How to Access Private Members of a Class in C++
-
Use
private
Access Specifier to Encapsulate Class Members in C++ -
Use
public
Functions to Retrieve Private Members of a Class in C++
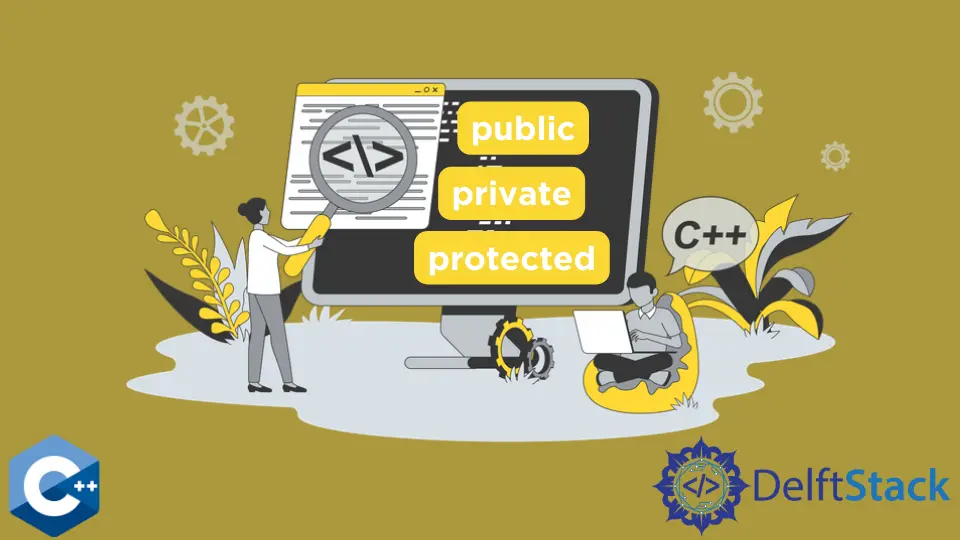
This article will explain several methods of how to access private members of a class in C++.
Use private
Access Specifier to Encapsulate Class Members in C++
Access specifiers are employed to implement a core feature of object-oriented programming - called encapsulation. As a result, we restrict the direct access to specific data members of the class and essentially construct an interface to operate on class data. The C++ provides several access specifier keywords like: public
, private
and protected
, which usually precede the members of the class that need to be qualified with corresponding accessibility.
Members defined after the private
specifier are accessible to member functions only and can’t be referred directly from the code that uses the class. A class is usually constructed with two sides in mind - the designer of the class and the user of the class. The latter is usually the one who is affected by the encapsulation. If the programmer defines members before the first access specifier, their accessibility is by default set to private
when the class
keyword is used, and public
on the struct
keyword.
In the following example, we implement a class called BaseClass
with two string data members declared as private
, so to access the values of these members, the designer of the class should include public
functions that retrieve them. Notice that we can remove the public
keyword from the code below and still have getUsername
and getName
functions as public
members.
Another perk of the encapsulation is the flexibility to modify the internal class structure without worrying about the user side’s compatibility issues. As long as the interface, thus, public functions don’t change, the user of the class does not need to modify her code.
#include <iostream>
#include <string>
#include <utility>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
class BaseClass {
public:
BaseClass() = default;
explicit BaseClass(string user, string nm)
: username(std::move(user)), name(std::move(nm)) {}
~BaseClass() = default;
string& getUsername() { return username; };
string& getName() { return name; };
private:
string username;
string name;
};
int main() {
BaseClass base("buddy", "Buddy Bean");
cout << "base -> name: " << base.getName() << endl;
cout << "base -> username: " << base.getUsername() << endl;
exit(EXIT_SUCCESS);
}
Output:
base -> name: Buddy Bean
base -> username: buddy
Use public
Functions to Retrieve Private Members of a Class in C++
private
members can be modified using the class interface functions, e.g changeUsername
function takes string
argument from the user of the class and it stores its value to the private
member - username
. Note that there is also a friend
keyword that distinguishes other classes and functions allowed to access the private
members of the class. These functions can be external, not part of the class mentioned above. Mind though, the incorrect usage of access specifiers most likely results in compiler errors.
#include <iostream>
#include <string>
#include <utility>
#include <vector>
using std::cout;
using std::endl;
using std::string;
using std::vector;
class BaseClass {
public:
BaseClass() = default;
explicit BaseClass(string user, string nm)
: username(std::move(user)), name(std::move(nm)) {}
~BaseClass() = default;
void changeUsername(const string& s) { username.assign(s); };
string& getUsername() { return username; };
string& getName() { return name; };
private:
string username;
string name;
};
int main() {
BaseClass base("buddy", "Buddy Bean");
cout << "base -> name: " << base.getName() << endl;
cout << "base -> username: " << base.getUsername() << endl;
base.changeUsername("jolly");
cout << "base -> username: " << base.getUsername() << endl;
exit(EXIT_SUCCESS);
}
Output:
base -> name: Buddy Bean
base -> username: buddy
base -> username: jolly
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook