How to Add Timed Delay in C++
-
Use the
sleep()
System Call to Add a Timed Delay in C++ -
Use the
usleep()
Function to Add a Timed Delay in C++ -
Use the
sleep_for()
Function to Add a Timed Delay in C++ -
Use the
sleep_until()
Function to Add a Timed Delay in C++ - Conclusion
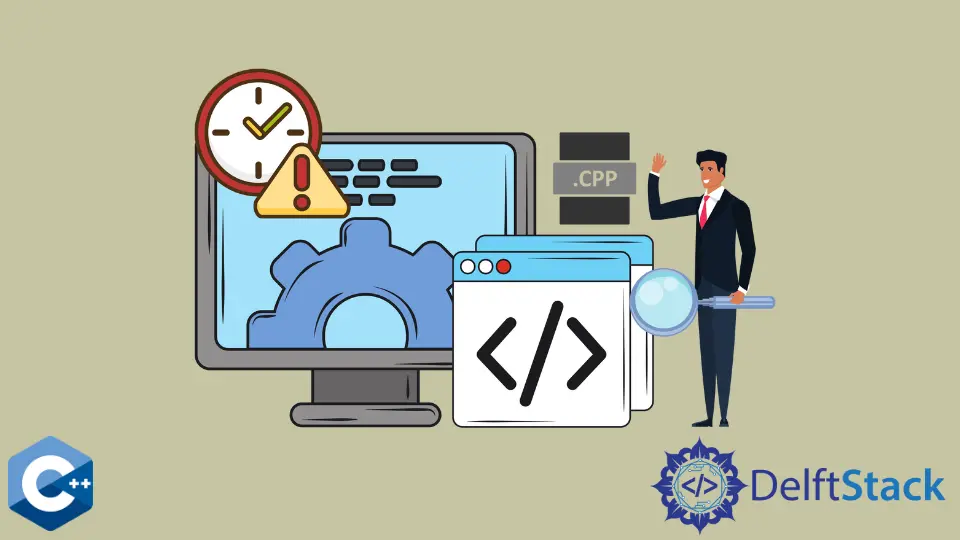
This tutorial will briefly guide you on adding a timed delay in your C++ program.
This can be done in many ways using C++ libraries’ functions. We will discuss some functions along with their working demo.
Any process, task, or thread in a computer program can sleep or become inactive. This means the execution of the process can be put on hold for the time it is in sleep
.
When the sleep interval expires, or a signal or interruption causes the execution to resume, the execution will be resumed. There are different methods for this purpose:
- the
sleep()
system call - the
usleep()
method - the
sleep_for()
method - the
sleep_until()
method
Use the sleep()
System Call to Add a Timed Delay in C++
A sleep
system call can put a program (task, process, or thread) to sleep. The time
parameter in a typical sleep
system call tells how much time the program needs to sleep or remain inactive.
The C++ language does not have its sleep
function. This functionality is provided by operating-system-specific files such as unistd.h
for Linux and Windows.h
for Windows.
To use the sleep()
function on a Linux or UNIX operating system, we must include the "unistd.h"
header file in our program.
Function Prototype
unsigned sleep(unsigned seconds);
It takes a parameter amount of seconds for which we need to suspend the execution and return 0 if it successfully returns to the execution. If the sleep
is interrupted in between, the total time minus the interruption time is returned.
Example Code:
#ifdef _WIN32
#include <Windows.h>
#else
#include <unistd.h>
#endif
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
cout << "Before sleep call" << endl;
cout.flush();
#ifdef _WIN32
Sleep(9000); // Sleep for 9000 milliseconds (9 seconds) on Windows
#else
sleep(9); // Sleep for 9 seconds on other platforms
#endif
cout << "After sleep call" << endl;
return 0;
}
In the header inclusion section, it uses preprocessor directives to include the appropriate header file based on the platform. On Windows (_WIN32
is defined), it includes <Windows.h>
, and on other platforms, it includes <unistd.h>
.
Next is setting the necessary standard C++ library headers for handling input and output (<iostream>
) and for general utilities (<cstdlib>
).
For the main
function of the code snippet, it outputs the message Before sleep call
to the console, followed by a new line. cout.flush()
ensures that the output is immediately written to the console.
Then, the conditional compilation (#ifdef _WIN32
) is used to determine the platform.
If on Windows, it calls Sleep(9000)
from <Windows.h>
, causing the program to pause execution for 9000 milliseconds (9 seconds). On other platforms, it uses sleep(9)
from <unistd.h>
for the same purpose.
After the sleep
, it prints After sleep call
to the console, followed by a new line.
Output:
Before sleep call
After sleep call
In the output, we have printed two statements. After printing the first one, the compiler waits for 9 seconds, and then the other is printed. Readers should execute the program to observe this delay.
Use the usleep()
Function to Add a Timed Delay in C++
Another function in the header unistd.h
is usleep()
, which allows you to pause the execution of a program for a set amount of time. The operation is identical to the previously described sleep()
function.
The function usleep()
suspends the caller thread’s execution for useconds microseconds
or until the signal interrupting the execution is sent.
Function Prototype
int usleep(useconds_t useconds);
This function takes as a parameter the no of microseconds for which you need to pause the execution and return 0 if successfully resumed and -1 if some failure occurs.
Example Code:
#include <unistd.h>
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
cout << "Hello ";
cout.flush();
usleep(1000000);
cout << "World";
cout << endl;
return 0;
}
This time, we only include <unistd.h>
, which contains declarations for various functions, including usleep
, which is used for microsecond-level sleep
.
Similarly to the method above, we set the necessary standard C++ library headers for handling input and output (<iostream>
) and for general utilities (<cstdlib>
).
For our main
function, the code outputs the string Hello
to the console using the cout
object. The cout.flush()
ensures that the output is immediately written to the console.
Pauses the program for 1000000 microseconds (1 second) using the usleep
function. This introduces a small delay between printing Hello
and World
. After the set time, the string World
will be printed to the console.
Output:
Hello World
As you can see in the above code snippet, we have provided a wait of 1000000 microseconds between printing two statements.
In C++ 11, we are provided with specific functions for making thread sleep. There are two methods for this purpose, sleep_for
and sleep_until
.
Let’s discuss these two methods.
Use the sleep_for()
Function to Add a Timed Delay in C++
The function sleep_for()
is defined in the <thread>
header. The sleep_for ()
function suspends the current thread’s execution for the duration specified in sleep duration.
Due to scheduling activities or resource contention delays, this function may be blocked for longer than the provided period.
Function Prototype
template <class Rep, class Period>
void sleep_for(const std::chrono::duration<Rep, Period>& sleep_duration);
Example Code:
#include <chrono>
#include <iostream>
#include <thread>
using namespace std;
int main() {
cout << "Hello I'm here before waiting" << endl;
this_thread::sleep_for(chrono::milliseconds(10000));
cout << "I waited for 10000 ms\n";
return 0;
}
In this method, we included the following header files: <chrono>
which provides facilities for performing time-related operations, <thread>
which provides facilities for creating, managing, and synchronizing threads, and <iostream>
which includes declarations for input/output stream objects, used for console output (cout
).
In this code snippet, it intends to output the string Hello I'm here before waiting
to the console using the cout
object, followed by a new line.
It will pause the program for 10000 milliseconds (10 seconds) using this_thread::sleep_for
. This function is part of the <thread>
header and allows the program to sleep for a specified duration.
After the pause time, it outputs the string I waited for 10000 ms
to the console, indicating that the program has completed the 10-second wait.
Output:
Hello I'm here before waiting
I waited for 10000 ms
In the above code snippet, we have made the main thread sleep for 10000 milliseconds, which means that the current thread will block 10000 milliseconds and then resume its execution.
Use the sleep_until()
Function to Add a Timed Delay in C++
The <thread>
header defines this function. The sleep_until ()
method stops a thread from running till the sleep time has passed.
Due to scheduling activities or resource contention delays, this function, like the others, may be blocked for longer than the specified time.
Function Prototype
template <class Clock, class Duration>
void sleep_until(const std::chrono::time_point<Clock, Duration>& sleep_time);
It takes in as a parameter the period for which the thread needs to be blocked and returns nothing.
Example Code:
#include <chrono>
#include <iostream>
#include <thread>
using namespace std;
int main() {
cout << "Hello I'm here before waiting" << endl;
// Syntax for sleep_until
std::this_thread::sleep_until(std::chrono::system_clock::now() +
std::chrono::seconds(3));
cout << "I waited for 3 seconds\n";
return 0;
}
In this method, we included the same header files in the sleep_for()
method.
For the sleep_until
function, it uses std::this_thread::sleep_until
to pause the program until 3 seconds from the current time. It calculates the future time point by adding the current time (std::chrono::system_clock::now()
) and a duration of 3 seconds (std::chrono::seconds(3)
).
Output:
Hello I'm here before waiting
I Waited for 2 seconds
It outputs the string Hello I'm here before waiting
to the console using the cout
object, followed by a new line. Then, it waits for a duration of 3 seconds to continue executing the code.
Conclusion
In conclusion, this tutorial provides a comprehensive guide to adding timed delays in C++ programs using various methods.
It covers the use of the sleep()
system call and usleep()
method for platform-specific delays, along with the more versatile <chrono>
library’s sleep_for()
and sleep_until()
methods.
The examples illustrate how to introduce delays with precision, making it adaptable for different timing requirements. Whether it’s a simple pause or a specific wait until a future time point, these methods enhance the control and synchronization of program execution.
The tutorial caters to both novice and experienced programmers seeking effective ways to manage time-related operations in C++.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn