C++ Inheriting Constructors
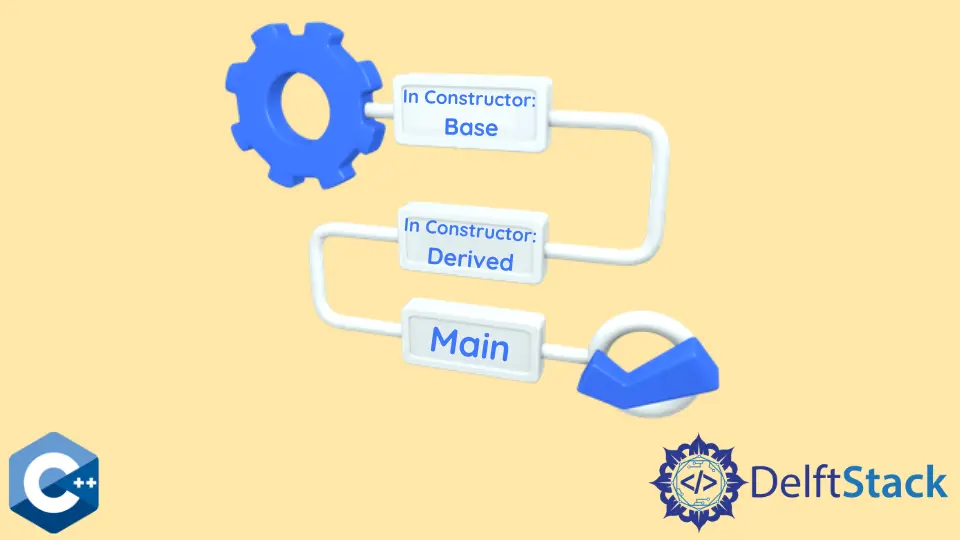
This article is about the inheritance concept in C++ and how we can inherit the base class’ constructors in the derived class.
Inheritance in C++
In C++, particularly in object-oriented programming, the most fundamental and widely used concept is that of inheritance. It is a feature in which we can define a class as a child class of another.
This also allows us to reuse some code functionalities and reduces our implementation time. It allows us to derive the functionalities and properties from the base class into the derived class.
- Base Class - The class whose properties and functionalities are used by another class is termed the base class. It is sometimes also referred to as super-class.
- Derived Class - The class that takes in the properties and functionalities of another class is termed the derived class. It is sometimes also referred to as sub-class.
Consider an example of some vehicles. Car, Bus and Truck are all automobiles, and all these automobiles have some common characteristics, and some are different.
As all of these are automobiles and all automobiles have some functionalities which are uniform among all. If we create classes for all these automobiles, we will be doing a lot of code duplication.
This is illustrated in the figure below.
You can see from the image above that all automobiles have the same functionalities. This will create duplication of code thrice.
Code duplication gives birth to many other problems such as chances of errors, increased processing time, etc. Inheritance is used to overcome such situations.
We can create an Automobile
class consisting of all these functionalities and derive the remaining classes with this class. By this, we can decrease code duplication and increase code reusability.
You can see from the above image that we have reduced the duplicate code, and all the functionalities are included in all the classes. Inheritance usually defines an is-a
relationship.
For example, a car is-a
automobile, a circle is-a
shape, a dog is-a
animal, etc.
Implementation of Inheritance in C++
We need to follow the following syntax to derive a subclass from a base class.
class derived - class - name : access - specifier base - class - name {
..
}
This access specifier is there to decide how we need to inherit the functionalities from the base class. Whether we need all of them or need some of them is decided based on the access specifier.
It can be public
, private
or protected
. If we do not specify the access specifier, it is private
by default.
Example:
public
class Automobile {
public:
getFuelAmount();
applyBrakes();
startEngine();
stopEngine();
} public class Car : public Automobile {
pressHorn();
} public class Bus : public Automobile {
pressHorn();
}
You can see from the above code that the two derived classes have only one function that is not uniform for both, as the sound of the horn is different for all the vehicles. Therefore, we have made that function in the derived class, and the rest are in the base class.
Consider another example of the Shapes
class.
#include <iostream>
#include <string>
using namespace std;
class Shapes {
public:
int w;
int h;
};
class Square : public Shapes {
public:
int getArea() { return w * h; }
};
int main() {
Square s;
s.w = 5;
s.h = 5;
cout << "Area: " << s.getArea() << endl;
return 0;
}
Output:
Area: 25
Constructor Inheritance in C++
We can see from the previous example that the functions and properties are inherited in the derived class as it is from the base class. But if we need to call the base class constructor, then we need to call it explicitly in the derived class’s constructor.
Constructors are not called automatically. Consider an example below.
#include <iostream>
using namespace std;
class baseClass {
public:
baseClass() { cout << "In constructor: Base" << endl; }
};
class derivedClass : public baseClass {
public:
derivedClass() : baseClass() { cout << "In Constructor: derived" << endl; }
};
int main() {
derivedClass d;
cout << "In main" << endl;
}
Output:
In constructor: Base
In Constructor: derived
In main
In C++11, this can be done using using-declaration
. With using-declaration
, we can use a derived class function in the base class.
The above example can be modified as follows.
#include <iostream>
using namespace std;
class baseClass {
public:
baseClass() { cout << "In constructor: Base" << endl; }
};
class derivedClass : public baseClass {
using baseClass::baseClass;
public:
derivedClass() { cout << "In Constructor: derived" << endl; }
};
int main() {
derivedClass d;
cout << "In main" << endl;
}
It will also yield the same output.
In constructor: Base
In Constructor: derived
In main
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn