How to Get Size of a Pointer in C
- Pointers in C Programming Language
-
Use the
sizeof()
Method to Get the Size of a Pointer in C -
Use the
malloc()
Method to Get the Size of a Pointer in C
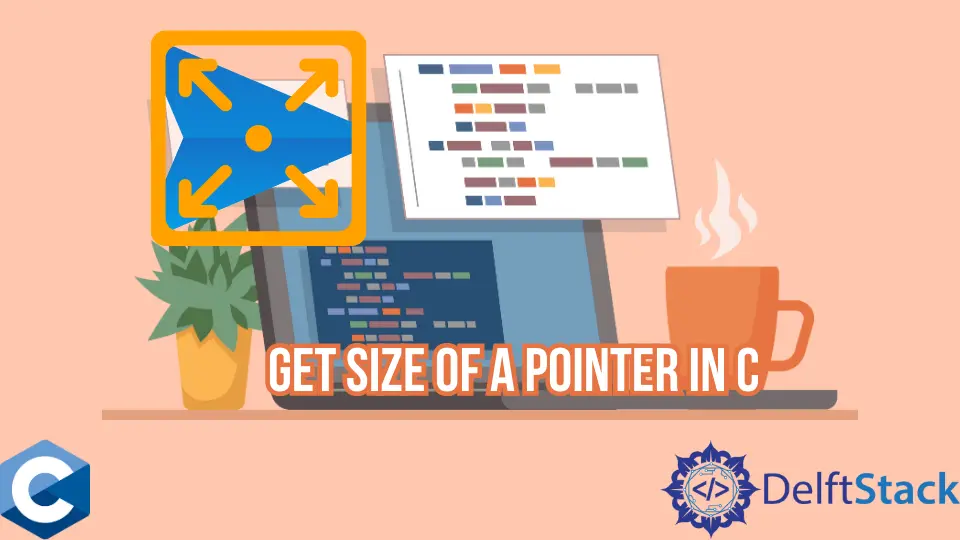
This article demonstrates how to determine the size of a pointer using the C programming language.
Pointers in C Programming Language
A variable known as a pointer is one whose value is the address of another variable; in other words, the pointer itself contains the direct address of the memory location. Before a pointer can be used to hold the location of a variable, it must first be declared.
Syntax:
type *var - name;
Here, the type
refers to the pointer’s base type
, it should be a valid data type, and the var-name
is the name
of the variable that stores the pointer. It’s important to note that the asterisk(*
) used to designate a pointer is the same asterisk used for multiplication.
On the other hand, the asterisk(*
) is used in this sentence to indicate that a particular variable is a pointer. The following are some of the different datatype declarations acceptable for pointers.
int *integerPointer;
char *characterPointer;
float *floatPointer;
double *doublePointer;
Use the sizeof()
Method to Get the Size of a Pointer in C
The sizeof()
method only accepts one parameter. It is an operator whose value is determined by the compiler; for this reason, it is referred to as a compile-time unary operator.
The sizeof()
function returns the unsigned integral data type.
Let’s have an example in which we’ll use the sizeof()
function to determine the size of various variables whose datatypes differ. Set the initial values for four variables named a
, b
, c
and d
using the datatypes int
, char
, float
and double
respectively.
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
After initializing the four pointer variables with the names *integerPointer,
*characterPointer,
*floatPointer,
and *doublePointer,
assign the values a
, b
, c
, and d
to them respectively.
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
Now, we need some printf
commands to output the size of the variables and the pointer variables.
These statements are required to utilize the sizeof
operator, and the parameters that should be supplied to it are a
, b
, c
, d
, and accordingly *integerPointer
, *characterPointer
, *floatPointer
and *doublePointer
.
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
The notation %lu
is used because the result of the sizeof
method is an unsigned integer.
Complete Source Code:
#include <stdio.h>
int main() {
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
return 0;
}
Output:
size of (a): = 4 bytes
size of (a) pointer: = 4 bytes
size of (b): = 1 bytes
size of (b) pointer: = 1 bytes
size of (c): = 4 bytes
size of (c) pointer: = 4 bytes
size of (d): = 8 bytes
size of (d) pointer: = 8 bytes
Use the malloc()
Method to Get the Size of a Pointer in C
Let us look at one more example of a pointer variable, using the malloc
technique to allocate the size.
Inside the main()
function, initiate a pointer variable with the datatype char
and name it *cPointer
. Assign a function with the name malloc()
and provide it with thesizeof()
method as an argument.
The sizeof
method accepts char *
as its argument.
int main() { char *cPointer = malloc(sizeof(char *)); }
At this point, we must print the value of *cPointer
in bytes.
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
Complete Source Code:
#include <stdio.h>
#include <stdlib.h>
int main() {
char *cPointer = malloc(sizeof(char *));
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
return 0;
}
Output:
The size of the pointer is 1 byte.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn