Enhance Effectiveness of windows.h in C++
-
Define
NOMINMAX
in a C++ Program to Enhance the Effectiveness ofWindows.h
-
Use the
WIN32_LEAN_AND_MEAN
to Enhance the Effectiveness ofWindows.h
in C++ -
Include Sub-Headers to Replace
Windows.h
in a C++ Program to Enhance Its Effectiveness
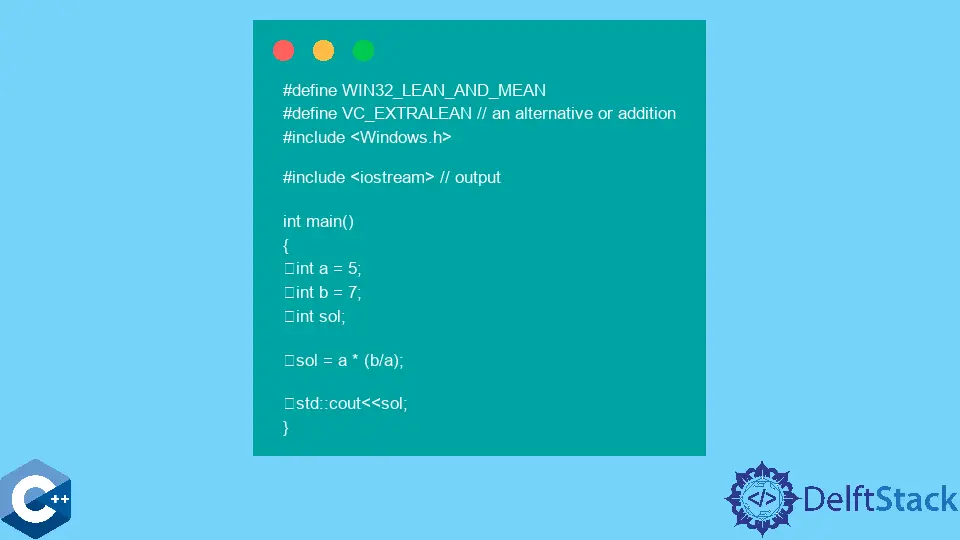
It’s universally accepted that #include <Windows.h>
is as bad practice as #include <bits/stdc++.h>
header file.
In this tutorial, you will learn the truth behind the usefulness of Windows.h
and whether it’s a bad or good practice in C++.
Because windows.h
includes/parses each standard header (the majority of them are unnecessary and nonportable) and even more when combined with using namespace std;
, it features a large number of common names in your C++ program’s namespace like next
which left you unable to use the other features inefficiently.
However, it’s a primary requirement for the Win32 programs even if it performs the same as bits/stdc++.h
and using namespace std;
combined.
Define NOMINMAX
in a C++ Program to Enhance the Effectiveness of Windows.h
MSDN documentation related to windows.h
explicitly defines which header files to include to perform a particular function. It’s not a bad practice to include windows.h
only when you disable its unnecessary features before including it via the macro.
Define NOMINMAX
before including windows.h
in your C++ program because otherwise, you will get access to a min
and a max
macro that will interface with std::min
and std::max
.
The windows.h
is an operating-system-specific header and comes with the WSDK (Windows Software Development Kit).
Its use is suggested to increase the usefulness of your #include
directives under Windows; however, it can prove extremely complex for entry-level or intermediate C++ developers, so they should use the windows.h
.
In general, do not use windows.h
in any situation where a function is an intention because the key to good programming in C++ is to never use macros in the place of a function.
// operating system (OS) specific
#define NOMINMAX // minimize the `windows.h` library
#include <Windows.h>
#include <iostream> // for output
/* an alternative to `#define NOMINMAX`
- you can define min and max macro in the following way
#ifndef NOMINMAX
#define min(x,y) ((x) < (y) ? (x) : (y))
#define max(x,y) ((x) > (y) ? (x) : (y))
#endif
*/
// no use of `using namespace std;` | increase complexity and decrease
// efficiency
int main() { std::cout << "Successful!"; }
Output:
Successful!
As a Windows-specific header file, it holds all the declarations for Windows API functions, common macros, and data types for various functions and subsystems and defines extremely large Windows-specific functions as a part of your C++ program.
It is a part of nearly every Windows-based C++ program because of no reasonable alternative. You can further compile it to exclude some unnecessary functions and pre-defined variables.
Use the WIN32_LEAN_AND_MEAN
to Enhance the Effectiveness of Windows.h
in C++
When WIN32_LEAN_AND_MEAN
is defined before the call to windows.h
, it will bypass some of the less useful or required Windows headers. It’s one of the faster solutions to increase windows.h
effectiveness.
Use VC_EXTRALEAN
or WIN32_LEAN_AND_MEAN
to reduce the size of the windows.h
header file, but using any one of these preprocessors sometimes missing your needs, and you need to include another header file.
Use MSDN search to name a function you want to use so that it can tell you which header file it belongs to.
It excludes less useful or specific-purpose APIs such as DDE, Windows Sockets, RPC, Shell, and Cryptography.
Furthermore, the documentation for OPENFILENAMEW
says that the commdlg.h
is a part of windows.h
, but WIN32_LEAN_AND_MEAN
excludes it from windows.h
, and you can include it separately because the commdlg.h
uses macros defined in windows.h
.
#define WIN32_LEAN_AND_MEAN
#define VC_EXTRALEAN // an alternative or addition
#include <Windows.h>
#include <iostream> // output
int main() {
int a = 5;
int b = 7;
int sol;
sol = a * (b / a);
std::cout << sol;
}
Output:
5
As it excludes some of the less frequently used Windows APIs, it speeds up the build process and reduces the size of Win32 header files.
The windows.h
includes it and many other sub-headers; hence specifically defining one can maximize the resources of your C++ processes.
Include Sub-Headers to Replace Windows.h
in a C++ Program to Enhance Its Effectiveness
The sub-headers of windows.h
seem to be structured in a fairly stable way. You can include one of the many smaller headers to define your needs instead of using windows.h
as a whole and only using its one or two features.
The windef.h
contains many of the base functions of windows.h
and is based on more than seven thousand lines of actual code. The fileapi.h
defines CreateFile
, FindFirstFile
, and other similar functions.
#include <fileapi.h>
#include <windef.h>
#include <iostream>
int main() {
typedef struct tagPOINT {
LONG x;
LONG y;
}
POINT,
*PPOINT, *NPPOINT, *LPPOINT;
std::cout << "The operation is successful!";
}
Output:
The operation is successful!
Additionally, you can use synchapi.h
for synchronization primitives (WaitForMultipleObjects
, InitializeCriticalSection
, or other synch functions part of windows.h
).
The debugapi.h
sub-header file is for OutputDebugString
, IsDebuggerPresent
, and other debugging functions.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub