Trigonometric Functions in C++
- Importance of Trigonometric Functions in C++
- Trigonometric Functions in C++
- Common Pitfalls and Best Practices in Using Trigonometric Functions
- Conclusion
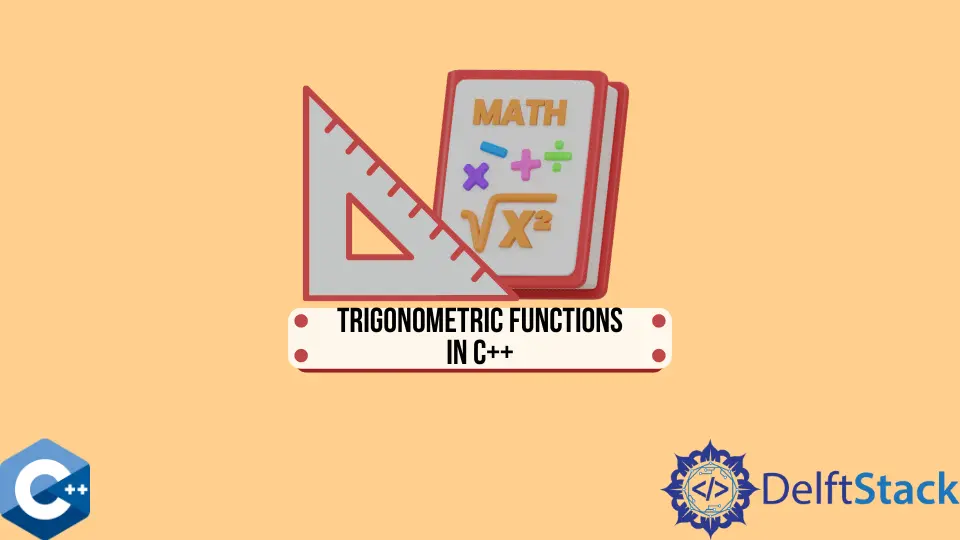
This article will explain how to use trigonometric functions of STL in C++.
Importance of Trigonometric Functions in C++
Trigonometric functions in C++ are essential for solving problems involving angles, rotations, and oscillations. They play a crucial role in various applications, including graphics programming, physics simulations, engineering calculations, and signal processing.
Understanding these functions enables precise modeling of cyclic phenomena, facilitating the development of accurate algorithms for tasks such as animation, geometry transformations, and wave analysis. Trigonometric functions are foundational tools for creating realistic visual effects, simulating physical systems, and solving mathematical problems in diverse fields.
In C++, incorporating these functions into code allows developers to achieve precision and efficiency, enhancing the capability to handle a wide range of mathematical and scientific computations with ease and accuracy.
Trigonometric Functions in C++
Understanding trigonometric functions in C++ is crucial for solving mathematical and scientific problems that involve angles and oscillatory behavior. These functions, such as sine, cosine, and tangent, are fundamental tools for modeling periodic phenomena, making them essential in fields like physics, engineering, computer graphics, and signal processing.
They enable accurate calculations related to rotations, waveforms, and geometric transformations. In C++, a solid grasp of trigonometric functions empowers developers to write efficient and precise code, ensuring the successful implementation of algorithms in various applications, from game development to scientific simulations.
Overall, proficiency in trigonometry enhances problem-solving capabilities and expands the range of problems that can be tackled in programming projects.
In C++, the trigonometric functions are part of the <cmath>
(or <math.h>
in C) header, and they are declared in the global namespace. Here is a list of the commonly used trigonometric functions in C++:
Function | Description |
---|---|
sin() |
Computes the sine of an angle. |
cos() |
Computes the cosine of an angle. |
tan() |
Computes the tangent of an angle. |
asin() |
Computes the arcsine (inverse sine) of a value. |
acos() |
Computes the arccosine (inverse cosine) of a value. |
atan() |
Computes the arctangent (inverse tangent) of a value. |
atan2() |
Computes the arctangent of the quotient of its arguments. |
sinh() |
Computes the hyperbolic sine of a value. |
cosh() |
Computes the hyperbolic cosine of a value. |
tanh() |
Computes the hyperbolic tangent of a value. |
asinh() |
Computes the inverse hyperbolic sine of a value. |
acosh() |
Computes the inverse hyperbolic cosine of a value. |
atanh() |
Computes the inverse hyperbolic tangent of a value. |
Please note that these functions operate on radians. If you have angles in degrees, you might need to convert them to radians using the formula: radians = degrees * (pi/180)
.
Additionally, it’s essential to be aware of the domain and range of these functions to avoid undefined behavior.
Let’s begin with a comprehensive code example that showcases the usage of these trigonometric functions in a cohesive manner. The following C++ program calculates and displays various trigonometric values for a given angle:
#include <cmath>
#include <iostream>
int main() {
// Angle in radians
double angle = 1.0;
// Trigonometric functions
double sinValue = sin(angle);
double cosValue = cos(angle);
double tanValue = tan(angle);
double asinValue = asin(sinValue);
double acosValue = acos(cosValue);
double atanValue = atan(tanValue);
double atan2Value = atan2(1.0, 2.0); // atan2(y, x)
double sinhValue = sinh(angle);
double coshValue = cosh(angle);
double tanhValue = tanh(angle);
double asinhValue = asinh(sinhValue);
double acoshValue = acosh(coshValue);
double atanhValue = atanh(tanhValue);
// Output the results
std::cout << "sin(" << angle << ") = " << sinValue << std::endl;
std::cout << "cos(" << angle << ") = " << cosValue << std::endl;
std::cout << "tan(" << angle << ") = " << tanValue << std::endl;
std::cout << "asin(sin(" << angle << ")) = " << asinValue << std::endl;
std::cout << "acos(cos(" << angle << ")) = " << acosValue << std::endl;
std::cout << "atan(tan(" << angle << ")) = " << atanValue << std::endl;
std::cout << "atan2(1.0, 2.0) = " << atan2Value << std::endl;
std::cout << "sinh(" << angle << ") = " << sinhValue << std::endl;
std::cout << "cosh(" << angle << ") = " << coshValue << std::endl;
std::cout << "tanh(" << angle << ") = " << tanhValue << std::endl;
std::cout << "asinh(sinh(" << angle << ")) = " << asinhValue << std::endl;
std::cout << "acosh(cosh(" << angle << ")) = " << acoshValue << std::endl;
std::cout << "atanh(tanh(" << angle << ")) = " << atanhValue << std::endl;
return 0;
}
The code begins by including the necessary headers, <iostream>
and <cmath>
, facilitating input and output operations and providing declarations for mathematical functions. The main()
function is then defined as the program’s entry point.
The angle
variable, initialized with a value of 1.0
, represents the angle in radians. Trigonometric values for sine, cosine, tangent, arcsine, arccosine, and arctangent are calculated using functions from <cmath>
.
The atan2
function computes the arctangent of the ratio 1.0/2.0
. Hyperbolic values for sine, cosine, tangent, arcsine, arccosine, and arctangent are also computed.
The results are then outputted using std::cout
, and the program concludes by returning 0
.
Output:
These values are the result of evaluating the trigonometric and hyperbolic functions with the specified angle. Keep in mind that trigonometric functions often return values in the range [-1, 1]
, and the outputs for inverse functions like asin
, acos
, and atan
represent angles in radians.
Additionally, hyperbolic functions deal with exponential growth and can produce different ranges of values. The atan2
function, in this case, calculates the arctangent of the ratio 1.0/2.0
, resulting in an angle of approximately 0.463648
radians.
Common Pitfalls and Best Practices in Using Trigonometric Functions
Common Pitfalls
Implicit Angle Unit Assumption
Developers may inadvertently assume angles are always in degrees. Trigonometric functions in C++ typically expect angles in radians. Failing to convert between units can lead to incorrect results.
Undefined Values
Using inverse trigonometric functions without validating input ranges may result in undefined values. For example, arcsine is only defined for input values within the range [-1, 1]
.
Precision Loss
Insufficient precision, especially when dealing with small angles or large values, can introduce inaccuracies in computations. This is crucial for applications requiring high precision.
Best Practices
Unit Conversion
Always convert angles to radians when using trigonometric functions. This ensures compatibility with C++ standard library conventions and avoids unexpected behavior.
Input Validation
Validate input values, especially when using inverse trigonometric functions. Check that inputs fall within the expected ranges to avoid undefined behavior.
Precision Management
Adjust the precision of calculations based on the specific requirements of the application. Using data types with higher precision may be necessary for accurate results.
Library Functions
Prefer using functions from the C++ standard library (<cmath>
) over manual implementations. Standard library functions are optimized and well-tested, reducing the chance of errors.
Avoid Redundant Calculations
Minimize redundant calculations by storing intermediate results when multiple trigonometric functions are called with the same angle. This can enhance performance in resource-intensive applications.
By adhering to these best practices and being mindful of common pitfalls, developers can leverage trigonometric functions effectively and ensure accurate results in their C++ programs.
Conclusion
Understanding trigonometric functions in C++ is paramount for solving mathematical problems, modeling cyclic phenomena, and implementing precise algorithms in various fields. These functions, like sin()
and cos()
, are fundamental tools for applications such as graphics programming, physics simulations, and engineering calculations.
To avoid common pitfalls, developers must be mindful of unit conversions, validate inputs for inverse functions, and manage precision effectively. Best practices include using standard library functions, validating inputs, and optimizing for performance.
By following these guidelines, programmers can harness the power of trigonometric functions, ensuring accuracy and efficiency in their C++ code.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook