Difference Between Public, Private, and Protected Inheritance in C++
- Base and Derived Classes in C++
- Public Inheritance in C++
- Protected Inheritance in C++
- Private Inheritance in C++
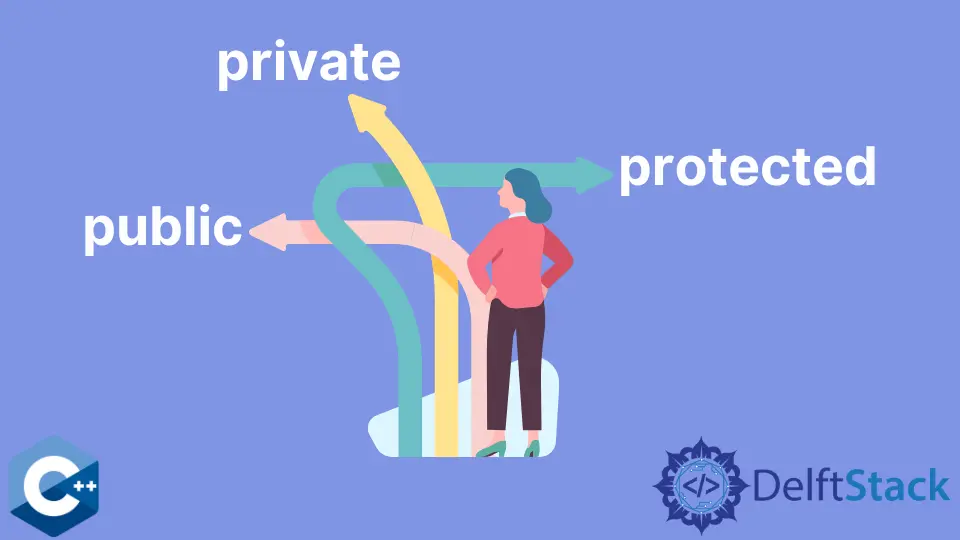
Inheritance is a fundamental concept in object-oriented programming; it helps you extend a class’s functionality. Inheritance allows you to reuse the code already written and tested in one class and use it in another, saving time, reducing errors, and simplifying your program’s design.
Inheritance can be referred to as an is-a
relationship between classes. So, for example, if we say that a car is an automobile, then we are saying that all cars are automobiles and all automobiles are cars.
In this article, we will discuss the following three types of inheritance:
- Public Inheritance
- Private Inheritance
- Protected Inheritance
But, before discussing the types of inheritance, we will discuss the concept of base and derived classes in C++.
Base and Derived Classes in C++
Base and derived classes are used to create a hierarchy of objects. The original class is called the base class, while other inherited classes are considered derived classes.
The base class gives a default implementation for all members, which can be overridden by derived classes when necessary.
A derived class can access all of its base class’s non-private members. Therefore, to prevent member functions of derived classes from accessing base-class members, those base-class members should be made private in the base class.
Public Inheritance in C++
Public inheritance is a type of inheritance in which one class acquires the features or properties of another class. It can be seen as an implementation of the is-a
relationship, where the inheriting class is considered an extension or specialization of the inherited ones.
When a class is derived from a public base class, the base class’s public members become the derived class’s public members, and the base class’s protected members become the derived class’s protected members. The private members of a base class are never readily reachable from a derived class, but they can be retrieved via calls to the base class’s public and protected members.
#include <iostream>
using namespace std;
class Demo {
private:
int x = 56;
protected:
int y = 27;
public:
int z = 67;
int getX() { return x; }
};
class PublicInheritance : public Demo {
public:
int getY() { return y; }
};
int main() {
PublicInheritance sam;
cout << "Private member = " << sam.getX() << endl;
cout << "Protected member= " << sam.getY() << endl;
cout << "Public member= " << sam.z << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Protected Inheritance in C++
Protected inheritance is a form of inheritance where the base class is protected. This means that the derived class will have all the public and protected members of the base class as protected members.
Deriving from a protected base class has its benefits in specific scenarios. For example, this would be an appropriate solution if you want to create a set of related classes but don’t want to make them public because they are only meant for internal use.
#include <iostream>
using namespace std;
class Demo {
private:
int x = 56;
protected:
int y = 27;
public:
int z = 67;
int getX() { return x; }
};
class ProtectedInheritance : protected Demo {
public:
int getY() { return y; }
int getZ() { return z; }
};
int main() {
ProtectedInheritance sam;
cout << "Protected member= " << sam.getY() << endl;
cout << "Public member= " << sam.getZ() << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Private Inheritance in C++
Private inheritance is when the derived class inherits from a private base class. This means that any public or protected base class members become private members of the derived class.
This can be useful when you want to create a subclass that provides only some of the features of its superclass.
#include <iostream>
using namespace std;
class Demo {
private:
int x = 56;
protected:
int y = 27;
public:
int z = 67;
int getX() { return x; }
};
class PrivateInheritance : private Demo {
public:
int getY() { return y; }
int getZ() { return z; }
};
int main() {
PrivateInheritance sam;
cout << "Protected member= " << sam.getY() << endl;
cout << "Public member= " << sam.getZ() << endl;
return 0;
}
Click here to check the working of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook