How to Use Private vs Protected Class Members in C++
-
Use the
private
Property to Denote the Class Members Inaccessible to Users of the Class in C++ -
Use the
protected
Property to Denote the Class Members Accessible to Member Functions of Derived Class or Friend Class
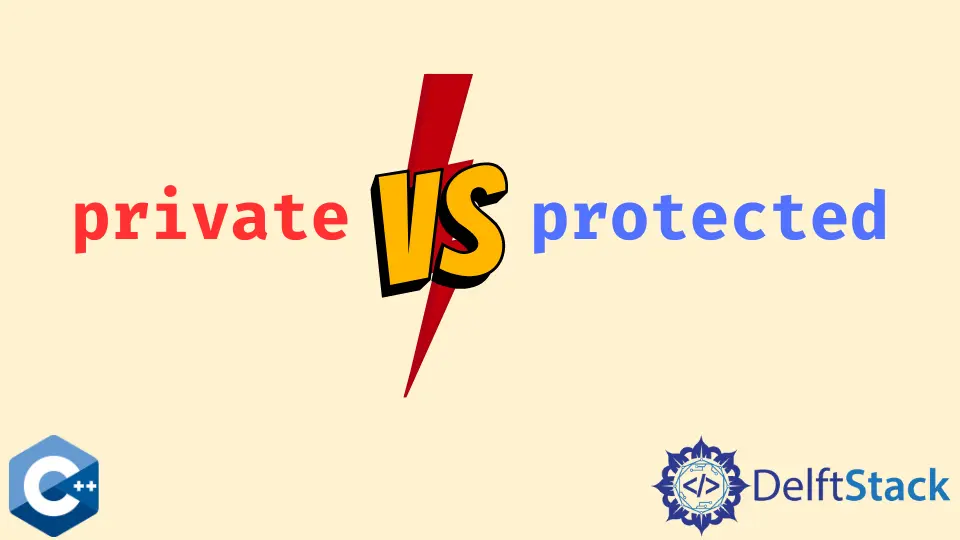
This article will demonstrate multiple methods about how to use private
vs protected
class members correctly in C++.
Use the private
Property to Denote the Class Members Inaccessible to Users of the Class in C++
The private
keyword is one of the fundamental parts of the C++ language to implement encapsulation features. The encapsulation’s main goal is to create an enforced interface for the class users and restrict direct access to only specific members. Note that defining the class interface implies that the user of the class need not modify or access data members directly but rather call public methods that are designed to do these operations on the given objects.
There are generally three keywords for access control: public
, private
, and protected
. Members defined after public
property is accessible to all users of the class. On the other hand, the private
specifier defines the members that can only be accessed by the class’s member functions. In the following example, code from the main
function can declare a variable of type CustomString
, but to access its member str
the code needs to call the getString
method, defined as public
property.
#include <iostream>
#include <string>
#include <utility>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
class CustomString {
public:
CustomString() = default;
explicit CustomString(const string& s) : str(s) { num = s.size(); }
virtual ~CustomString() = default;
string& getString() { return str; };
private:
string str;
protected:
int num{};
};
int main() {
CustomString str1("Hello There 1");
cout << "str1: " << str1.getString() << endl;
exit(EXIT_SUCCESS);
}
Output:
str1: Hello There 1
Use the protected
Property to Denote the Class Members Accessible to Member Functions of Derived Class or Friend Class
Another keyword available for access control is a protected
property that makes the members declared after accessible to class member functions, derived class members, and even friend classes. Notice that the two of the later ones cannot access the protected
members directly from the base class object but rather from the derived class object. The next example demonstrates the CustomSentence
class that derives from the CustomString
, but the num
member of the latter class is a protected
member. Thus, it can’t be accessed from the CustomString
object. Namely, the getSize
function is not part of the CustomString
class, and only CustomSentence
objects can retrieve the num
value by calling the method.
#include <iostream>
#include <string>
#include <utility>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
class CustomString {
public:
CustomString() = default;
explicit CustomString(const string& s) : str(s) { num = s.size(); }
virtual ~CustomString() = default;
string& getString() { return str; };
private:
string str;
protected:
int num{};
};
class CustomSentence : public CustomString {
public:
CustomSentence() = default;
explicit CustomSentence(const string& s) : sent(s) { num = s.size(); }
~CustomSentence() override = default;
int getSize() const { return num; };
string& getSentence() { return sent; };
private:
string sent;
};
int main() {
CustomString str1("Hello There 1");
CustomSentence sent1("Hello There 2");
cout << "str1: " << str1.getString() << endl;
cout << "sent1: " << sent1.getSentence() << endl;
cout << "size: " << sent1.getSize() << endl;
exit(EXIT_SUCCESS);
}
Output:
str1: Hello There 1
sent1: Hello There 2
size: 13
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook