Excess Elements in Scalar Initializer Warning in C
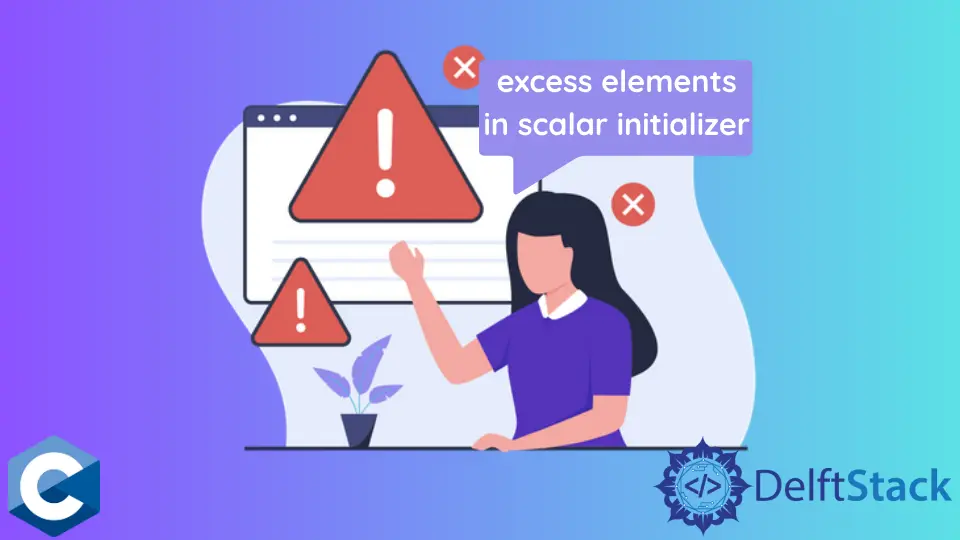
When working with C, you may encounter a warning message that says "excess elements in scalar initializer."
. This warning comes when we initialize the array with too many elements.
In this guide, we’ll dive into the details of this warning and how to resolve it.
Solve the Warning Message excess elements in scalar initializer
in C
Example Code 1:
#include <stdio.h>
int main(void) {
int array[2][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Output:
In function 'main':
[Warning] excess elements in array initializer
[Warning] (near initialization for 'array')
The above error comes because the declared is int[2][3][4]
, but we are trying to initialize it as if it were an int [3][3][4]
.
To resolve this error, we have to correct the size of the array.
Corrected Code(Example 1):
#include <stdio.h>
int main(void) {
int array[3][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19, 0}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Let’s have another example.
Example code 2:
#include <stdio.h>
int main(void) {
static char(*check)[13] = {
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13},
{11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130}};
// Your code here
return 0;
}
We also get the same warning; the compiler gives the warning because we have passed two-pointers, but only a single pointer to an array of 13
elements exists. More elements than necessary are declared.
We can resolve this in two ways.
Corrected Code 1 (Example 2):
#include <stdio.h>
int main(void) {
// Define two character arrays
char ar1[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13};
char ar2[] = {11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130};
// Create an array of character pointers and initialize it with the addresses
// of ar1 and ar2
char *check[] = {ar1, ar2};
// Print the elements in ar1
printf("ar1: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", ar1[i]);
}
printf("\n");
// Print the elements in ar2
printf("ar2: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", ar2[i]);
}
printf("\n");
// Print the elements using the check array of pointers
printf("check[0]: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", check[0][i]);
}
printf("\n");
printf("check[1]: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Output:
ar1: 1 2 3 4 5 6 7 8 9 10 11 12 13
ar2: 11 22 33 44 55 66 77 88 99 100 110 120 130
check[0]: 1 2 3 4 5 6 7 8 9 10 11 12 13
check[1]: 11 22 33 44 55 66 77 88 99 100 110 120 130
Corrected Code 2 (Example 2): We have only one array pointer. The pointer points to an array of 10
elements. We can increment the array pointer to grab the next 10
elements.
#include <stdio.h>
int main(void) {
char(*check)[10] = (char[][10]){{1, 2, 3, 4, 5, 6, 7, 8, 9, 10},
{0, 31, 29, 31, 30, 31, 30, 31, 31, 30}};
// Print the elements in the first sub-array
printf("check[0]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[0][i]);
}
printf("\n");
// Print the elements in the second sub-array
printf("check[1]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Output:
check[0]: 1 2 3 4 5 6 7 8 9 10
check[1]: 0 31 29 31 30 31 30 31 31 30