C++에서 파일 다운로드
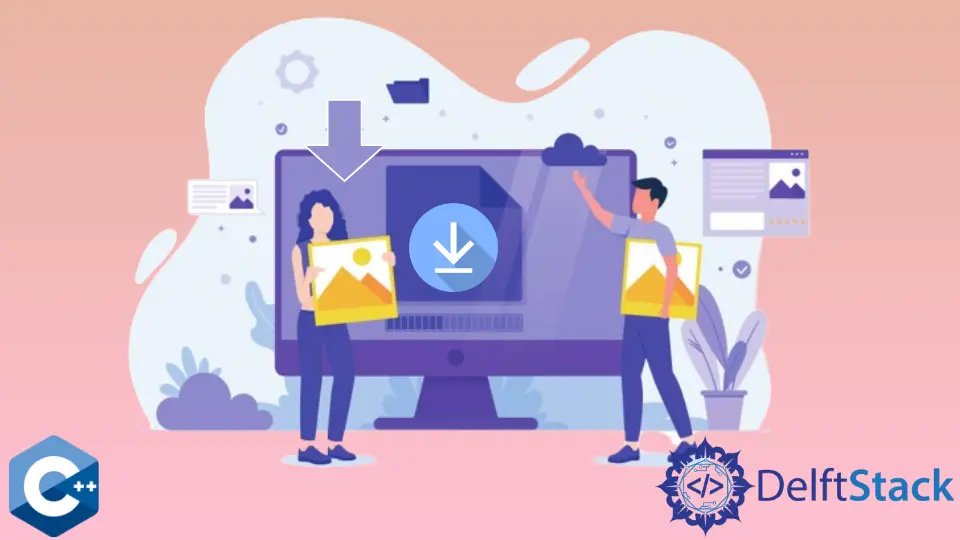
이 자습서에서는 C++를 사용하여 파일을 다운로드하는 방법을 보여줍니다.
C++에서 파일 다운로드
C++를 사용하여 파일을 다운로드하는 것은 win32
API [URLDownloadToFile
])를 사용하여 수행할 수 있는 쉬운 작업입니다. 이 API는 주어진 링크에서 우리 컴퓨터의 파일을 다운로드할 수 있습니다.
필요에 따라 다운로드한 항목을 파일 또는 문자열로 저장할 수 있습니다. 이 두 가지 작업은 서로 다른 작업을 사용하여 수행할 수 있습니다.
파일로 다운로드하는 것부터 시작하겠습니다.
C++ 파일로 다운로드
위에서 언급했듯이 win32
API [URLDownloadToFile
])를 사용할 수 있으며 주어진 링크에서 모든 파일을 다운로드할 수 있습니다. 파일은 동일한 작업 디렉토리에 저장됩니다.
예를 들어 보겠습니다.
#include <windows.h>
#include <cstdio>
#include <string>
#pragma comment(lib, "Urlmon.lib")
using namespace std;
int main() {
// the URL from where the file will be downloaded
string SourceURL = "https://picsum.photos/200/300.jpg";
// destination file
string DestinationFile = "DemoFile.jpg";
// URLDownloadToFile returns S_OK on success
if (S_OK == URLDownloadToFile(NULL, SourceURL.c_str(),
DestinationFile.c_str(), 0, NULL)) {
printf("The file is successfully downloaded.");
return 0;
}
else {
printf("Download Failed");
return -1;
}
}
위의 코드를 컴파일하기 전에 위의 코드는 Visual Studio Express, Visual C++ 또는 기타 관련 항목을 사용하는 NSVC
컴파일러에서만 작동한다는 점을 여기서 언급해야 합니다. URLDownloadToFile
API가 포함되어 있지 않기 때문에 MinGW
컴파일러에서는 작동하지 않습니다.
위의 코드를 실행하면 링크에서 파일을 다운로드합니다.
출력을 참조하십시오.
The file is successfully downloaded.
바이트 문자열로 C++ 다운로드
이 방법은 win32
API URLOpenBlockingStream
을 사용하여 파일을 파일에 저장할 수도 있는 바이트 문자열로 다운로드합니다. 이는 까다로운 단계별 프로세스입니다. 아래 단계를 따르십시오.
- 먼저
URLOpenBlockingStream
을 사용하여 URL에 대한IStream
인터페이스를 가져옵니다. - 그런 다음 HTTP 및 HTTPS 프로토콜이 모두 작동할 URL을 삽입합니다.
IStream
을 사용할 수 있게 되면read
기능을 사용하여 바이트를 다운로드합니다. 여기서 루프를 사용해야 할 수도 있습니다.- 바이트가 문자열로 수집되면 파일에 저장하거나 문자열을 다운로드할 수 있습니다.
위의 단계를 기반으로 예제를 시도하고 바이트 단위로 Google 홈페이지를 가져오도록 합시다.
#pragma comment(lib, "urlmon.lib")
#include <urlmon.h>
#include <cstdio>
#include <iostream>
#include <string>
#define getURL URLOpenBlockingStreamA
using namespace std;
int main() {
IStream* DemoStream;
const char* SourceURL = "http://google.com";
if (getURL(0, SourceURL, &DemoStream, 0, 0)) {
cout << "An Error has occured.";
cout << "Please Check the internet";
cout << "Please Check the source URL.";
return -1;
}
// this char array will be filled with bytes from the URL
char DemoBuff[100];
// keep appending the bytes to this string
string DemoStr;
unsigned long Bytes_Read;
while (true) {
DemoStream->Read(DemoBuff, 100, &Bytes_Read);
if (0U == Bytes_Read) {
break;
}
// appending and collecting to the string
DemoStr.append(DemoBuff, Bytes_Read);
};
DemoStream->Release();
cout << DemoStr << endl;
return 0;
}
위의 코드는 바이트 문자열로 Google 홈페이지를 가져옵니다. 바이트 문자열로 무언가를 다운로드하는 것은 효율적이지 않지만 파일에 바이트를 저장하고 나중에 사용할 수도 있습니다. 위 코드의 출력은 긴 바이트 문자열입니다.
URLOpenBlockingStream
은 MinGW
C++ 컴파일러에서도 작동하지 않으므로 첫 번째 코드와 동일한 컴파일러로 위 코드를 실행해야 합니다.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook