Éléments en excès dans l'avertissement de l'initialiseur scalaire en C
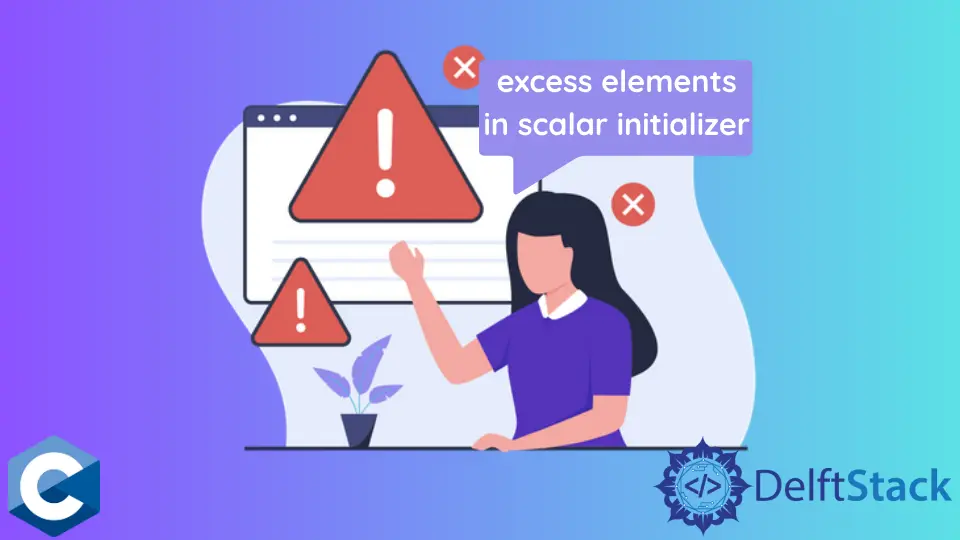
Lorsque vous travaillez avec C, vous pouvez rencontrer un message d’avertissement qui dit "excess elements in scalar initializer."
. Cet avertissement apparaît lorsque nous initialisons le tableau avec trop d’éléments.
Dans ce guide, nous allons examiner les détails de cet avertissement et comment le résoudre.
Résoudre le message d’avertissement excess elements in scalar initializer
en C
Code exemple 1 :
#include <stdio.h>
int main(void) {
int array[2][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Sortie :
In function 'main':
[Warning] excess elements in array initializer
[Warning] (near initialization for 'array')
L’erreur ci-dessus se produit parce que le tableau déclaré est int [2][3][4]
, mais nous essayons de l’initialiser comme s’il s’agissait d’un int [3][3][4]
.
Pour résoudre cette erreur, nous devons corriger la taille du tableau.
Code corrigé (Exemple 1) :
#include <stdio.h>
int main(void) {
int array[3][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19, 0}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Prenons un autre exemple.
Code exemple 2 :
#include <stdio.h>
int main(void) {
static char(*check)[13] = {
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13},
{11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130}};
// Your code here
return 0;
}
Nous obtenons également le même avertissement ; le compilateur donne l’avertissement car nous avons passé deux pointeurs, mais seul un seul pointeur vers un tableau de 13
éléments existe. Plus d’éléments que nécessaire sont déclarés.
Nous pouvons résoudre cela de deux manières.
Code corrigé 1 (Exemple 2) :
#include <stdio.h>
int main(void) {
// Define two character arrays
char ar1[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13};
char ar2[] = {11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130};
// Create an array of character pointers and initialize it with the addresses
// of ar1 and ar2
char *check[] = {ar1, ar2};
// Print the elements in ar1
printf("ar1: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", ar1[i]);
}
printf("\n");
// Print the elements in ar2
printf("ar2: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", ar2[i]);
}
printf("\n");
// Print the elements using the check array of pointers
printf("check[0]: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", check[0][i]);
}
printf("\n");
printf("check[1]: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Sortie :
ar1: 1 2 3 4 5 6 7 8 9 10 11 12 13
ar2: 11 22 33 44 55 66 77 88 99 100 110 120 130
check[0]: 1 2 3 4 5 6 7 8 9 10 11 12 13
check[1]: 11 22 33 44 55 66 77 88 99 100 110 120 130
Code corrigé 2 (Exemple 2) : Nous n’avons qu’un seul pointeur de tableau. Le pointeur pointe vers un tableau de 10
éléments. Nous pouvons incrémenter le pointeur de tableau pour obtenir les 10
éléments suivants.
#include <stdio.h>
int main(void) {
char(*check)[10] = (char[][10]){{1, 2, 3, 4, 5, 6, 7, 8, 9, 10},
{0, 31, 29, 31, 30, 31, 30, 31, 31, 30}};
// Print the elements in the first sub-array
printf("check[0]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[0][i]);
}
printf("\n");
// Print the elements in the second sub-array
printf("check[1]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Sortie :
check[0] : 1 2 3 4 5 6 7 8 9 10
check[1] : 0 31 29 31 30 31 30 31 31 30