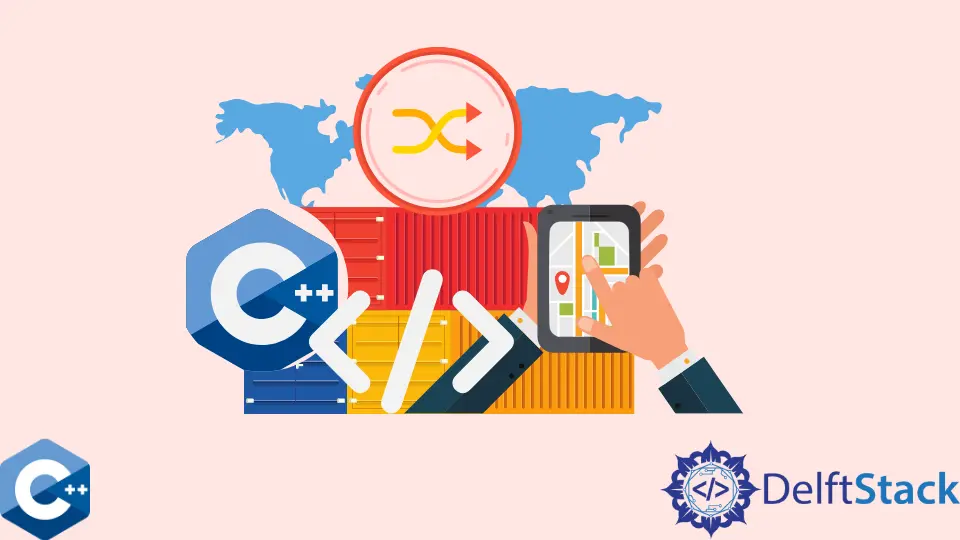
This article explains several methods of how to use the STL unordered_map
container in C++.
Use the std::unordered_map
Element to Declare an Unordered Map Container in C++
The std::unordered_map
element implements an associative container of key-value pairs where each key is unique. In contrast to the std::map
, the std::unordered_map
container does not store the elements in sorted order. Thus, the container is mostly utilized for element lookup, and position does not matter. Moreover, the position of elements over the lifetime of the object is not guaranteed to be fixed. So the programmer should treat the order as undefined.
The following example demonstrates the list initialization of the unordered_map
container and then outputs the contents of both maps. Note that the descending order of the m1
elements does not imply they are sorted.
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
std::map<int, string> m2 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
printMap(m1);
printMap(m2);
return EXIT_SUCCESS;
}
Output:
4 : orange;
3 : grape;
2 : banana;
1 : apple;
1 : apple;
2 : banana;
3 : grape;
4 : orange;
Use the contains
Member Function to Check if the Given Element Exists in a Map in C++
The contains
member function has been part of the std::unordered_map
container since the C++20 update. You can use the function to check if the given element exists in the map. This command accepts the key-value as the only argument and returns true
if the key is found. The function has the constant average running time, with the worst case being the linear depending on the size of the container itself.
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
for (int x : {1, 2, 3, 4, 5}) {
if (m1.contains(x)) {
cout << x << ": Found\n";
} else {
cout << x << ": Not found\n";
}
}
return EXIT_SUCCESS;
}
Output:
1: Found
2: Found
3: Found
4: Found
5: Not found
Use the erase
Member Function to Remove the Specific Element From a Map in C++
You can utilize the erase
member function to remove the given key-value pair from the map. The function has three overloads, the first of which takes the iterator to the map element, which needs to be removed from the container. The second function overload takes two iterators to specify the range, which will be removed from the map. Be aware that the given range must be a valid one within the calling container object. The third overload can take the key value of the element that should be removed.
In the following example, we remove each element that has an even number as the key value.
#include <iostream>
#include <map>
#include <unordered_map>
using std::cout;
using std::endl;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
for (auto it = m1.begin(); it != m1.end();) {
if (it->first % 2 == 0)
it = m1.erase(it);
else
++it;
}
printMap(m1);
return EXIT_SUCCESS;
}
Output:
3 : grape;
1 : apple;
Use the insert
Member Function to Add New Elements to a Map in C++
Another core member function of the unordered_map
container is the insert
function, which you can use to add new elements to the map. The function has multiple overloads, but we utilize the first one that just takes a key-value pair. Note that we also use the std::make_pair
function to construct a new pair with the given argument values.
#include <iostream>
#include <map>
#include <unordered_map>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::unordered_map<int, string> m1 = {
{1, "apple"},
{2, "banana"},
{3, "grape"},
{4, "orange"},
};
vector<string> vec{"papaya", "olive", "melon"};
auto count = 0;
for (const auto& item : vec) {
m1.insert(std::make_pair(count, item));
}
printMap(m1);
return EXIT_SUCCESS;
}
Output:
0 : papaya;
4 : orange;
3 : grape;
2 : banana;
1 : apple;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook