How to Allocate and Deallocate the Memory in C++
-
Allocate and Deallocate the Memory Using the
new
anddelete
Operators - Allocate and Deallocate the Memory Using C Standard Library
- Benefits of Dynamic Memory Allocation Using the Standard Library
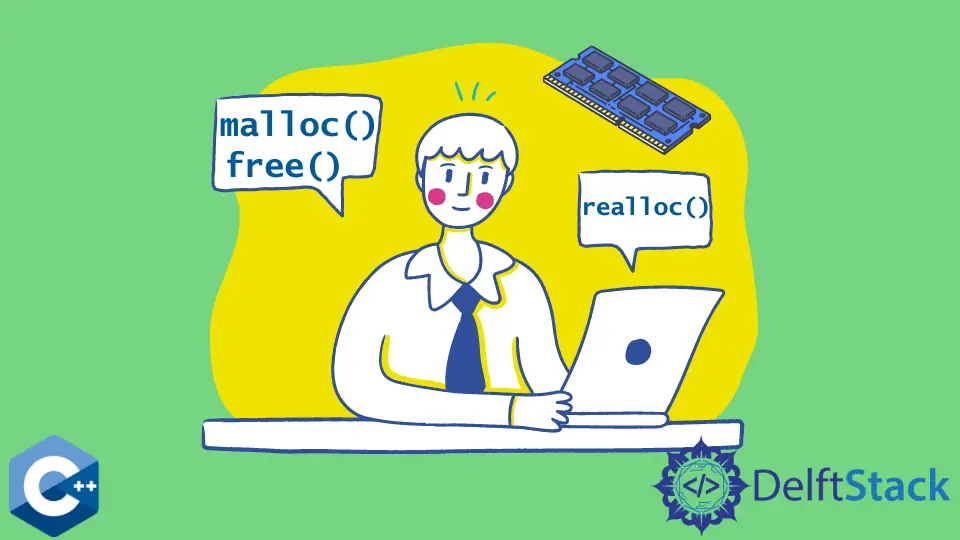
The compiler automatically controls the memory allotted to variables in other programming languages like Java and Python. But in C++, this is not the case.
Since its creation, memory allocation and deallocation have been integral to the C++ language. It is responsible for managing dynamic memory in the program, which includes allocating memory to be used during program execution, releasing allocated memory when it is no longer needed, and determining what happens to allocated memory when the program ends.
Memory allocation and deallocation are managed by the operating system’s virtual memory manager, which uses a Paging technique.
The C++ programming language provides several functions to allocate and free up memory. These functions include malloc
, calloc
, realloc
, free
, new
, and delete
.
Let’s start with the new
and delete
operators.
Allocate and Deallocate the Memory Using the new
and delete
Operators
the new
Operator
The new
operator allocates the memory for an object of a specified type. First, it creates a new object in memory, which can then be initialized with values.
If no value is specified, the default constructor is used to initialize the object. The syntax of the new
operator is:
pointer - variable = new data - type
The pointer of type data type is used in this case. Any built-in data type, including arrays, or any user-defined data type, including structure and class, could be used as the data type.
What if there is insufficient memory available during program execution?
If there is insufficient memory in a heap to allocate, the new request fails by throwing an error message of type std::bad alloc
; however, if nothrow
is used with the new
operator, in which scenario it returns a NULL pointer. As a result, looking for the new
’s pointer variable before running its program is essential.
the delete
Operator
The delete
operator releases or deallocates memory allocated by the new
operator. The syntax of the delete
operator is as follows:
delete pArray;
Here, pArray
refers to an array allocated with the new
operator.
Typically, the delete
operator performs two functions:
- First, it destroys the object by invoking its destructor.
- It deallocates the memory.
Example Using the new
and delete
Operators:
#include <iostream>
using namespace std;
int main() {
int* x;
x = new int;
*x = 34;
cout << *x << endl;
delete x;
return 0;
}
In this example, firstly, we allocated the memory using the new
operator, then deallocated it using the delete
operator.
Click here to check the working of the code as mentioned above.
Allocate and Deallocate the Memory Using C Standard Library
Using malloc()
, free()
, and realloc()
, the C standard library makes it easier to allocate and deallocate memory. These functions are available in the C++ language.
the malloc()
Function
malloc()
is a function in the C++ programming language that allocates a contiguous memory space of the specified size. It is used to allocate dynamic memory for variables, arrays, and other data structures.
The malloc()
function takes three arguments:
- The first argument specifies the number of bytes to allocate.
- The second argument specifies the type of data to allocate.
- The third argument is an address where to store the allocated data.
the free()
Function
The free()
function in C++ releases or deallocates a region of memory that was previously allocated using the malloc()
operator. It returns this memory region to the system so it can be reused later.
the realloc()
Function
realloc()
is a function that can change the size of a memory block. It is used to change the size of an allocation, typically to enlarge it when it has been exhausted.
realloc()
takes in three parameters: a pointer to the old allocation, information to the new allocation, and a new size for the new allocation.
The old pointer points to the original allocated memory block before reallocation, and the new information means to the newly allocated memory after reallocation. The new size specifies how much space should be allocated for this block by setting its length in bytes or the number of items if this parameter is not given.
Benefits of Dynamic Memory Allocation Using the Standard Library
The library provides several types of functions for managing dynamic memory, such as:
- Allocating and freeing blocks of memory on the heap;
- Copying data between blocks of allocated memory;
- Accessing the size of an allocated block of memory at run time;
- Checking whether a block of allocated memory is empty; and
- Freeing all blocks in a heap at once.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook