How to Append Text to a File in C++
-
Append Text to a File in C++ Using
ofstream
With thestd::ios::app
Flag -
Append Text to a File in C++ Using
fstream
With thestd::ios::app
Flag -
Append Text to a File in C++ Using
std::ofstream
Without Flags -
Append Text to a File in C++ Using
std::fstream
and thewrite()
Method - Conclusion
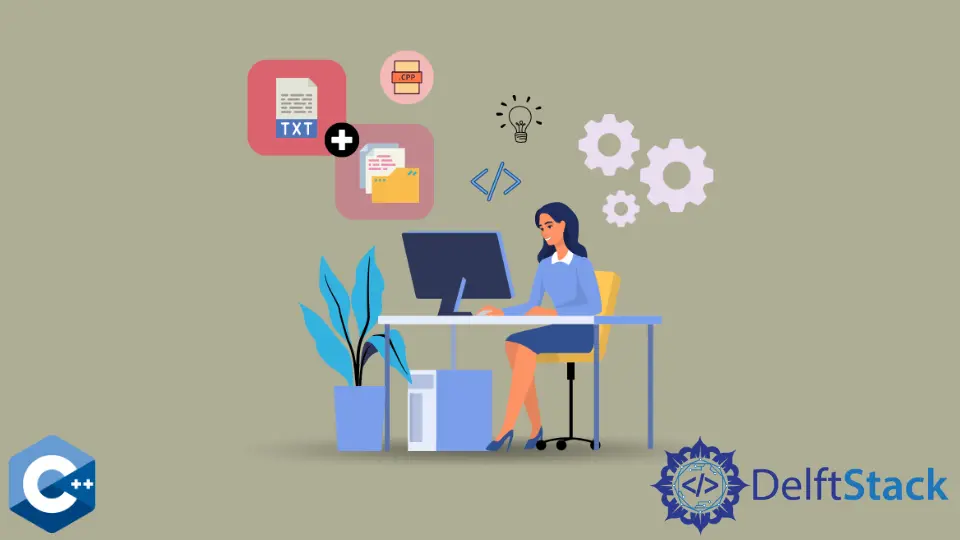
Working with files is a common task in programming, and C++ provides powerful tools for file manipulation. One common operation is appending text to an existing file.
This article explores multiple methods to accomplish this, offering code examples and explanations to help you understand the process thoroughly.
Append Text to a File in C++ Using ofstream
With the std::ios::app
Flag
In C++, the std::ofstream
class is part of the Standard Template Library (STL) and is used for writing to files. It’s an excellent choice when you want to create, open, write, or append data to text files.
Moreover, the std::ios::app
flag is an important component of std::ofstream
. When used, it instructs the stream to open the file in append mode, which means any data you write to the stream will be added to the end of the existing file content.
If the file doesn’t exist, it will be created.
Now, to append text to a file in C++ using std::ofstream
with the std::ios::app
flag, you can follow these steps:
-
Include the necessary header files:
#include <fstream> #include <iostream>
-
Open the file in append mode (
std::ios::app
) using anstd::ofstream
object. -
Write the text you want to append to the file using the
<<
operator. -
Close the file when you’re done.
Here’s an example:
#include <fstream>
#include <iostream>
int main() {
// File path
std::string filePath = "example.txt";
// Open the file in append mode
std::ofstream outputFile(filePath, std::ios::app);
if (!outputFile) {
std::cerr << "Failed to open the file for appending." << std::endl;
return 1;
}
// Text to append to the file
std::string textToAppend = "This is some appended text.";
// Append the text to the file
outputFile << textToAppend << std::endl;
// Close the file
outputFile.close();
std::cout << "Text appended to the file successfully." << std::endl;
return 0;
}
In this example, we first opened the file example.txt
in append mode using std::ios::app
. If the file doesn’t exist, it will be created.
We then appended the specified text to the file using the <<
operator, and finally, we closed the file.
Make sure to check for errors when opening the file, as shown in the example, to handle cases where the file cannot be opened.
Append Text to a File in C++ Using fstream
With the std::ios::app
Flag
Similar to the ofstream
approach, you can use std::fstream
with the std::ios::app
flag to open a file for both reading and appending.
In C++, the std::fstream
class is part of the Standard Template Library (STL) and is used for file input and output. It is also an excellent choice when you need to create, open, write, or append data to text files.
The std::ios::app
flag is a vital component of std::fstream
. When applied, it directs the stream to open the file in append mode.
In this mode, any data written to the stream will be added to the end of the existing file content. If the file doesn’t exist, it will be created.
To append text to a file in C++ using std::fstream
with the std::ios::app
flag, you can follow these steps:
-
Include the necessary headers:
#include <fstream> #include <iostream> #include <string>
-
Create a
std::fstream
object and open the file in append mode:std::fstream file("example.txt", std::ios::app);
-
Check if the file is opened successfully using
if (!file.is_open())
. -
Append text to the file using
file << textToAppend << std::endl;
, replacingtextToAppend
with the desired text. -
Close the file when done with
file.close();
.
Here’s the complete example:
#include <fstream>
#include <iostream>
#include <string>
int main() {
// Open the file in append mode
std::fstream file("example.txt", std::ios::app);
// Check if the file is opened successfully
if (!file.is_open()) {
std::cerr << "Error opening the file!" << std::endl;
return 1; // Exit with an error code
}
// Text to append
std::string textToAppend = "This is the text to append.";
// Append text to the file
file << textToAppend << std::endl;
// Close the file
file.close();
return 0;
}
This code will open the file in append mode, append the specified text, and then close the file.
If the file does not exist, it will be created. If it exists, new content will be added to the end of the file without overwriting the existing content.
Append Text to a File in C++ Using std::ofstream
Without Flags
Now, if you want to create a new file or overwrite the existing content, you can use std::ofstream
without any flags. If the file already exists, this will effectively clear its content and start with a blank slate.
Here’s a basic example:
#include <fstream>
#include <iostream>
int main() {
// Creating a new file or overwriting existing content
std::ofstream outputFile("example.txt");
// Checking if the file is successfully opened
if (outputFile.is_open()) {
outputFile << "This will overwrite existing content.\n";
outputFile.close(); // Always close the file when done
} else {
std::cerr << "Unable to open the file.\n";
}
return 0;
}
In this code snippet, we first created a std::ofstream
object named outputFile
and specified the file we want to work with, in this case, example.txt
.
Append Text to a File in C++ Using std::fstream
and the write()
Method
Another method to solve this problem is to call the write
member function explicitly from the fstream
object rather than use the <<
operator as we’ve done in the previous examples. This method should be faster for most scenarios, but always profile your code and compare timings if you have strict performance requirements.
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::string;
int main() {
string text("Some huge text to be appended");
string filename("tmp.txt");
fstream file;
file.open(filename, std::ios_base::app);
if (file.is_open()) file.write(text.data(), text.size());
cout << "Done!" << endl;
return EXIT_SUCCESS;
}
In the code above, we opened the file in append mode (std::ios_base::app
) using the open()
method of the fstream
object. We have also checked if the file was successfully opened using file.is_open()
.
Then, we used the write()
method to write the text as binary data to the file. As we can see, this method takes two arguments: a pointer to the data and the number of bytes to write.
Finally, we printed Done!
to indicate that the operation was complete.
Conclusion
Each method presented in this article offers a unique approach to appending text to a file. The choice of method depends on your specific requirements and coding style.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook