How to Download a File in C++
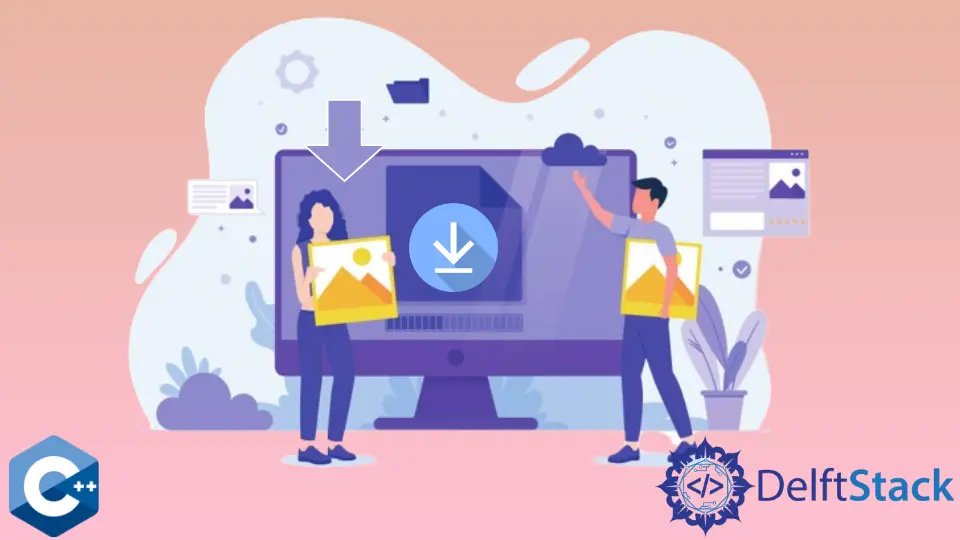
This tutorial demonstrates how to download a file using C++.
Download a File in C++
Downloading a file using C++ is an easy operation that can be done using the win32
API URLDownloadToFile
. This API can download a file in our computer from the given link.
We can save the downloaded item as a file or string based on our needs. Both of these can be done using different operations.
Let’s start with downloading as a file.
C++ Download as a File
As mentioned above, we can use the win32
API URLDownloadToFile
, we can download any file from the given link. The file will be saved in the same working directory.
Let’s try an example.
#include <windows.h>
#include <cstdio>
#include <string>
#pragma comment(lib, "Urlmon.lib")
using namespace std;
int main() {
// the URL from where the file will be downloaded
string SourceURL = "https://picsum.photos/200/300.jpg";
// destination file
string DestinationFile = "DemoFile.jpg";
// URLDownloadToFile returns S_OK on success
if (S_OK == URLDownloadToFile(NULL, SourceURL.c_str(),
DestinationFile.c_str(), 0, NULL)) {
printf("The file is successfully downloaded.");
return 0;
}
else {
printf("Download Failed");
return -1;
}
}
Before compiling the above code, it should be mentioned here that the above code will only work with the NSVC
compiler using Visual Studio Express, Visual C++ or any other related one. It will not work with the MinGW
compiler because it doesn’t contain the URLDownloadToFile
API.
Once we run the above code, it will download the file from the link.
See the output:
The file is successfully downloaded.
C++ Download as a String of Bytes
This method uses the win32
API URLOpenBlockingStream
to download the file as a string of bytes which can also be saved in a file. This is a tricky step-by-step process; follow the steps below.
- First, use the
URLOpenBlockingStream
to get anIStream
interface to the URL. - Then insert the URL where both HTTP and HTTPS protocols will work.
- Once the
IStream
is made available, use theread
function to download the bytes. We may need to use a loop here. - Once the bytes are collected in a string, we can either save them to a file or download the string.
Let’s try an example based on the above steps and try to get the Google homepage in bytes.
#pragma comment(lib, "urlmon.lib")
#include <urlmon.h>
#include <cstdio>
#include <iostream>
#include <string>
#define getURL URLOpenBlockingStreamA
using namespace std;
int main() {
IStream* DemoStream;
const char* SourceURL = "http://google.com";
if (getURL(0, SourceURL, &DemoStream, 0, 0)) {
cout << "An Error has occured.";
cout << "Please Check the internet";
cout << "Please Check the source URL.";
return -1;
}
// this char array will be filled with bytes from the URL
char DemoBuff[100];
// keep appending the bytes to this string
string DemoStr;
unsigned long Bytes_Read;
while (true) {
DemoStream->Read(DemoBuff, 100, &Bytes_Read);
if (0U == Bytes_Read) {
break;
}
// appending and collecting to the string
DemoStr.append(DemoBuff, Bytes_Read);
};
DemoStream->Release();
cout << DemoStr << endl;
return 0;
}
The code above will get the Google homepage in a string of bytes; though it’s not efficient to download something in a string of bytes, we can also save the bytes in a file and use it later. The output for the above code will be a long string of bytes.
Make sure to run the above code with the same compiler as the first code because the URLOpenBlockingStream
will also not work with the MinGW
C++ compiler.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook