C++ でファイルをダウンロードする
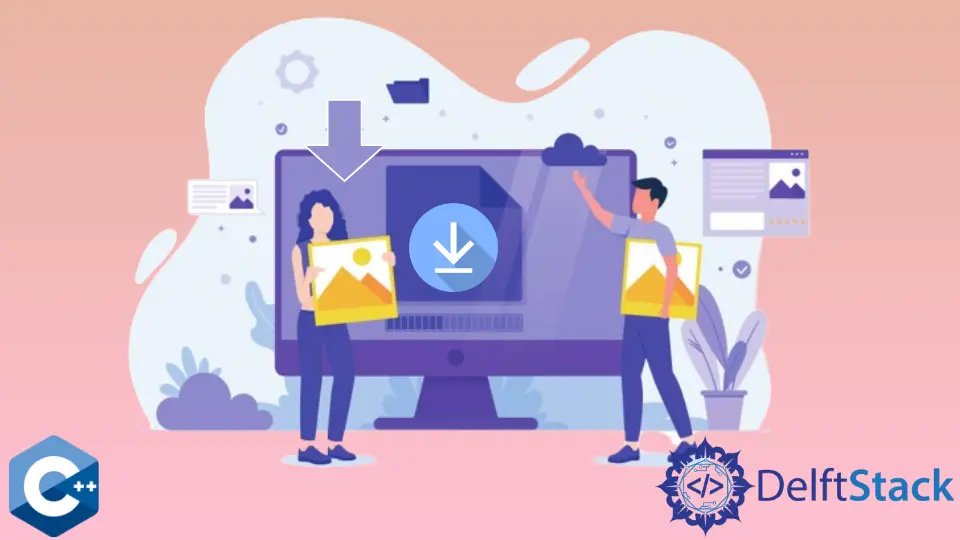
このチュートリアルでは、C++ を使用してファイルをダウンロードする方法を示します。
C++ でファイルをダウンロードする
C++ を使用したファイルのダウンロードは、win32
API [URLDownloadToFile
] を使用して実行できる簡単な操作です。 この API は、指定されたリンクからコンピューターにファイルをダウンロードできます。
必要に応じて、ダウンロードしたアイテムをファイルまたは文字列として保存できます。 これらは両方とも、異なる操作を使用して実行できます。
ファイルとしてダウンロードすることから始めましょう。
C++ ファイルとしてダウンロード
上記のように、win32
API [URLDownloadToFile
]) を使用できます。指定されたリンクから任意のファイルをダウンロードできます。 ファイルは同じ作業ディレクトリに保存されます。
例を試してみましょう。
#include <windows.h>
#include <cstdio>
#include <string>
#pragma comment(lib, "Urlmon.lib")
using namespace std;
int main() {
// the URL from where the file will be downloaded
string SourceURL = "https://picsum.photos/200/300.jpg";
// destination file
string DestinationFile = "DemoFile.jpg";
// URLDownloadToFile returns S_OK on success
if (S_OK == URLDownloadToFile(NULL, SourceURL.c_str(),
DestinationFile.c_str(), 0, NULL)) {
printf("The file is successfully downloaded.");
return 0;
}
else {
printf("Download Failed");
return -1;
}
}
上記のコードをコンパイルする前に、上記のコードは、Visual Studio Express、Visual C++、またはその他の関連するコンパイラを使用する NSVC
コンパイラでのみ機能することに注意してください。 URLDownloadToFile
API が含まれていないため、MinGW
コンパイラーでは機能しません。
上記のコードを実行すると、リンクからファイルがダウンロードされます。
出力を参照してください。
The file is successfully downloaded.
バイト文字列としての C++ ダウンロード
このメソッドは、win32
API URLOpenBlockingStream
を使用して、ファイルに保存できるバイト文字列としてファイルをダウンロードします。 これはトリッキーな段階的なプロセスです。 以下の手順に従ってください。
- まず、
URLOpenBlockingStream
を使用して、URL へのIStream
インターフェイスを取得します。 - 次に、HTTP プロトコルと HTTPS プロトコルの両方が機能する URL を挿入します。
IStream
が利用可能になったら、read
関数を使用してバイトをダウンロードします。 ここでループを使用する必要があるかもしれません。- バイトが文字列に収集されたら、それらをファイルに保存するか、文字列をダウンロードできます。
上記の手順に基づいた例を試して、Google ホームページをバイト単位で取得してみましょう。
#pragma comment(lib, "urlmon.lib")
#include <urlmon.h>
#include <cstdio>
#include <iostream>
#include <string>
#define getURL URLOpenBlockingStreamA
using namespace std;
int main() {
IStream* DemoStream;
const char* SourceURL = "http://google.com";
if (getURL(0, SourceURL, &DemoStream, 0, 0)) {
cout << "An Error has occured.";
cout << "Please Check the internet";
cout << "Please Check the source URL.";
return -1;
}
// this char array will be filled with bytes from the URL
char DemoBuff[100];
// keep appending the bytes to this string
string DemoStr;
unsigned long Bytes_Read;
while (true) {
DemoStream->Read(DemoBuff, 100, &Bytes_Read);
if (0U == Bytes_Read) {
break;
}
// appending and collecting to the string
DemoStr.append(DemoBuff, Bytes_Read);
};
DemoStream->Release();
cout << DemoStr << endl;
return 0;
}
上記のコードは、Google ホームページをバイト文字列で取得します。 何かをバイト列でダウンロードするのは効率的ではありませんが、バイトをファイルに保存して後で使用することもできます。 上記のコードの出力は、長いバイト文字列になります。
URLOpenBlockingStream
は MinGW
C++ コンパイラでも機能しないため、最初のコードと同じコンパイラで上記のコードを実行してください。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook