C でポインターのサイズを取得する
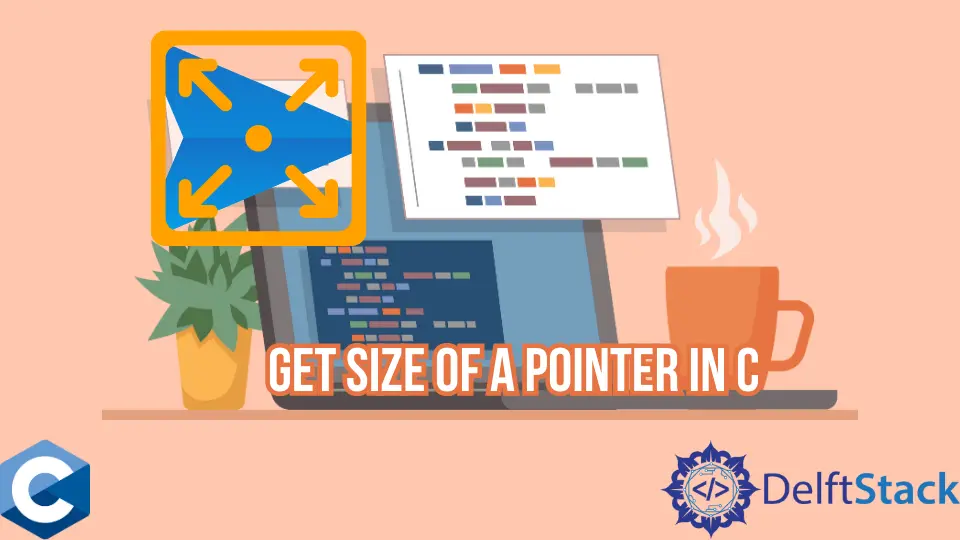
この記事では、C プログラミング言語を使用してポインターのサイズを確認する方法について説明します。
C プログラミング言語のポインター
ポインターと呼ばれる変数は、値が別の変数のアドレスである変数です。 つまり、ポインタ自体には、メモリ位置の直接アドレスが含まれています。 ポインターを使用して変数の位置を保持するには、まずポインターを宣言する必要があります。
構文:
type *var - name;
ここで、type
はポインターの base type
を参照し、有効なデータ型である必要があり、var-name
はポインターを格納する変数の name
です。 ポインターを指定するために使用されるアスタリスク (*
) は、乗算に使用されるアスタリスクと同じであることに注意することが重要です。
一方、アスタリスク (*
) は、この文で特定の変数がポインターであることを示すために使用されます。 以下は、ポインターに使用できるさまざまなデータ型宣言の一部です。
int *integerPointer;
char *characterPointer;
float *floatPointer;
double *doublePointer;
sizeof()
メソッドを使用して C でポインターのサイズを取得する
sizeof()
メソッドは 1つのパラメーターのみを受け入れます。 これは、コンパイラによって値が決定される演算子です。 このため、コンパイル時単項演算子と呼ばれます。
sizeof()
関数は符号なし整数データ型を返します。
sizeof()
関数を使用して、データ型が異なるさまざまな変数のサイズを決定する例を見てみましょう。 データ型 int
、 char
、 float
および double
をそれぞれ使用して、a
、 b
、 c
および d
という名前の 4つの変数の初期値を設定します。
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
*integerPointer
、 *characterPointer
、*floatPointer
および *doublePointer
という名前の 4つのポインター変数を初期化した後、値 a
、b
、c
および d
を それらをそれぞれ。
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
ここで、変数のサイズとポインター変数を出力するためにいくつかの printf
コマンドが必要です。
これらのステートメントは、sizeof
演算子を使用するために必要であり、これに指定する必要があるパラメーターは、a
、b
、c
、d
で、それに応じて *integerPointer
、*characterPointer
、*floatPointer
と *doublePointer
が与えられる。
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
sizeof
メソッドの結果は符号なし整数であるため、表記法 %lu
が使用されます。
完全なソース コード:
#include <stdio.h>
int main() {
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
return 0;
}
出力:
size of (a): = 4 bytes
size of (a) pointer: = 4 bytes
size of (b): = 1 bytes
size of (b) pointer: = 1 bytes
size of (c): = 4 bytes
size of (c) pointer: = 4 bytes
size of (d): = 8 bytes
size of (d) pointer: = 8 bytes
C でmalloc()
メソッドを使用して ポインターのサイズを取得する
malloc
手法を使用してサイズを割り当てるポインター変数の例をもう 1つ見てみましょう。
main()
関数内で、データ型 char
のポインター変数を開始し、*cPointer
という名前を付けます。 malloc()
という名前の関数を割り当て、sizeof()
メソッドを引数として提供します。
sizeof
メソッドは、引数として char *
を受け入れます。
int main() { char *cPointer = malloc(sizeof(char *)); }
この時点で、*cPointer
の値をバイト単位で出力する必要があります。
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
完全なソース コード:
#include <stdio.h>
#include <stdlib.h>
int main() {
char *cPointer = malloc(sizeof(char *));
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
return 0;
}
出力:
The size of the pointer is 1 byte.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn