C++ Subclass Inheritance
- C++ Subclass Inheritance
- Multiple Inheritance in Subclass in C++
- Hierarchical Inheritance in Subclass in C++
- The Diamond Problem in Inheritance in C++
- Conclusion
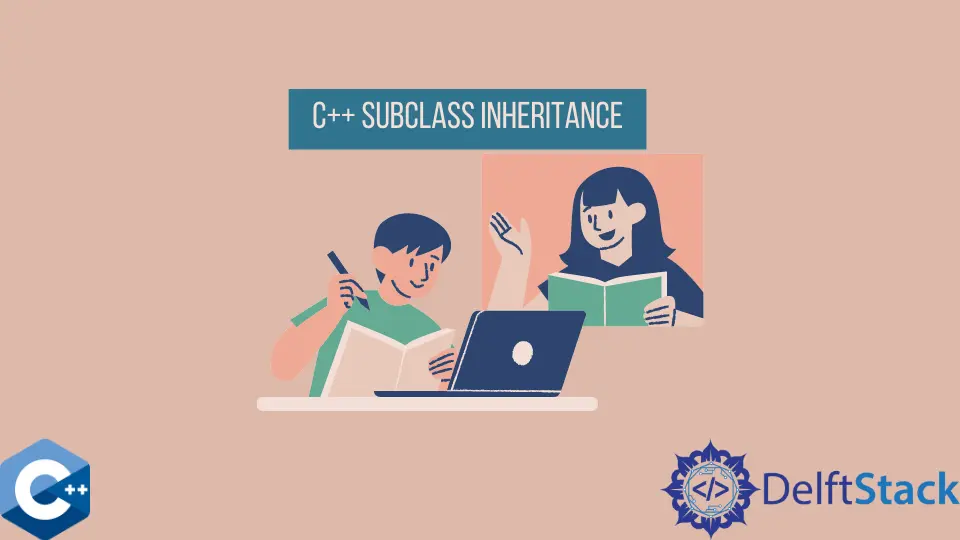
Inheritance is a feature of OOP in which a class acquires the properties and behavior of another class. A class that inherits another class is called the subclass, whereas the class whose properties are inherited is known as the base class.
This article will discuss the diamond problem that occurs while inheriting the classes.
C++ Subclass Inheritance
Inheritance is a feature that lets one class inherit the properties of another class. The class that inherits the properties of another class is known as the subclass or the derived class, whereas the class whose properties are inherited is known as the base class or the parent class.
A real-world example of inheritance can be a child inheriting the properties of its parents.
There are majorly five types of inheritance in C++, namely single inheritance, multiple inheritance, multilevel inheritance, hierarchical inheritance, and hybrid inheritance.
- Single Inheritance - In this type of inheritance, a single subclass inherits from a single base class.
- Multiple Inheritance - In this type of inheritance, the subclass is inherited from more than one base class.
- Hierarchical Inheritance - In this type of inheritance, multiple subclasses are inherited from the same base class.
- Multilevel Inheritance - In this type of inheritance, a class is inherited by another class which in turn is also inherited by some other class. For example, we have three classes,
A
,B
, andC
, in which classC
is inherited by classB
and classB
is inherited by classA
. - Hybrid Inheritance - In this type of inheritance, more than one type of inheritance is combined.
We will first talk about the multiple and hierarchical inheritance in C++.
Multiple Inheritance in Subclass in C++
As described earlier, a subclass is inherited by multiple other base classes in multiple inheritances. Let us take an example to understand multiple inheritance in detail.
#include <iostream>
using namespace std;
class Base1 {
public:
Base1() { cout << "Base class 1" << endl; }
};
class Base2 {
public:
Base2() { cout << "Base class 2" << endl; }
};
class Derived : public Base2, public Base1 {
public:
Derived() { cout << "Derived class" << endl; }
};
int main() {
Derived d;
return 0;
}
Output:
Base class 2
Base class 1
Derived class
The above code has two base classes, Base1
and Base2
, from which the Derived
class is inherited. However, you must note the order in which the constructors of the base classes are called.
First, the Base2
class constructor is called because the Derived
class inherits it first and then the Base1
constructor.
Hierarchical Inheritance in Subclass in C++
As described earlier, multiple subclasses are inherited from a single base class in hierarchical inheritance. Let’s take an example to understand the hierarchical inheritance in detail.
#include <iostream>
using namespace std;
class Base {
public:
Base() { cout << "Base class" << endl; }
};
class Derived1 : public Base {
public:
Derived1() { cout << "Derived class 1" << endl; }
};
class Derived2 : public Base {
public:
Derived2() { cout << "Derived class 2" << endl; }
};
int main() {
Derived1 d1;
Derived2 d2;
return 0;
}
Output:
Base class
Derived class 1
Base class
Derived class 2
The above code example has three classes in which the Derived1
and Derived2
classes are inherited from the common Base
class.
The Diamond Problem in Inheritance in C++
The diamond problem occurs when we combine the hierarchical and multiple inheritances. This problem is called so because the classes form a diamond shape while inheriting each other.
Let’s say we have a scenario where we have four classes A
, B
, C
, and D
.
- Class
A
acts as a base class. - Class
B
is inherited by classA
. - Class
C
is also inherited by classA
. - Class
D
is inherited by both classB
and classC
.
Now let us see the problem that occurs with the help of a code.
#include <iostream>
using namespace std;
class A {
public:
A() { cout << "Constructor A here!" << endl; }
};
class B : public A {
public:
B() { cout << "Constructor B here!" << endl; }
};
class C : public A {
public:
C() { cout << "Constructor C here!" << endl; }
};
class D : public B, public C {
public:
D() { cout << "Constructor D here!" << endl; }
};
int main() {
D d;
return 0;
}
Output:
Constructor A here!
Constructor B here!
Constructor A here!
Constructor C here!
Constructor D here!
The above output shows that the constructor of class A
has been called twice because class D
gets two copies of class A
. One by inheriting class B
and the other by inheriting class C
.
This gives rise to ambiguities and is called the diamond problem in C++.
This problem mainly occurs when a class inherits from multiple base classes inherited from a common base class.
However, this problem can be solved using the virtual
keyword in C++. We make the two-parent classes inherit from the same base class as virtual classes so that the child class does not get two copies of the common grandparent class.
Therefore, we are making the class B
and class C
virtual classes in our code example.
Let us see the solution to this problem with the help of a code example.
#include <iostream>
using namespace std;
class A {
public:
A() { cout << "Constructor A here!" << endl; }
};
class B : virtual public A {
public:
B() { cout << "Constructor B here!" << endl; }
};
class C : virtual public A {
public:
C() { cout << "Constructor C here!" << endl; }
};
class D : public B, public C {
public:
D() { cout << "Constructor D here!" << endl; }
};
int main() {
D d;
return 0;
}
Output:
Constructor A here!
Constructor B here!
Constructor C here!
Constructor D here!
Therefore, as shown in the above output, now we are getting only one copy of the constructor of class A
. The virtual
keyword tells the compiler that both class B
and class C
is inheriting from the same base class A
; therefore, it should be called only once.
Conclusion
In this article, we have briefly discussed inheritance and the types of inheritance. However, we have mainly discussed two types of inheritance- multiple and hierarchical in detail which gives rise to a problem known as the diamond problem in inheritance.
The diamond problem occurs when a class inherits from more than one base class, which is also inherited from a single base class. However, this problem is solved using the virtual
keyword in C++.