C에서 포인터의 크기 얻기
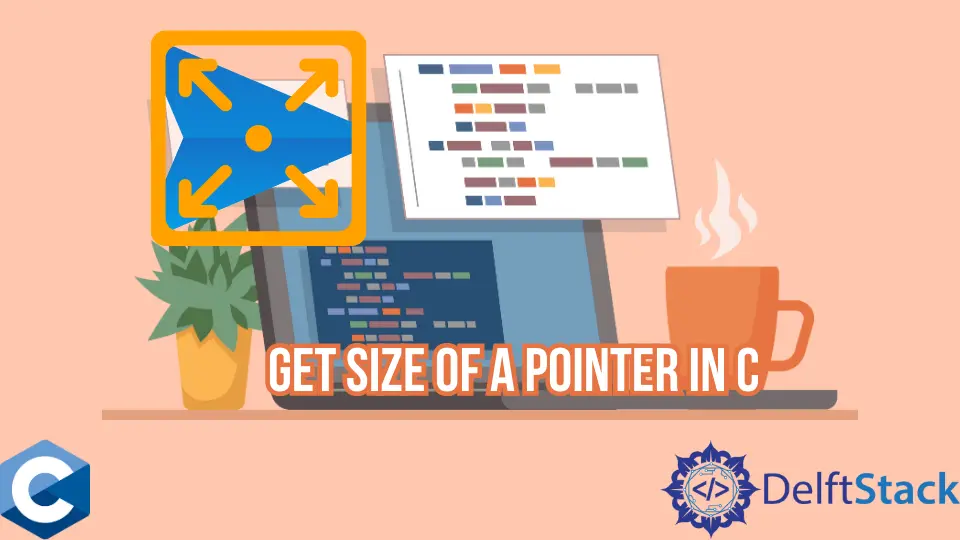
이 기사에서는 C 프로그래밍 언어를 사용하여 포인터의 크기를 결정하는 방법을 보여줍니다.
C 프로그래밍 언어의 포인터
포인터로 알려진 변수는 값이 다른 변수의 주소인 변수입니다. 즉, 포인터 자체에 메모리 위치의 직접 주소가 포함됩니다. 포인터를 사용하여 변수의 위치를 유지하려면 먼저 포인터를 선언해야 합니다.
통사론:
type *var - name;
여기서 type
은 포인터의 기본 유형
을 나타내며 유효한 데이터 유형이어야 하며 var-name
은 포인터를 저장하는 변수의 이름
입니다. 포인터를 지정하는 데 사용되는 별표(*
)는 곱셈에 사용되는 별표와 동일하다는 점에 유의해야 합니다.
한편 이 문장에서 별표(*
)는 특정 변수가 포인터임을 나타내기 위해 사용됩니다. 다음은 포인터에 허용되는 몇 가지 다른 데이터 유형 선언입니다.
int *integerPointer;
char *characterPointer;
float *floatPointer;
double *doublePointer;
sizeof()
메서드를 사용하여 C에서 포인터 크기 가져오기
sizeof()
메소드는 하나의 매개변수만 허용합니다. 컴파일러에 의해 값이 결정되는 연산자입니다. 이러한 이유로 컴파일 타임 단항 연산자라고 합니다.
sizeof()
함수는 부호 없는 정수 데이터 유형을 반환합니다.
데이터 유형이 다른 다양한 변수의 크기를 결정하기 위해 sizeof()
함수를 사용하는 예를 들어 보겠습니다. 각각 int
, char
, float
및 double
데이터 유형을 사용하여 a
, b
, c
및 d
라는 이름의 4개 변수에 대한 초기 값을 설정합니다.
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
*integerPointer
, *characterPointer
, *floatPointer
및 *doublePointer
라는 이름으로 초기화 한 후 각각 a
, b
, c
, d
값을 할당합니다.
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
이제 변수와 포인터 변수의 크기를 출력하려면 printf
명령이 필요합니다.
이러한 명령문은 sizeof
연산자를 활용하는 데 필요하며 여기에 제공되어야 하는 매개변수는 a
, b
, c
, d
및 그에 따라 *integerPointer
, *characterPointer
, *floatPointer
, *doublePointer
입니다.
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
sizeof
메서드의 결과가 부호 없는 정수이기 때문에 %lu
표기가 사용됩니다.
완전한 소스 코드:
#include <stdio.h>
int main() {
int a = 10;
char b = 'x';
float c = 10.01;
double d = 10.01;
int *integerPointer = &a;
char *characterPointer = &b;
float *floatPointer = &c;
double *doublePointer = &d;
printf("size of (a) = %lu bytes", sizeof(a));
printf("\nsize of (a) pointer = %lu bytes", sizeof(*integerPointer));
printf("\nsize of (b) = %lu bytes", sizeof(b));
printf("\nsize of (b) pointer = %lu bytes", sizeof(*characterPointer));
printf("\nsize of (c) = %lu bytes", sizeof(c));
printf("\nsize of (c) pointer = %lu bytes", sizeof(*floatPointer));
printf("\nsize of (d) = %lu bytes", sizeof(d));
printf("\nsize of (d) pointer = %lu bytes", sizeof(*doublePointer));
return 0;
}
출력:
size of (a): = 4 bytes
size of (a) pointer: = 4 bytes
size of (b): = 1 bytes
size of (b) pointer: = 1 bytes
size of (c): = 4 bytes
size of (c) pointer: = 4 bytes
size of (d): = 8 bytes
size of (d) pointer: = 8 bytes
malloc()
메서드를 사용하여 C에서 포인터 크기 가져오기
malloc
기술을 사용하여 크기를 할당하는 포인터 변수의 또 다른 예를 살펴보겠습니다.
main()
함수 내에서 데이터 유형 char
로 포인터 변수를 시작하고 이름을 *cPointer
로 지정합니다. malloc()
이라는 이름의 함수를 할당하고 sizeof()
메소드를 인수로 제공하십시오.
sizeof
메서드는 char *
를 인수로 받아들입니다.
int main() { char *cPointer = malloc(sizeof(char *)); }
이 시점에서 *cPointer
값을 바이트 단위로 출력해야 합니다.
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
완전한 소스 코드:
#include <stdio.h>
#include <stdlib.h>
int main() {
char *cPointer = malloc(sizeof(char *));
printf("Size of the pointer is %lu bytes.\n", sizeof(*cPointer));
return 0;
}
출력:
The size of the pointer is 1 byte.
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn