How to Calculate Factorial of a Number in C++
- Understanding Factorial
- Use Iterative Method to Calculate Factorial of a Number in C++
- Use Recursive Method to Calculate Factorial of a Number
- Conclusion
- FAQ
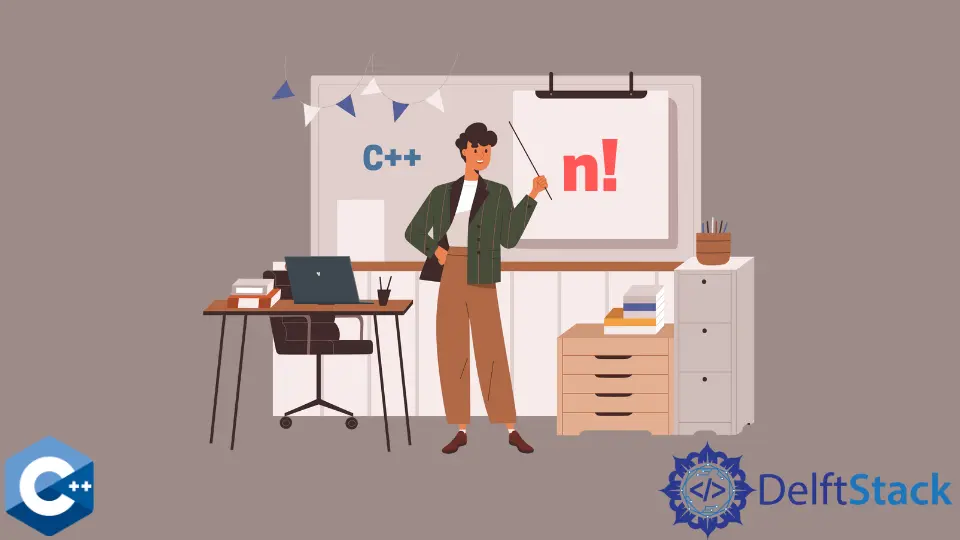
Calculating the factorial of a number is a fundamental concept in mathematics and programming. In C++, the factorial function is often used in combinatorial problems, probability calculations, and algorithm design. Whether you’re a beginner or an experienced programmer, understanding how to compute factorials can significantly enhance your coding skills.
In this article, we will explore various methods to calculate the factorial of a number in C++. We will cover both iterative and recursive approaches, providing clear code examples and explanations. By the end of this article, you will have a solid grasp of how to implement factorial calculations in C++.
Understanding Factorial
Before diving into the code, let’s clarify what a factorial is. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers less than or equal to n. For example, the factorial of 5 (5!) is calculated as:
5! = 5 × 4 × 3 × 2 × 1 = 120
Factorials grow rapidly with increasing n, making them a fascinating subject in both mathematics and programming. Now, let’s explore how to compute factorials in C++.
Use Iterative Method to Calculate Factorial of a Number in C++
The factorial of the number is calculated by multiplying all whole numbers starting from one and including the given number. Note that the straightforward algorithm is to use iteration using one of the loop statements. In the following example code, we implemented a while
loop which accumulates the multiplied values in a variable and returns the resulting integer by value to the caller. Note that the loop decrements the number in each cycle, and the n--
expression is utilized to store the value before it is decremented.
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial1(int n) {
int ret = 1;
while (n > 1) ret *= n--;
return ret;
}
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial1(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
Use Recursive Method to Calculate Factorial of a Number
Another solution is to employ recursive function calls for factorial calculation. Recursion is the feature of the function to call itself from its body. The main part of the recursive function is to define a condition that ensures that it returns to the caller and not get dragged into infinite loop-style behavior. In this case, we specify the if
condition to denote the state when the recursive call should be made. Otherwise, the function should return 1.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial2(int n) {
if (n > 1) return factorial2(n - 1) * n;
return 1;
}
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial2(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
Alternatively, we can merge the recursive function in a one-liner implementation where the ?:
expression evaluates if the given number equals 0
or 1
, in which case the function returns the 1
. If the condition is false, then the recursive call is made, and it continues with the new function stack frame until the condition is true. The example code outputs the factorial of each element in the declared vector
.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial3(int n) { return (n == 1 || n == 0) ? 1 : n * factorial3(n - 1); }
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial3(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
Conclusion
Calculating the factorial of a number in C++ can be accomplished through various methods, each with its advantages and disadvantages. The iterative method is straightforward and efficient for smaller integers, while the recursive method offers a more elegant solution but may encounter limitations with larger inputs. Understanding these methods enhances your programming skills and prepares you for more complex algorithmic challenges. Now that you have the tools to compute factorials in C++, you can apply this knowledge to various mathematical and computational problems.
FAQ
-
What is a factorial?
A factorial is the product of all positive integers up to a given number n, denoted as n!. -
Can I calculate factorials for negative numbers?
No, factorials are defined only for non-negative integers. -
What is the maximum value for which I can calculate a factorial in C++?
The maximum value depends on the data type used. Forunsigned long long
, it can handle factorials up to 20! without overflow. -
Why would I use recursion to calculate factorial?
Recursion provides a more elegant and concise solution, making the code easier to read and maintain. -
What are the practical applications of factorial?
Factorials are used in combinatorics, probability, and various algorithms, particularly in calculating permutations and combinations.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook