How to Create a Header File in C++
-
Use
.h
or.hpp
Suffixes to Create a Header File in C++ - Use Header Files to Break Down Separate Function Blocks of the Program in Modules
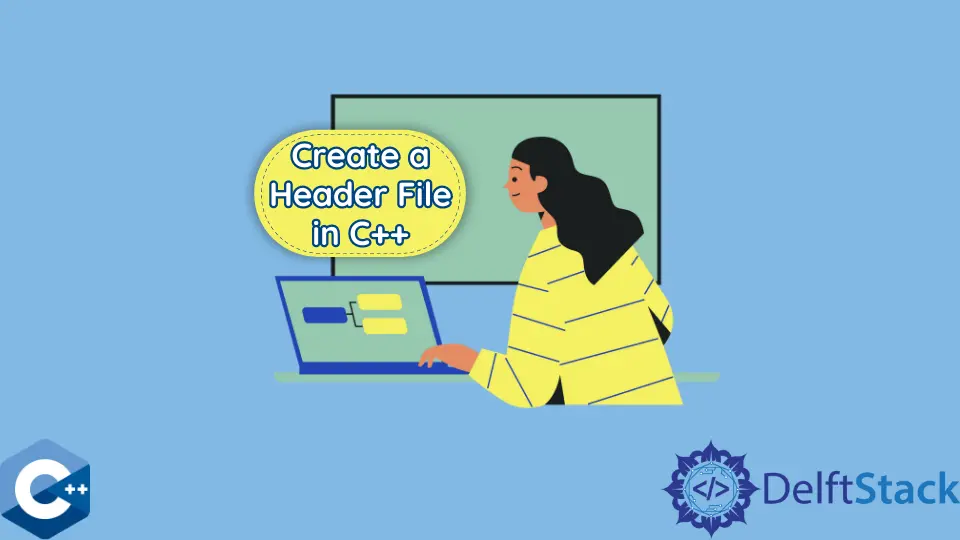
This article will explain several methods of how to create a header file in C++.
Use .h
or .hpp
Suffixes to Create a Header File in C++
Contemporary programs are rarely written without libraries, which are code constructs implemented by others. C++ provides special token - #include
to import needed library header files and external functions or data structures. Note that, generally, library header files have a particular filename suffix like library_name.h
or library_name.hpp
. C++ program structure provides the concept of header files to ease the usage of certain reusable code blocks. Thus, users can create their own header files and include them in source files as needed.
Suppose that the user needs to implement a class named Point
containing two data members of type double
. The class has two constructors and +
operator defined in it. It also has a print
function to output both data members’ values to the cout
stream. Generally, there are also header guards enclosing the Point
class definition to ensure no name clashes occur when including in relatively large programs. Note that there should be a consistent naming scheme for the variables names defined after include guard; usually, these variables are named after the class itself.
#ifndef POINT_H
#define POINT_H
class Point {
double x, y;
public:
Point();
Point(double, double);
Point operator+(const Point &other) const;
void print();
};
#endif
Another method to structure a header file for the Point
class is to include the function implementation code in the same file. Note that putting the previous code snippet into a Point.hpp
file and including it will raise several undefined errors. Since the functions are defined in the following example code, we can include it as a Point.hpp
header file and use the class with its methods.
#include <iostream>
#include <vector>
#ifndef POINT_H
#define POINT_H
class Point {
double x, y;
public:
Point();
Point(double, double);
Point operator+(const Point &other) const;
void print();
};
Point::Point() { x = y = 0.0; }
Point::Point(double a, double b) {
x = a;
y = b;
}
Point Point::operator+(const Point &other) const {
return {x + other.x, y + other.y};
}
void Point::print() { std::cout << "(" << x << "," << y << ")" << std::endl; }
#endif
Use Header Files to Break Down Separate Function Blocks of the Program in Modules
Alternatively, one can utilize a module-based separation scheme for headers and corresponding source files of the given class to implement a more flexible project file structure. In this design, one should define each functionally distinct class in a separate .hpp
header file and implement its methods in a source file with the same name. Once the class’s needed header file is included in the main source file and compiled, the preprocessor will combine the code blocks from all included header files, and the result would be the same as compiling the following source code, which implements the functionality in a single source file.
#include <iostream>
#include <vector>
#ifndef POINT_H
#define POINT_H
class Point {
double x, y;
public:
Point();
Point(double, double);
Point operator+(const Point &other) const;
void print();
};
Point::Point() { x = y = 0.0; }
Point::Point(double a, double b) {
x = a;
y = b;
}
Point Point::operator+(const Point &other) const {
return {x + other.x, y + other.y};
}
void Point::print() { std::cout << "(" << x << "," << y << ")" << std::endl; }
#endif
using std::cin;
using std::cout;
int main() {
double x, y;
cin >> x >> y;
Point a1(x, y);
cin >> x >> y;
Point a2(x, y);
cout << "a1: ";
a1.print();
cout << "a2: ";
a2.print();
a1 = a1 + a2;
cout << "a1+a2: ";
a1.print();
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook