Garbage Collection in C++
- Understanding Memory Management in C++
- Manual Memory Management in C++
- Common Pitfalls in Memory Management
- Best Practices for Memory Management in C++
- Conclusion
- FAQ
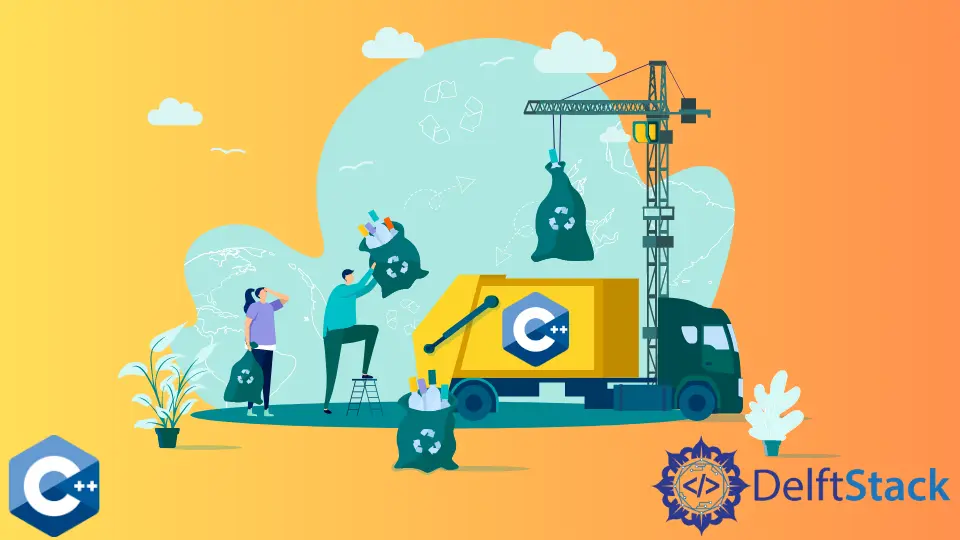
Garbage collection is a crucial concept in programming, especially when it comes to memory management. In languages like C++, developers must manage memory manually, which can be both empowering and daunting. Unlike languages that feature automatic garbage collection, C++ requires programmers to allocate and deallocate memory using commands like new
and delete
.
This article will delve into the intricacies of garbage collection in C++, discussing its significance, challenges, and best practices for effective memory management. Whether you’re a novice or an experienced developer, understanding garbage collection in C++ is essential for writing efficient and robust applications.
Understanding Memory Management in C++
Memory management in C++ revolves around two primary concepts: stack and heap memory. The stack is where local variables are stored, and its size is limited. It automatically manages memory allocation and deallocation, ensuring that once a function exits, its local variables are removed. However, the heap is where dynamic memory allocation occurs. Here, memory is allocated at runtime, allowing for greater flexibility but also requiring careful management.
When you use the new
operator, memory is allocated on the heap. This memory remains allocated until you explicitly free it using the delete
operator. Failing to do so can lead to memory leaks, where memory that is no longer needed remains allocated, leading to inefficient use of resources. In contrast, if you delete memory that is still in use, it can lead to undefined behavior and program crashes.
Understanding the balance between stack and heap memory is essential for effective garbage collection in C++. Properly managing memory can enhance application performance, prevent memory leaks, and improve overall stability.
Manual Memory Management in C++
In C++, memory management is predominantly manual. This means that programmers have to take responsibility for allocating and deallocating memory. Using new
and delete
correctly is vital to ensuring that your application runs smoothly.
Here’s a basic example:
#include <iostream>
int main() {
int* ptr = new int;
*ptr = 42;
std::cout << "Value: " << *ptr << std::endl;
delete ptr;
return 0;
}
Output:
Value: 42
In this example, we allocate memory for an integer using new
and then assign it a value. After using the allocated memory, we must call delete
to free it. If we forget to call delete
, the memory remains allocated, leading to a memory leak. This manual approach requires vigilance and discipline from the programmer, as overlooking these details can have significant consequences.
Common Pitfalls in Memory Management
While manual memory management provides flexibility, it also introduces several pitfalls. One common issue is memory leaks, which occur when allocated memory is never released. This can happen if a programmer forgets to call delete
or if the program exits unexpectedly without cleaning up.
Another pitfall is dangling pointers. These occur when a pointer still references memory that has been deallocated. Accessing such memory can lead to undefined behavior, crashes, or data corruption.
Here’s an example of a dangling pointer:
#include <iostream>
int main() {
int* ptr = new int(10);
delete ptr;
std::cout << *ptr << std::endl;
return 0;
}
Output:
Segmentation fault
In this example, after calling delete
, ptr
becomes a dangling pointer. Attempting to access it results in a segmentation fault. To prevent such issues, it’s crucial to nullify pointers after deletion.
Best Practices for Memory Management in C++
To avoid common pitfalls associated with manual memory management in C++, there are several best practices you can follow:
-
Always pair new with delete: Whenever you allocate memory using
new
, ensure that you release it withdelete
. This helps prevent memory leaks. -
Use smart pointers: C++11 introduced smart pointers, like
std::unique_ptr
andstd::shared_ptr
, which automatically manage memory. They help prevent memory leaks and dangling pointers by automatically deleting the memory when it is no longer needed. -
Initialize pointers: Always initialize pointers to
nullptr
when you declare them. This practice helps avoid dangling pointers. -
Use RAII (Resource Acquisition Is Initialization): This principle ties resource management to object lifetime. When an object goes out of scope, its destructor is called, releasing any resources it holds.
Here’s an example using a smart pointer:
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr = std::make_unique<int>(42);
std::cout << "Value: " << *ptr << std::endl;
return 0;
}
Output:
Value: 42
In this example, std::unique_ptr
automatically manages the memory. When ptr
goes out of scope, the memory is freed automatically, eliminating the risk of memory leaks.
Conclusion
Garbage collection in C++ is a critical aspect of memory management that requires diligence and understanding. While the manual approach offers flexibility, it also demands careful attention to detail. By following best practices, such as using smart pointers and adhering to the RAII principle, developers can effectively manage memory and avoid common pitfalls. Understanding garbage collection is essential for anyone looking to write efficient and robust C++ applications.
FAQ
-
What is garbage collection in C++?
Garbage collection in C++ refers to the manual process of managing memory allocation and deallocation, requiring programmers to use commands likenew
anddelete
. -
Why is manual memory management important in C++?
Manual memory management is important in C++ because it allows developers to have fine-grained control over memory usage, which can lead to optimized performance and resource management. -
What are smart pointers in C++?
Smart pointers are objects that manage the lifetime of dynamically allocated memory, automatically deallocating it when it is no longer needed, thus preventing memory leaks. -
How can I prevent memory leaks in C++?
To prevent memory leaks, always pairnew
withdelete
, consider using smart pointers, and ensure that all allocated memory is released before the program exits.
- What are the risks of using dangling pointers?
Dangling pointers can lead to undefined behavior, crashes, or data corruption when accessed after the memory they reference has been deallocated.