How to Use the delete Operator in C++
-
Use the
delete
Operator to Free the Allocated Resources for the Object -
Use the
delete
Operator to Free Elements in Vector
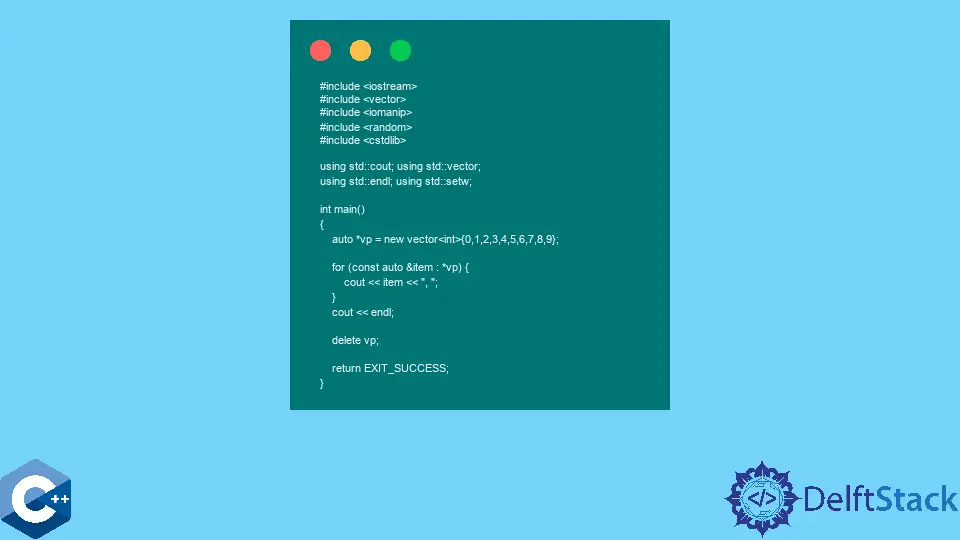
This article will explain several methods of how to use the delete
operator in C++.
Use the delete
Operator to Free the Allocated Resources for the Object
Dynamically allocated objects using the new
operator should be freed before the program exit, which means that the caller is responsible for explicitly invoking the delete
operation. Notice that constructor initializers can be utilized to declare and initialize objects with the new
operator. The following example code demonstrates such a scenario and then frees the corresponding resources before returning from the main
function. Mind that not calling the delete
operator on the heap objects will result in a memory leak, leading to massive memory usage for the long-running processes.
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
int main() {
int *num = new int(1024);
auto *sp = new string(10, 'a');
cout << *num << endl;
cout << *sp << endl;
for (const auto &item : *vp) {
cout << item << ", ";
}
cout << endl;
delete num;
delete sp;
return EXIT_SUCCESS;
}
Output:
1024
aaaaaaaaaa
new
and delete
operators can also be used with standard library containers. Namely, the std::vector
object is allocated and initialized in a single statement in the following example code. Once the new
operator returns a pointer to the vector
we can conduct operations on it as with the regular vector
object.
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
int main() {
auto *vp = new vector<int>{0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
for (const auto &item : *vp) {
cout << item << ", ";
}
cout << endl;
delete vp;
return EXIT_SUCCESS;
}
Output:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
Use the delete
Operator to Free Elements in Vector
Using the delete
operator with dynamically allocated arrays is slightly different. Generally, new
and delete
operate on objects one at a time, but the arrays need multiple elements to be allocated with one call. Thus, the square bracket notation is utilized to specify the number of elements in the array, as demonstrated in the following example code. Allocation like this returns a pointer to the first element, which can be dereferenced to access different members. Finally, when the array is not needed, it should be freed with a special delete
notation containing empty square brackets, which guarantees that every element has been deallocated.
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int SIZE = 10;
constexpr int MIN = 1;
constexpr int MAX = 1000;
void initPrintIntVector(int *arr, const int &size) {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int i = 0; i < size; ++i) {
arr[i] = distr(eng) % MAX;
cout << setw(3) << arr[i] << "; ";
}
cout << endl;
}
int main() {
int *arr1 = new int[SIZE];
initPrintIntVector(arr1, SIZE);
delete[] arr1;
return EXIT_SUCCESS;
}
Output:
311; 468; 678; 688; 468; 214; 487; 619; 464; 734;
266; 320; 571; 231; 195; 873; 645; 981; 261; 243;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook