How to Check if a File Exists in C++
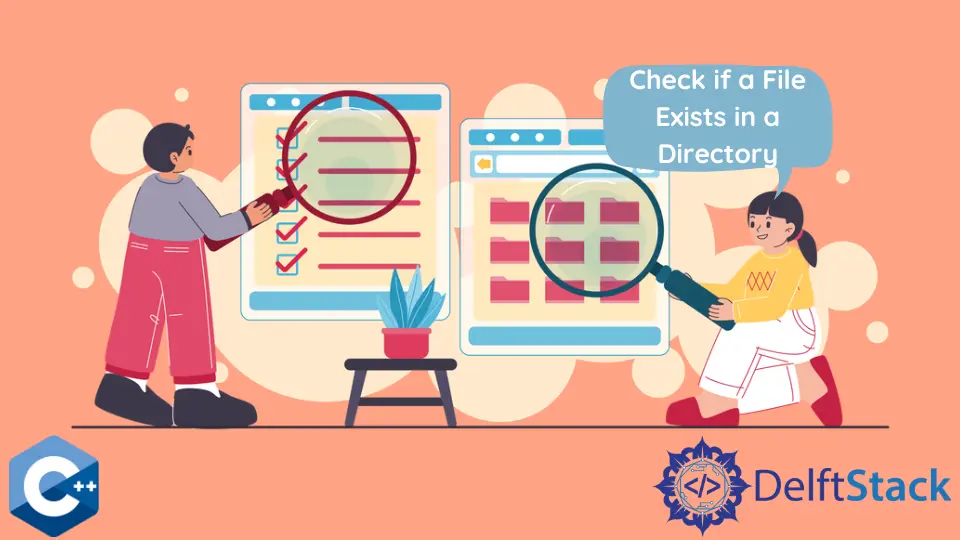
This article will introduce C++ methods to check if a certain file exists in a directory. Note, though, the following tutorial is based on C++ 17 filesystem
library, which is only supported in new compilers.
Use std::filesystem::exists
to Check if a File Exists in a Directory
The exists
method takes a path as an argument and returns boolean value true
if it corresponds to an existing file or directory. In the following example, we initialize a vector with arbitrary filenames to check them in the filesystem with exists
functions. Be aware that the exists
method only checks the current directory where the executable file is located.
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
for (const auto &file : files_to_check) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
}
return EXIT_SUCCESS;
}
Above code can be reimplemented with for_Each
STL algorithm, which will provide better code reusability:
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
auto check = [](const auto &file) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
};
for_each(files_to_check.begin(), files_to_check.end(), check);
return EXIT_SUCCESS;
}
The exists
method can be more informative if combined with other <filesystem>
library routines like: is_directory
and is_regular_file
. Generally, some filesystem methods don’t distinguish between files and directories, but we can use specific file type checking functions to verify path names, as shown in the following sample code:
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::exists;
using std::filesystem::is_directory;
using std::filesystem::is_regular_file;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
for (const auto &file : files_to_check) {
if (exists(file)) {
if (is_directory(file)) cout << "Directory exists\n";
if (is_regular_file(file))
cout << "File exists\n";
else
cout << "Exists\n";
} else {
cout << "Doesn't exist\n";
};
};
return EXIT_SUCCESS;
}
Now let’s consider a case when we want to navigate to a particular directory and check for a specific filename if it exists. To do this, we need to use the current_path
method, which returns the current directory if no argument is passed, or it can change the current working directory to to the given path specified path if argument is given. Don’t forget to change directory path and file names based on your system to verify program output better:
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
using std::filesystem::current_path;
using std::filesystem::exists;
using std::filesystem::is_directory;
using std::filesystem::is_regular_file;
int main() {
vector<string> files_to_check = {"main.cpp", "Makefile", "hello-world"};
current_path("../");
for (const auto &file : files_to_check) {
exists(file) ? cout << "Exists\n" : cout << "Doesn't exist\n";
}
return EXIT_SUCCESS;
}
We can also check what permissions the current user has on files in a directory while iteration through directory_iterator
. The status
method is used to get permissions and store them in a special class called perms
. Retrieved permissions can be displayed with bitwise operations on perms
structure. The following example shows the extraction of owner permissions only (see the full list here).
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
using std::filesystem::directory_iterator;
using std::filesystem::exists;
using std::filesystem::perms;
using std::string;
int main() {
vector<std::filesystem::perms> f_perm;
string path = "../";
for (const auto& file : directory_iterator(path)) {
cout << file << " - ";
cout << ((file.status().permissions() & perms::owner_read) != perms::none
? "r"
: "-")
<< ((file.status().permissions() & perms::owner_write) != perms::none
? "w"
: "-")
<< ((file.status().permissions() & perms::owner_exec) != perms::none
? "x"
: "-")
<< endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook