Inserisci nuovi elementi in una mappa STL in C++
-
Usa la funzione membro
insert
per aggiungere un nuovo elemento astd::map
in C++ -
Usa la funzione membro
insert_or_assign
per aggiungere un nuovo elemento astd::map
in C++ -
Usa la funzione membro
emplace
per aggiungere un nuovo elemento astd::map
in C++ -
Usa la funzione membro
emplace_hint
per aggiungere un nuovo elemento astd::map
in C++
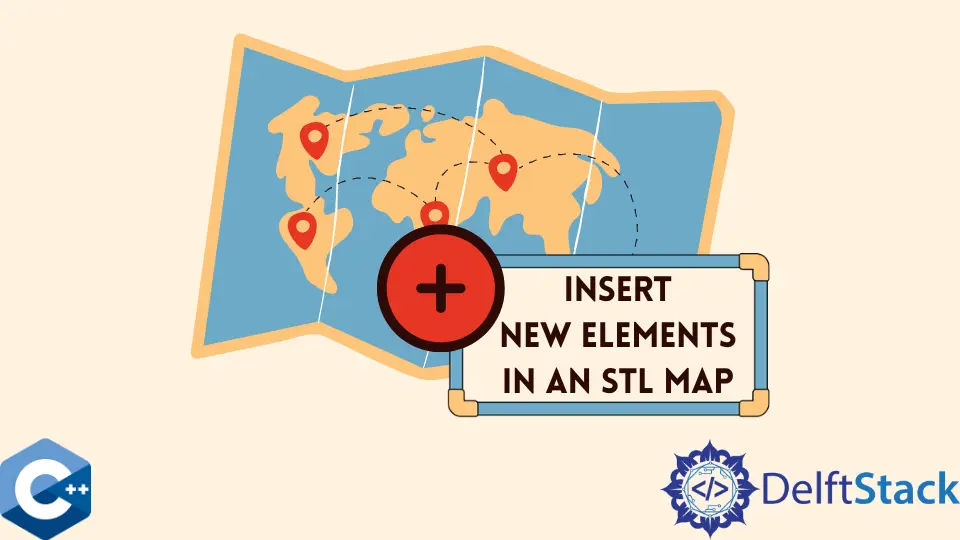
Questo articolo spiegherà più metodi su come aggiungere nuovi elementi nell’oggetto std::map
in C++.
Usa la funzione membro insert
per aggiungere un nuovo elemento a std::map
in C++
Il contenitore STL map
fornisce una struttura dati per la memorizzazione di coppie chiave-valore come elementi, ordinati automaticamente al momento dell’inserimento o dell’inizializzazione. Nuovi elementi possono essere aggiunti a un oggetto std::map
utilizzando diversi metodi, il più comune dei quali è la funzione insert
.
La funzione membro insert
ha più overload. Tuttavia, il più semplice prende solo un singolo argomento che specifica il riferimento const
all’oggetto inserito. Un altro overload può accettare un riferimento di valore r e usare il comando std::forward
per costruire un elemento sul posto, come illustrato nel codice di esempio seguente.
Quest’ultimo overload restituisce una coppia dell’iteratore all’elemento inserito e il valore bool
, indicando l’avvenuto inserimento. Dimostriamo anche un overload della funzione insert
che prende un elenco di valori-chiave simile all’elenco dell’inizializzatore e costruisce elementi dai valori. Tuttavia, questo overload non ha un tipo restituito.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.insert({"k", "ktop"});
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << "\n";
m1.insert({{"k", "ktop"}, {"p", "ptop"}});
printMap(m1);
return EXIT_SUCCESS;
}
Produzione:
Not Inserted h : htop;
k : ktop;
p : ptop;
Usa la funzione membro insert_or_assign
per aggiungere un nuovo elemento a std::map
in C++
Il nuovo standard C++17 fornisce una funzione membro chiamata insert_or_assign
, che inserisce un nuovo elemento in una mappa se la chiave data non esiste già. In caso contrario, la funzione assegnerà il nuovo valore all’elemento con la chiave esistente.
La funzione ha diversi overload, ma quello chiamato nell’esempio seguente accetta due argomenti come riferimenti di valore r a una chiave ea un valore. Questo overload restituisce anche una coppia di iteratori e un valore bool
. Si noti che controlliamo lo stato dell’inserimento con un operatore condizionale e stampiamo i messaggi corrispondenti.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.insert_or_assign("p", "ptop");
ret.second ? cout << "Inserted" : cout << "Assigned";
cout << "\n";
ret = m1.insert_or_assign("t", "ttop");
ret.second ? cout << "Inserted" : cout << "Assigned";
cout << "\n";
printMap(m1);
return EXIT_SUCCESS;
}
Produzione:
Inserted Inserted h : htop;
k : ktop;
p : ptop;
t : ttop;
Usa la funzione membro emplace
per aggiungere un nuovo elemento a std::map
in C++
Un’altra funzione membro fornita con il contenitore std::map
è emplace
. Questa funzione fornisce efficienza in contrasto con la funzione insert
in quanto non richiede la copia dei valori degli argomenti. Al contrario, la funzione emplace
costruirà l’elemento in-place, il che significa che i parametri dati vengono inoltrati al costruttore della classe. Si noti che, a seconda degli argomenti, verrà chiamato il costruttore corrispondente.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.emplace("t", "ttop");
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << endl;
ret = m1.emplace(std::make_pair("t", "ttop"));
ret.second ? cout << "Inserted" : cout << "Not Inserted";
cout << endl;
printMap(m1);
return EXIT_SUCCESS;
}
Produzione:
Inserted Not Inserted h : htop;
k : ktop;
t : ttop;
Usa la funzione membro emplace_hint
per aggiungere un nuovo elemento a std::map
in C++
In alternativa, si può utilizzare la funzione membro emplace_hint
per inserire un nuovo elemento in una mappa con un parametro aggiuntivo chiamato hint
, che specifica una posizione in cui l’operazione dovrebbe iniziare la ricerca. Restituirebbe un iteratore all’elemento appena inserito se il processo avesse esito positivo. In caso contrario, viene restituito l’iteratore dell’elemento esistente con la stessa chiave.
#include <iostream>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
template <typename Map>
void printMap(Map& m) {
for (auto& p : m) cout << p.first << " : " << p.second << ";" << endl;
cout << endl;
}
int main() {
std::map<string, string> m1 = {
{"h", "htop"},
{"k", "ktop"},
};
auto ret = m1.emplace_hint(m1.begin(), "t", "ttop");
m1.emplace_hint(ret, "m", "mtop");
m1.emplace_hint(m1.begin(), "p", "ptop");
printMap(m1);
return EXIT_SUCCESS;
}
Produzione:
h : htop;
k : ktop;
m : mtop;
p : ptop;
t : ttop;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook