在 C++ 中計算數字的階乘
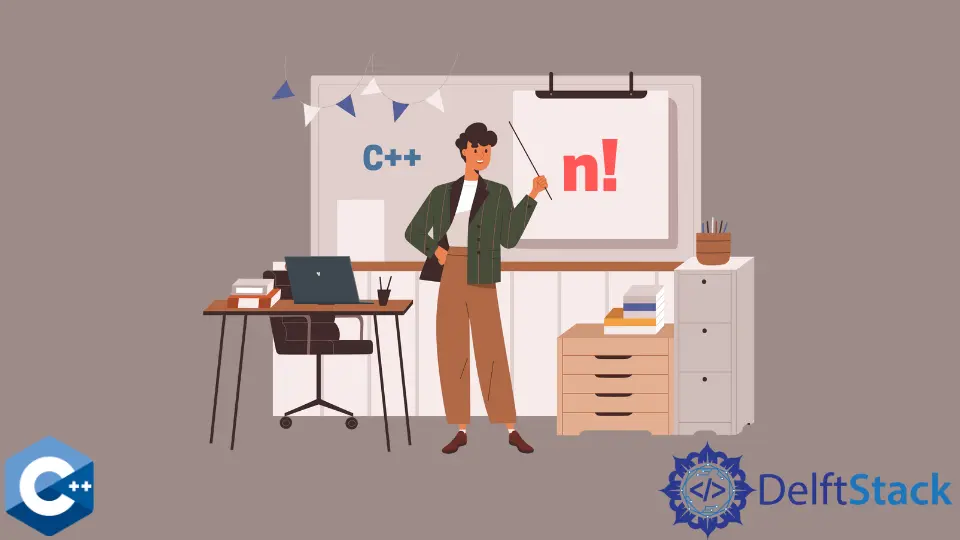
本文將介紹幾種在 C++ 中如何計算數字階乘的方法。
使用迭代法在 C++ 中計算數字的階乘
該數字的階乘是通過將所有整數從一個開始,包括給定數字相乘而得出的。注意,直接演算法是使用迴圈語句之一使用迭代。在下面的示例程式碼中,我們實現了一個 while
迴圈,該迴圈將相乘的值累加到一個變數中,然後將按值生成的整數返回給呼叫者。請注意,迴圈在每個迴圈中減小數字,並且在數值減小之前,使用 n--
表示式儲存該值。
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial1(int n) {
int ret = 1;
while (n > 1) ret *= n--;
return ret;
}
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial1(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
使用遞迴方法計算數字的階乘
另一種解決方案是採用遞迴函式呼叫進行階乘計算。遞迴是該函式從其主體進行呼叫的功能。遞迴函式的主要部分是定義一個條件,以確保它返回到呼叫方並且不會陷入無限迴圈樣式的行為中。在這種情況下,我們指定 if
條件來表示應進行遞迴呼叫的狀態。否則,該函式應返回 1。
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial2(int n) {
if (n > 1) return factorial2(n - 1) * n;
return 1;
}
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial2(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
或者,我們可以將遞迴函式合併為單行實現,其中 ?:
表示式計算給定數字等於 0
還是 1
,在這種情況下該函式返回 1
。如果條件為假,則進行遞迴呼叫,並繼續執行新的函式棧幀,直到條件為真為止。示例程式碼輸出已宣告的向量中每個元素的階乘。
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int factorial3(int n) { return (n == 1 || n == 0) ? 1 : n * factorial3(n - 1); }
template <typename T>
void printVector(vector<T> &vec) {
for (const auto &item : vec) {
cout << item << ", ";
}
cout << endl;
}
int main() {
vector<int> vec = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
printVector(vec);
for (const auto &item : vec) {
cout << factorial3(item) << ", ";
}
cout << endl;
return EXIT_SUCCESS;
}
輸出:
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
1, 2, 6, 24, 120, 720, 5040, 40320, 362880, 3628800,
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu