Exceso de elementos en advertencia de inicializador escalar en C
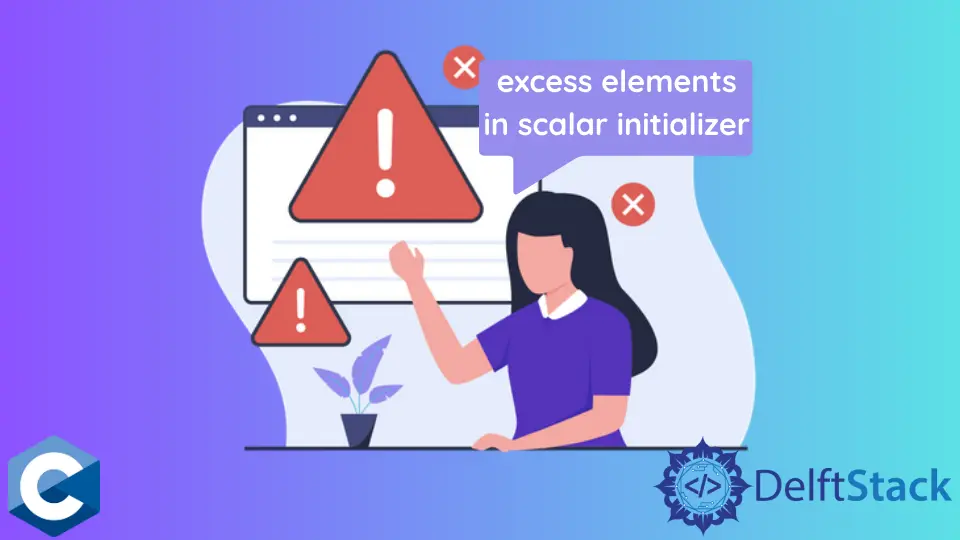
Al trabajar con C, es posible que te encuentres con un mensaje de advertencia que dice "excess elements in scalar initializer."
. Esta advertencia aparece cuando inicializamos el array con demasiados elementos.
En esta guía, profundizaremos en los detalles de esta advertencia y cómo resolverla.
Solucionar el mensaje de advertencia excess elements in scalar initializer
en C
Código de ejemplo 1:
#include <stdio.h>
int main(void) {
int array[2][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Salida:
In function 'main':
[Warning] excess elements in array initializer
[Warning] (near initialization for 'array')
El error anterior ocurre porque se declara como int[2][3][4]
, pero estamos intentando inicializarlo como si fuera un int[3][3][4]
.
Para resolver este error, debemos corregir el tamaño del array.
Código corregido (Ejemplo 1):
#include <stdio.h>
int main(void) {
int array[3][3][4] = {
{{11, 22, 33}, {44, 55, 66}, {0, 0, 0, 0}},
{{161, 102, 13}, {104, 15, 16}, {107, 18, 19, 0}},
{{100, 20, 30, 400}, {500, 60, 70, 80}, {960, 100, 110, 120}}};
// Your code here
return 0;
}
Veamos otro ejemplo.
Código de ejemplo 2:
#include <stdio.h>
int main(void) {
static char(*check)[13] = {
{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13},
{11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130}};
// Your code here
return 0;
}
También obtenemos la misma advertencia; el compilador da la advertencia porque hemos pasado dos punteros, pero solo existe un solo puntero a un array de 13
elementos. Hay más elementos de los necesarios declarados.
Podemos resolver esto de dos formas.
Código corregido 1 (Ejemplo 2):
#include <stdio.h>
int main(void) {
// Define two character arrays
char ar1[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13};
char ar2[] = {11, 22, 33, 44, 55, 66, 77, 88, 99, 100, 110, 120, 130};
// Create an array of character pointers and initialize it with the addresses
// of ar1 and ar2
char *check[] = {ar1, ar2};
// Print the elements in ar1
printf("ar1: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", ar1[i]);
}
printf("\n");
// Print the elements in ar2
printf("ar2: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", ar2[i]);
}
printf("\n");
// Print the elements using the check array of pointers
printf("check[0]: ");
for (int i = 0; i < sizeof(ar1) / sizeof(ar1[0]); i++) {
printf("%d ", check[0][i]);
}
printf("\n");
printf("check[1]: ");
for (int i = 0; i < sizeof(ar2) / sizeof(ar2[0]); i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Salida:
ar1: 1 2 3 4 5 6 7 8 9 10 11 12 13
ar2: 11 22 33 44 55 66 77 88 99 100 110 120 130
check[0]: 1 2 3 4 5 6 7 8 9 10 11 12 13
check[1]: 11 22 33 44 55 66 77 88 99 100 110 120 130
Código corregido 2 (Ejemplo 2): Solo tenemos un puntero a un array. El puntero apunta a un array de 10
elementos. Podemos incrementar el puntero al array para tomar los siguientes 10
elementos.
#include <stdio.h>
int main(void) {
char(*check)[10] = (char[][10]){{1, 2, 3, 4, 5, 6, 7, 8, 9, 10},
{0, 31, 29, 31, 30, 31, 30, 31, 31, 30}};
// Print the elements in the first sub-array
printf("check[0]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[0][i]);
}
printf("\n");
// Print the elements in the second sub-array
printf("check[1]: ");
for (int i = 0; i < 10; i++) {
printf("%d ", check[1][i]);
}
printf("\n");
return 0;
}
Salida:
check[0]: 1 2 3 4 5 6 7 8 9 10
check[1]: 0 31 29 31 30 31 30 31 31 30